Page load timeouts play a significant role in web development and user experience, acting as a safeguard against slow-loading pages and contributing to the overall success of a website or web application. Developers work diligently to manage and optimize load times to keep users engaged and satisfied. This article focuses on discussing how to set page load timeouts in Selenium.
What are Selenium Timeouts?
Timeouts in Selenium are the longest periods a WebDriver will wait for specific circumstances or events to happen during a web page interaction. In Selenium, there are many kinds of timeouts:
1. Implicit Wait
Implicit wait is used in software testing or web automation. It is a mechanism used to manage the interaction time among the web elements.
- It controls and instructs the WebDriver to wait for a certain amount of time, before they throw any exception, on not finding the requested element on time.
- While working with Selenium in web automation too, it instructs a WebDriver to wait and poll the DOM until the webelement is found or implicit wait time is elapsed, whichever happens first.
- It tells the WebDriver to wait for a specified amount of time before attempting to locate an element or to throw any exception.
- The WebDriver will go on to the next stage if the element is located before the implicit wait time runs out.
- A TimeoutException is triggered if the element cannot be located in the allotted amount of time.
Example:
Python3
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.common.desired_capabilities import DesiredCapabilities
import time
driver = webdriver.Chrome()
driver.implicitly_wait( 10 )
driver.maximize_window()
ele = driver.find_element(By. ID , "twotabsearchtextbox" )
time.sleep( 4 )
ele.send_keys( "iphone" )
time.sleep( 4 )
driver.quit()
|
Output:
.gif)
Output for implicit wait in Selenium
Explanation:
In the code given above, the driver will wait up to 10 seconds by regularly polling the DOM to find the element. If the element is found within the 10 seconds, it will be written back, or, if the element is not found past 10 seconds, TimeoutException will occur.
2. Explicit Wait
Explicit wait refers to a context used for handling time related synchronization issues, which mostly occurs in a system having different program execution speeds.
- Due to this, it leads to pause or a deliberate delay in the execution of a particular program, until its certain conditions get satisfied and fulfilled before proceeding further.
- By explicitly waiting for a condition to be met, developers can avoid race conditions and ensure that the program progresses only when it is in a valid state.
- In web automation with Selenium too, it is a condition which is defined on the WebDriver to wait for a certain condition to occur within the elapsed time before proceeding to the next stage in the script or code.
- This means, that two conditions are checked in one wait condition, an elapsed time and at the same time, the desired condition to occur.
Example:
Python3
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
import time
driver = webdriver.Chrome()
driver.maximize_window()
wait = WebDriverWait(driver, 10 )
element = wait.until(EC.presence_of_element_located((By. ID , "twotabsearchtextbox" )))
time.sleep( 3 )
element.send_keys( "bestsellers books fiction" )
time.sleep( 3 )
driver.quit()
|
Output:
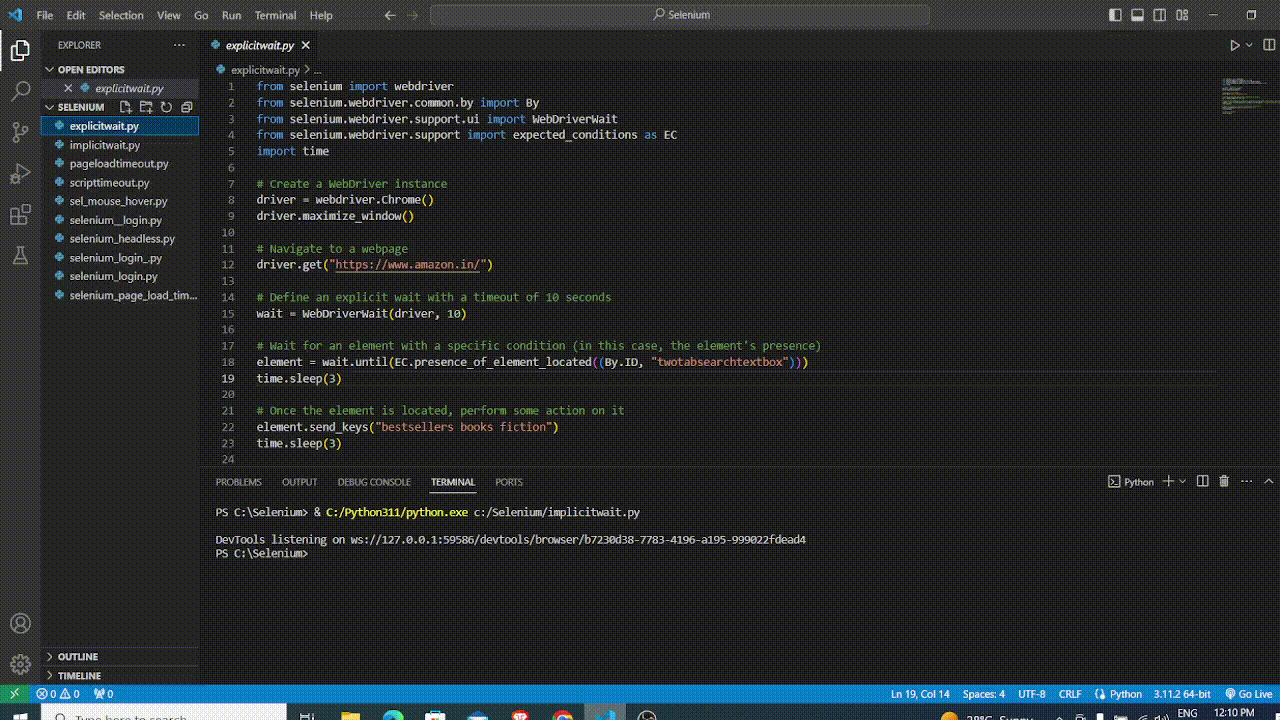
Output for explicit wait in Selenium
Explanation:
- In the code given above, if you want to access any link before moving forward within the stipulated time (here, 10 seconds), you will be waiting for 10 seconds while the element (or link) is available on the webpage.
