How to Set Offset an HTML Anchor Element to Adjust Fixed Header in CSS ?
Last Updated :
18 Jul, 2023
When designing a website with a fixed header, we might face an issue where clicking on a link obscures the target element due to the header. This affects the user experience negatively as the content gets hidden. In this article, we will see how to offset HTML anchors for a fixed header, ensuring proper visibility for users. HTML anchors, also called anchor links or hash links, create internal links on a webpage. Clicking on them scrolls to the target element, but a fixed header can hide it.
Syntax:
<a href="#section-id">Text</a>
Here, the “#section-id” refers to the section’s identifier that the anchor link should navigate to. For example, if the section has the ID “about”, the anchor link would be <a href=”#about”>About</a>.
Approaches: Using CSS
One approach is to adjust the scroll position of the anchor link using CSS. By making the position:fixed to the fixed header’s height, we can ensure the target section is not obscured. The given HTML code displays a webpage that has a header that remains fixed in position and includes offsetting anchor links that adjust the scrolling position. The <header> tag represents the fixed header, which includes a navigation menu designed with CSS. Each navigation link corresponds to a specific section on the page, identified by its unique id. The CSS properties control the header and navigation menu’s position and appearance. By using CSS, the target element is adjusted to accommodate the fixed header. When anchor links are clicked, the browser smoothly scrolls to the relevant sections. This code showcases a fixed header with offset anchor links for better navigation.
Example 1: This example illustrates the basic techniques that can be used to offset an HTML anchor to accommodate a fixed header.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
offsetting an html anchor to
adjust for fixed header
</ title >
< style >
body {
padding: 0px;
margin: 0px;
}
/* Add styles to adjust for the fixed header */
#target-element {
display: block;
position: relative;
top: -100px;
/* Replace with the height of your fixed header */
visibility: hidden;
}
/* Style the navigation menu */
header {
position: fixed;
top: 0;
width: 100%;
height: 100px;
background-color: green;
z-index: 100;
}
nav {
display: flex;
justify-content: space-between;
align-items: center;
height: 100%;
padding: 0 20px;
}
nav ul {
list-style: none;
margin: 0;
padding: 0;
display: flex;
}
nav ul li {
margin-right: 20px;
}
nav ul li:last-child {
margin-right: 0;
}
nav ul li a {
color: #fff;
text-decoration: none;
font-size: 16px;
font-weight: bold;
transition: color 0.3s ease;
}
nav ul li a:hover {
color: red;
}
</ style >
</ head >
< body >
< header >
< nav >
< ul >
< li >
< a href = "#home" >Home</ a >
</ li >
< li >
< a href = "#about" >About</ a >
</ li >
< li >
< a href = "#services" >Services</ a >
</ li >
< li >
< a href = "#contact" >Contact</ a >
</ li >
</ ul >
</ nav >
</ header >
< section id = "home"
style="height: 200px;
margin-top: 10rem;">
< h1 >Welcome to Our Website</ h1 >
</ section >
< section id = "about"
style = "height: 300px;" >
< h2 >About Us</ h2 >
</ section >
< section id = "services"
style = "height: 400px;" >
< h2 >Our Services</ h2 >
</ section >
< section id = "contact"
style = "height: 600px;" >
< h2 >Contact Us</ h2 >
</ section >
< a href = "#target-element" >
Go to Target Element
</ a >
</ body >
</ html >
|
Output:
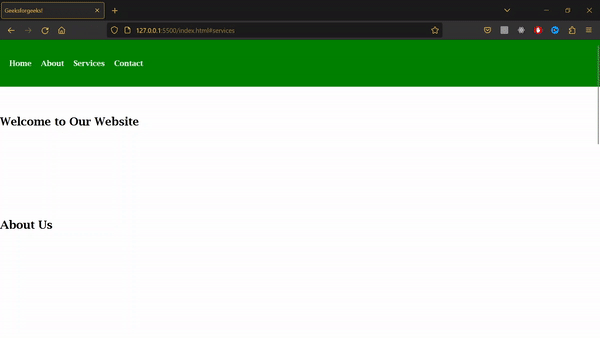
Approach 2: Using Javascript
This approach is to utilize JavaScript to adjust the scroll position after clicking on an anchor link. By offsetting the scroll position by the fixed header’s height, you can ensure the target element is visible below the header.
