How to Send an Image using Ajax ?
Last Updated :
25 Oct, 2022
Ajax stands for Asynchronous Javascript and XML and is used to make indirect requests to other origins. It can help you to perform a change to the web page dynamically.
We can make an AJAX request with a special object called XMLHttpRequest which provides us with different methods to create an HTTP requests.
Syntax:
xhr = new XMLHttpRequest();
xhr.onload = ()=>{...};
xhr.open(<method>,<url>);
xhr.send();
Methods Used:
- open: This accepts 3 parameters:
- method: the HTTP method
- url: the resource path
- async / sync
- send: Sends the final request
- onload: To listen for the response
In this article, we will learn to upload an image using AJAX.
Approach:
To send an image we need to make a post request to the server and upload the image firstly by converting it into DATAURL format.
Steps:
- Use a file input button to select an image
- Add an oninput event listener which gets the uploaded file with e.target.files property
- Using FileReader.readAsDataURL method converts the acquired file into dataurl.
- Getting the input creates a new instance of XMLHttpRequest
- Prepare the POST request and send the data in string format
- On the server side, we are using express to listen for POST requests at localhost:3000
Example 1: Below is the implementation of the above steps:
- Client: This is the client-side code that uploads the image to the server.
HTML
< html >
< body >
< input type = "file" />
< script type = "text/javascript" >
let result;
let input = document.getElementsByTagName('input')[0];
input.oninput=(e)=>{
console.log(e);
let file = e.target.files[0];
const reader = new FileReader();
reader.onload = (evt) => {
console.log(evt.target.result);
result = evt.target.result;
const xhr = new XMLHttpRequest();
xhr.open('POST', url);
xhr.send(result);
};
reader.readAsDataURL(file);
}
</ script >
</ body >
</ html >
|
- Server: Accepting the Image with EXPRESS post listener
Javascript
'use strict' ;
const express = require( 'express' );
run(). catch (err => console.log(err));
async function run() {
const app = express();
app.post( '/events' , (req, res) => {
res.set({
'Cache-Control' : 'no-cache' ,
'Access-Control-Allow-Origin' : '*'
});
req.on( "data" , (data) => {
console.log(data.toString());
})
res.send();
})
}
|
Output:
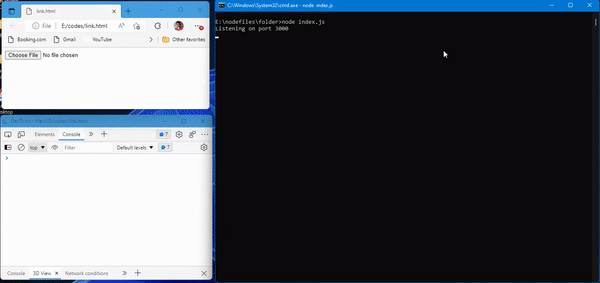
OUTPUT
In the above GIF image, we find that the image is getting transferred in DATAURL format. The tabs on the left represent the client and the large black tab on the right represents the server.
We can select the image with the file input button and immediately the transfer takes place.
Example 2: In this example, we will send an image and display the image which is reverted back from the server in the img tag.
- Client: The client displays the image in the img tag after receiving back the image with xhr.onload as shown below
HTML
< html >
< body >
< input type = "file" />
< img src = "" />
< script type = "text/javascript" >
let result;
let input = document.getElementsByTagName('input')[0];
input.oninput = (e) => {
let file = e.target.files[0];
const reader = new FileReader();
reader.onload = (evt) => {
result = evt.target.result;
const xhr = new XMLHttpRequest();
xhr.onload = (res) => {
document.getElementsByTagName('img')[0].src = res.target.response;
}
xhr.open('POST', url);
xhr.send(result);
};
reader.readAsDataURL(file);
}
</ script >
</ body >
</ html >
|
- Server: The server is sending the data back using the status and send method after listening with the on “data” listener.
Javascript
var express = require( 'express' );
var app = express();
app.post( '/events' , (req, res) => {
res.set({
'Cache-Control' : 'no-cache' ,
'Access-Control-Allow-Origin' : '*'
});
req.on( "data" , (data) => {
res.status(200);
res.send(data.toString());
})
})
app.listen(3000);
|
Output:
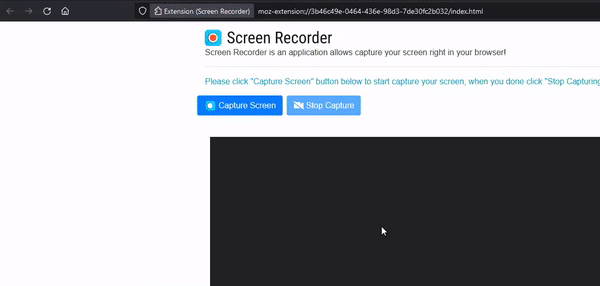
OUTPUT
In the above GIF image, we find that the image is getting transferred in DATAURL format. The image is reverted back from the server and it is displayed on the client.
Share your thoughts in the comments
Please Login to comment...