- At the same time, checking for the ExpectedCondition for the presence of the element (or link) located.
- These ExpectedConditions can be anything, they can be ‘elementToBeClickable’, ‘TitleContains’, ‘ElementIsVisible’, ‘presence_of_element_located’, etc.
- To provide the element and condition to wait for, you may use the WebDriverWait class in conjunction with the expected_conditions module.
- The WebDriver keeps running the script if the condition is satisfied within the allotted timeout, otherwise, TimeoutException gets triggered.
- Even though it depends upon the situation, explicit wait makes more sense because it gives you the control.
- So, you can choose both wait time as well as ExpectedCondition, especially in AJAX based applications where the elements are already present on the webpage but, not accessible or not clickable or not enabled.
3. Page Load Timeout
Page load timeout suggests a webpage or an application to wait for a maximum amount of time to load itself and its pages successfully, before coming to a conclusion whether the webpage or the application is working or not. When a user or a system initiates a request for a webpage or an application then, there is an expectation that the specified action will complete itself within a limited timeframe. If this exceeds then, the system gets compelled to take some predefined actions, such as signaling an error or throwing a timeout message as an exception. While working with Selenium in web automation too, the Selenium WebDriver would take a maximum time to wait for a webpage to load itself completely. One should be very cautious while setting an appropriate timeout for a page, as this can vary among pages in terms of complexity and loading times due to factors such as slow internet connection, heavy JavaScript, or any asynchronous requests (e.g., AJAX). It is set using the set_page_load_timeout method on the WebDriver instance. If the page doesn’t load within the specified time, a TimeoutException is raised. This is specifically used for handling page load events.
Example:
Python3
from selenium import webdriver
driver = webdriver.Chrome()
driver.set_page_load_timeout( 2 )
try :
print ( "Page title:" , driver.title)
except Exception as e:
print ( "Page load failed:" , str (e))
finally :
driver.quit()
|
As shown above, when the page exceeds its page load time (here, 2 seconds), a TimeoutException is raised.
Output:

Output for page load timeout
4. Script Timeout
Script timeout, generally, refers to the maximum amount of time during which a script or a program is allowed to execute itself, before being considered as time consuming. This mechanism also helps in handling the scripts or programs, preventing them to run indefinitely, which further could result in performance issues or unresponsiveness in a system. In the context of web automation with Selenium too, it is the maximum time limit during which a script (especially, a JavaScript code) is allowed to execute or run itself within a web browser. With the help of this, the WebDriver easily allows for the execution of JavaScript in a page. It is set using the set_script_timeout method on the WebDriver instance. If a piece of JavaScript code running in the page takes longer than the specified time to execute, a TimeoutException is raised.
Example:
Python3
from selenium import webdriver
driver = webdriver.Chrome()
driver.set_script_timeout( 10 )
try :
driver.execute_script( "for(let i=0; i<1000000; i++);" )
print ( "Script executed successfully" )
except Exception as e:
print ( "Script execution failed:" , str (e))
finally :
driver.quit()
|
As shown above, since, the script executes itself within the maximum time limit, successful execution of the script is shown as its output.