Here, JavaScript is utilized to enable smooth scrolling when anchor links starting with # are clicked. The code selects these anchor links using a document.querySelectorAll(‘a[href^=”#”]’) and adds event listeners to prevent the default behavior. When an anchor link is clicked, the script uses document.querySelector to find the target element. The window.scrollTo() method is then called, smoothly scrolling to the target element’s offsetTop position using the ‘smooth’ property.
Example 2: This is another approach that will be utilized to adjust an HTML anchor to account for a fixed header.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
offsetting an html anchor to
adjust for fixed header
</ title >
< style >
body {
margin: 0px;
padding: 0px;
}
/* Add styles to adjust for the fixed header */
#target-element {
display: block;
position: relative;
top: -100px;
/* Replace with the height of your fixed header */
visibility: hidden;
}
/* Style the navigation menu */
header {
position: fixed;
top: 0;
width: 100%;
height: 100px;
background-color: green;
z-index: 100;
}
nav {
display: flex;
justify-content: space-between;
align-items: center;
height: 100%;
padding: 0 20px;
}
nav ul {
list-style: none;
margin: 0;
padding: 0;
display: flex;
}
nav ul li {
margin-right: 20px;
}
nav ul li:last-child {
margin-right: 0;
}
nav ul li a {
color: #fff;
text-decoration: none;
font-size: 16px;
font-weight: bold;
transition: color 0.3s ease;
}
nav ul li a:hover {
color: red;
}
</ style >
</ head >
< body >
< header >
< nav >
< ul >
< li >
< a href = "#home" >Home</ a >
</ li >
< li >
< a href = "#about" >About</ a >
</ li >
< li >
< a href = "#services" >Services</ a >
</ li >
< li >
< a href = "#contact" >Contact</ a >
</ li >
</ ul >
</ nav >
</ header >
< section id = "home"
style="height: 200px;
margin-top: 10rem;">
< h1 >Welcome to Our Website</ h1 >
</ section >
< section id = "about"
style = "height: 400px;" >
< h2 >About Us</ h2 >
</ section >
< section id = "services"
style = "height: 400px;" >
< h2 >Our Services</ h2 >
</ section >
< section id = "contact"
style = "height: 400px;" >
< h2 >Contact Us</ h2 >
</ section >
< script >
// Smooth scrolling functionality using JavaScript
document.querySelectorAll('a[href^="#"]').forEach(anchor => {
anchor.addEventListener('click', function (e) {
e.preventDefault();
const targetElement =
document.querySelector(this.getAttribute('href'));
window.scrollTo({
top: targetElement.offsetTop,
behavior: 'smooth'
});
});
});
</ script >
</ body >
</ html >
|
Output:
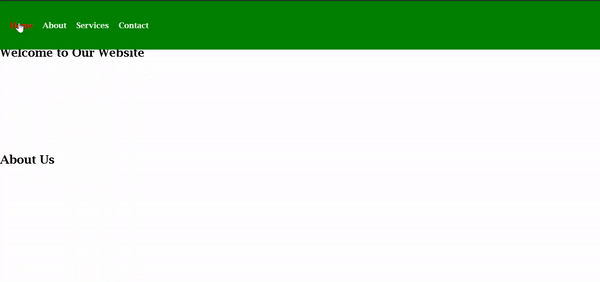
In conclusion, To ensure the visibility of anchor links on pages with fixed headers, offsetting an HTML anchor is a technique that adjusts the scroll position by the height of the header. This can be done using JavaScript or CSS, improving the user experience.
Share your thoughts in the comments
Please Login to comment...