How to Select an Element by ID in JavaScript ?
Last Updated :
05 Feb, 2024
In JavaScript, we can use the “id” attribute to interact with HTML elements. The “id” attribute in HTML assigns a unique identifier to an element. This uniqueness makes it easy for JavaScript to precisely target and manipulate specific elements on a webpage. Selecting elements by ID helps in dynamic content updates, event handling, and DOM manipulation.
Using getElementById method
JavaScript hase getElementById
method to access and retrieve a reference to an HTML element by its unique identifier. This method enables dynamic interaction with the Document Object Model (DOM) by allowing direct manipulation of specific elements on a web page.
Syntax:
let element = document.getElementById("myElement");
Example: To demonstrate selecting an HTML element by ID in JavaScript and then using it for updating the state of another HTML element.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" />
< meta name = "viewport" content=" width = device -width,
initial-scale = 1 .0" />
< title >Selecting by ID in JavaScript</ title >
</ head >
< body >
< div style = "margin: 80px" >
< h1 id = "myElement" >Hello, World!</ h1 >
< button onclick = "changeColor()" >Change Color</ button >
</ div >
< script >
function changeColor() {
// Selecting element by ID
let element = document.getElementById("myElement");
// Manipulating the selected element
element.style.color = "red";
}
</ script >
</ body >
</ html >
|
Output:
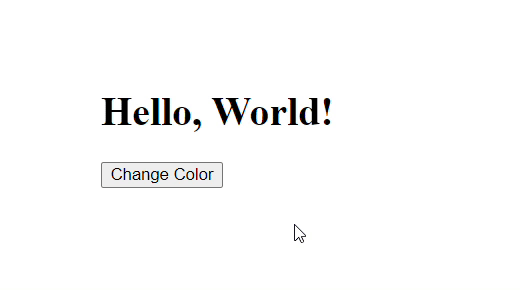
Using the query selector method
The querySelector method is a more flexible and versatile approach to element selection. In this case, the CSS selector #myElement targets the element with the specified ID. While querySelector is powerful, it’s essential to note that if multiple elements share the same ID, it will only return the first one encountered in the document.
Syntax:
let element = document.querySelector("#myElement");
Example: This HTML example creates a webpage with a heading and a button. Clicking the button triggers a JavaScript function that changes the text color of the heading to blue.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" />
< meta name = "viewport" content=" width = device -width,
initial-scale = 1 .0" />
< title >Selecting by ID in JavaScript</ title >
</ head >
< body >
< div style = "margin: 80px" >
< h1 id = "myElement" >Hello, World!</ h1 >
< button onclick = "changeColor()" >Change Color</ button >
</ div >
< script >
function changeColor() {
// Selecting element by ID
let element = document.querySelector("#myElement");
// Manipulating the selected element
element.style.color = "blue";
}
</ script >
</ body >
</ html >
|
Output:
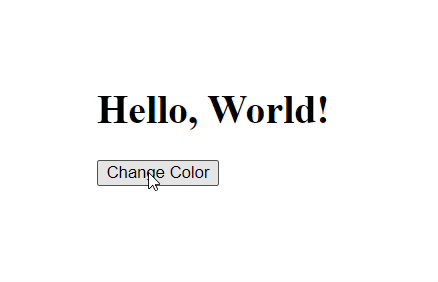
Share your thoughts in the comments
Please Login to comment...