Output:

Output for script timeout
Why page load timeout matters?
As talked about page load timeout, it is an important asset which every webpage on a browser requires and needs to have. There are various reasons for this, which are briefly discussed below:
- Page Completeness: Page load timeout in Selenium is necessary for a page completeness. This is because while handling any page, the Selenium interacts with the webelements on the webpage to perform any action till its end. So, without waiting for the page to load fully, it’s possible to encounter elements that are not yet available, leading to NoSuchElementExceptions or other errors.
- Consistency: Page load timeout is also very important to handle or maintain the consistency in the webpages. This is because there are various causes which may lead to malfunctioning of the page, making it challenging to stay reliable and stable among different use cases and test cases.
- User Experience Simulation: Setting a page load timeout also helps in simulating a better user experience. This is because users typically wait for a web page to load fully before interacting with it, and so, your automation should also mimic the same.
What is Timeout Exception?
Now, coming to the timeout exception, it is a type of exception that arises when a program or script runs out of its allotted time to complete a particular task or action. Also, many programming languages and frameworks use this to handle those operations that comparatively take longer time to execute themselves, so that they do not hang for long.
In the context of web automation and Selenium, a timeout exception commonly refers to the TimeoutException class provided by Selenium. This exception is raised when an operation related to web elements or page loading takes longer than a predefined timeout period.
There are certain reasons for the cause of timeout exceptions, some of which are described below:
- Network delays: When a user makes an HTTP request to a remote server for the data transmission, a network delay may occur which can further cause timeout exception.
- Resource unavailability: While accessing any webpage or an application, if any resource such as any file, database or hardware device goes unavailable, a timeout exception occurs.
- Complex or time consuming computations: This condition arises when an algorithm or task takes longer time than expected, which leads to code timeout exceptions.
- Web Automation: This occurs while waiting for an element to become available, clickable or visible on a webpage, when it goes timeout and leads to timeout exception.
How page load timeout works in Selenium?
As we know, in Selenium, the page load timeout is a setting that determines the maximum amount of time the WebDriver should wait for a web page to load completely before raising a TimeoutException. And, this timeout is important as it ensures that the test cases work properly and do not hangs anytime. To understand this, let’s look at its working and how set_page_load_timeout works with it.
1. Setting the Page Load Timeout
To set the page load timeout, one can make use of set_page_load_timeout method of the WebDriver instance. The role of this method is to take a single argument as time (in seconds), in order to allow the page to load.
2. WebDriver’s Behavior
While navigating through the webpage with the help of “get” method, the WebDriver keeps a check on the timer of the page load timeout which has been set. If the page doesn’t load within the specified time, the WebDriver will raise a TimeoutException. This allows to handle the timeout gracefully in the test scripts.
3. Handling Timeout Exceptions
When a page load timeout occurs, TimeoutExceptions can easily be caught. Common strategies include logging the timeout, retrying the navigation, or moving on to the next test step. The approach to be chosen may depend on the specific requirements of the test suite and the behavior of the testing application.
4. Impact on Subsequent Commands
It’s important to note that the page load timeout setting applies only to page navigation commands like get. It does not affect the implicit or explicit waits set using implicitly_wait or WebDriverWait. These waits are used to wait for elements on the page to become available, while the page load timeout is specifically for waiting for the entire page to load.
How to handle timeout exception in Selenium?
As read, if any webelement on a webpage or a webpage goes timeout or takes longer time to load, it leads to timeout exceptions. And, these exceptions need to be handled as soon as possible to avoid any further chaos. It’s an important part of writing robust and reliable test scripts. So, to handle these exceptions, one can follow certain steps and strategies to apply it in their codes and scripts. They are discussed below:
1. Implicit Wait
Handling of timeout exceptions can be done with the help of implicit wait. Implicit wait is a mechanism in which it instructs the WebDriver to wait for a certain amount of time for a page or a webelement to load itself, before throwing NoSuchElementException. Exception handling is done using driver.manage().timeouts().implicitlyWait() in which the WebDriver waits for a certain amount of time for which it is instructed. If it finds the elements or gets the result within the specified time, it proceeds with the next action, otherwise, throws a NoSuchElementException.
2. Explicit Wait
An explicit wait can also take part in handling timeout exceptions. It is a mechanism which allows to wait for a specific condition to be met before proceeding with the next action. Unlike implicit waits, explicit waits are not global and are applied only to specific elements or conditions. Explicit waits are commonly used to handle exceptions in Selenium because they give finer control over waiting for elements to appear, disappear, or meet other conditions. This helps make tests more reliable and efficient.
3. Timeouts with PageLoad Strategy
Setting timeouts with a PageLoad Strategy in Selenium may also result in handling timeout exceptions. It is a mechanism to control the behavior of the WebDriver for how long it should wait for a page to load before proceeding with other actions. One can use timeouts with a PageLoad Strategy to enhance the reliability of the test scripts and reduce the likelihood of certain exceptions. This can be done using pageLoadTimeout to ensure that the page has fully loaded before attempting to interact with its elements. If the page fails to load within the specified time, a TimeoutException can be caught and handled as an exception.
4. Handling StaleElementReferenceException
In Selenium, handling StaleElementReferenceException is an important part for writing robust and reliable test scripts. This exception is suppose to occur when any previously located webelement on the webpage becomes “stale” or is no longer attached with the DOM (Document Object Model). Any modification in the webpage such as page refresh, page update or any other changes leads to such exceptions. After this, if anyone tries to interact with the webelements to perform any actions, such as clicking, sending keys, etc., it will throw an exception.
How to set page load timeout in selenium?
In Selenium with Python, you can set a page load timeout using the ‘timeouts’ feature provided by the WebDriver. Below are the steps that can be taken to perform the given task.
1. Install Selenium
Firstly, to start with, you need to install Selenium in your machine. To do so, you can use command prompt to run the following command.
pip install selenium
periods
2. Import the necessary modules
Next, install all the required modules in your system.
from selenium import webdriver
from selenium.common.exceptions import TimeoutException
import time
3. Create an instance for WebDriver
In the next step, create an instance for the WebDriver which you are going to use. Here, Chrome is chosen.
driver = webdriver.Chrome()
driver.maximize_window()
4. Set the page load timeout
Now, use the ‘set_page_load_timeout’ method to set the maximum time the WebDriver should wait for a page to load completely. In the example, it’s set to 3 seconds (but can be changed).
page_load_timeout = 3
driver.set_page_load_timeout(page_load_timeout)
5. Navigate to a webpage
With the help of try block, navigate to the URL of the webpage.
driver.get("https://www.google.com/")
6. Automate the code
Now, wrap your navigation and automation code within a try-except block to catch the TimeoutException. If the page doesn’t load within the specified timeout, a TimeoutException will be raised.
print(f"Page load exceeded {page_load_timeout} seconds.")
7. Close the WebDriver
Finally, close the WebDriver.
driver.quit()
Here’s an Python example to take you through the complete understanding for the above steps:
Python
from selenium import webdriver
from selenium.common.exceptions import TimeoutException
import time
driver = webdriver.Chrome()
driver.maximize_window()
page_load_timeout = 3
driver.set_page_load_timeout(page_load_timeout)
try :
except TimeoutException:
print (f "Page load exceeded {page_load_timeout} seconds." )
else :
print (driver.title)
finally :
driver.quit()
|
In the above given example, ‘set_page_load_timeout’ method is first used to set the maximum time for the WebDriver for which it should wait to let the page complete its loading. Then, after, automation is performed with the try-except block to catch the ‘TimeoutException’. If the page doesn’t load within the specific timeout, a ‘TimeoutException’ will be raised. Handling of the page load timeout, as needed, is done in the except block. Along with these, an else block is also added to show if no exception occurs in the try block, and prints the title of the webpage. Ultimately, finally block is used to ensure to close the WebDriver instance to release the resources.
Output:
With exception:

output with exception
As shown above, when the page load exceeds its timeout, output appears as “Page load exceeded 2 seconds”
Without exception:

output without exception
As shown above, when the page load doesn’t exceeds its timeout, then no exception occurs and the output appears as the title of the webpage.
Conclusion
To sum up, establishing and setting up a page load timeout for a webpage or a webelement on a webpage is an essential component of web automation as it keeps a check on and guarantees the test scripts or the codes to not hang for soo long at anytime during the execution. Also, by defining a maximum timeout for any action, it leads to execution of a page within that maximum allotted time, or else, Selenium would throw a timeout exception. So, for test automations to be robust and dependable, one needs to adapt the techniques to handle such exceptions and which allows to gracefully manage scenarios in which a page loads more slowly than anticipated, keeping the scripts from becoming stuck and failing.
Share your thoughts in the comments
Please Login to comment...