How to Schedule a Task in Python?
Last Updated :
15 Mar, 2024
We will see what is task scheduling in Python and how to schedule a task in Python with examples. In this article, we will see how we can schedule a task in Python.
What is Task Scheduling in Python?
Task scheduling involves automating processes by defining tasks to be executed at predetermined times or intervals. In Python, task scheduling simplifies repetitive operations by allowing developers to schedule tasks to run autonomously, enhancing productivity and efficiency.
Key Concepts:
- Task Scheduling: Task scheduling entails automating processes by defining tasks to be executed at predetermined times or intervals.
- schedule Library: Python’s
schedule
library simplifies task scheduling with a straightforward and intuitive syntax. - Time-Based Scheduling: Tasks can be scheduled to run at precise times using the
at()
method. - Interval-Based Scheduling: The
every()
method enables tasks to be scheduled at regular intervals. - Task Functions: Task functions are Python functions executed when scheduled tasks are triggered.
How to Schedule a Task in Python?
Below, are the examples of how to schedule a task In Python With Examples.
- Schedule Task at Specific Time
- Schedule Task at Specific Intervals
- Python Automated Email Scheduler
To schedule tasks in Python using the schedule
library, First install the schedule
library, use the following command:
pip install schedule
After installing the library, import the necessary modules (schedule
and time
) in your Python script using the following commands:
import schedule
import time
Schedule Task at Specific Time
In this example, in below Python code the `schedule` library to schedule a daily task of printing a specific message at 10:35 AM. The code enters an infinite loop, checking for pending tasks and executing them while avoiding high CPU usage by incorporating a 1-second sleep interval.
Python3
import schedule
import time
# Define a function to print a message
def print_message():
print("Hello! It's time to code on GFG")
# Schedule the task to run every day at 7:00 AM
schedule.every().day.at("10:35").do(print_message)
while True:
schedule.run_pending()
time.sleep(1)
Output
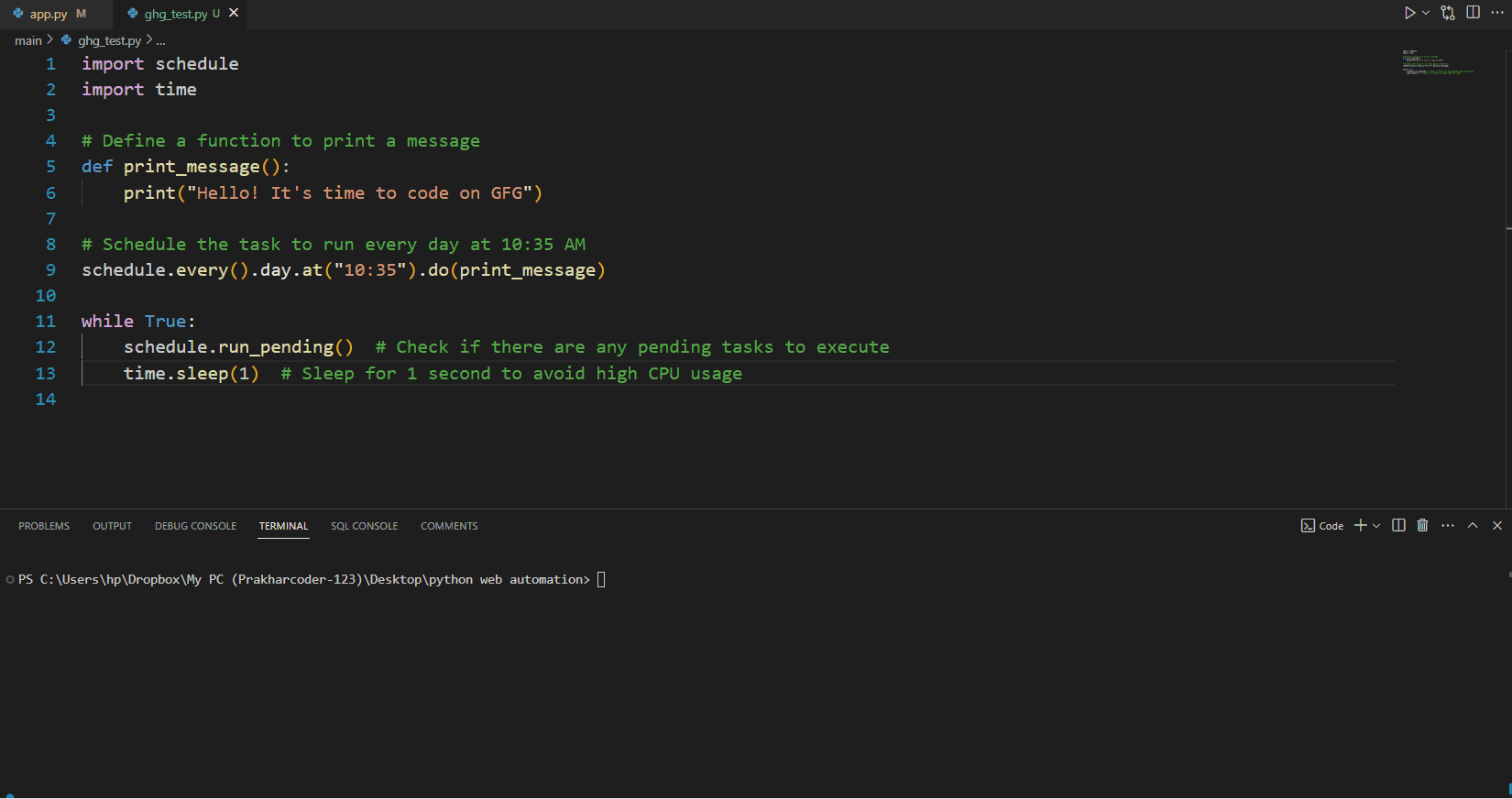
Schedule Task at Intervals
In this example, in below Python code, the `schedule` library is employed to execute a task every 5 seconds. The task, defined by the `print_message()` function, prints the current time. below code runs indefinitely, checking and executing pending tasks while incorporating a 1-second sleep interval for efficiency.
Python3
import schedule
import time
def print_message():
print("Task executed at:", time.strftime("%H:%M:%S"))
# Schedule task to run every 5 seconds
schedule.every(5).seconds.do(print_message)
# Keep the program running to allow scheduled tasks to execute
while True:
schedule.run_pending()
time.sleep(1)
Output
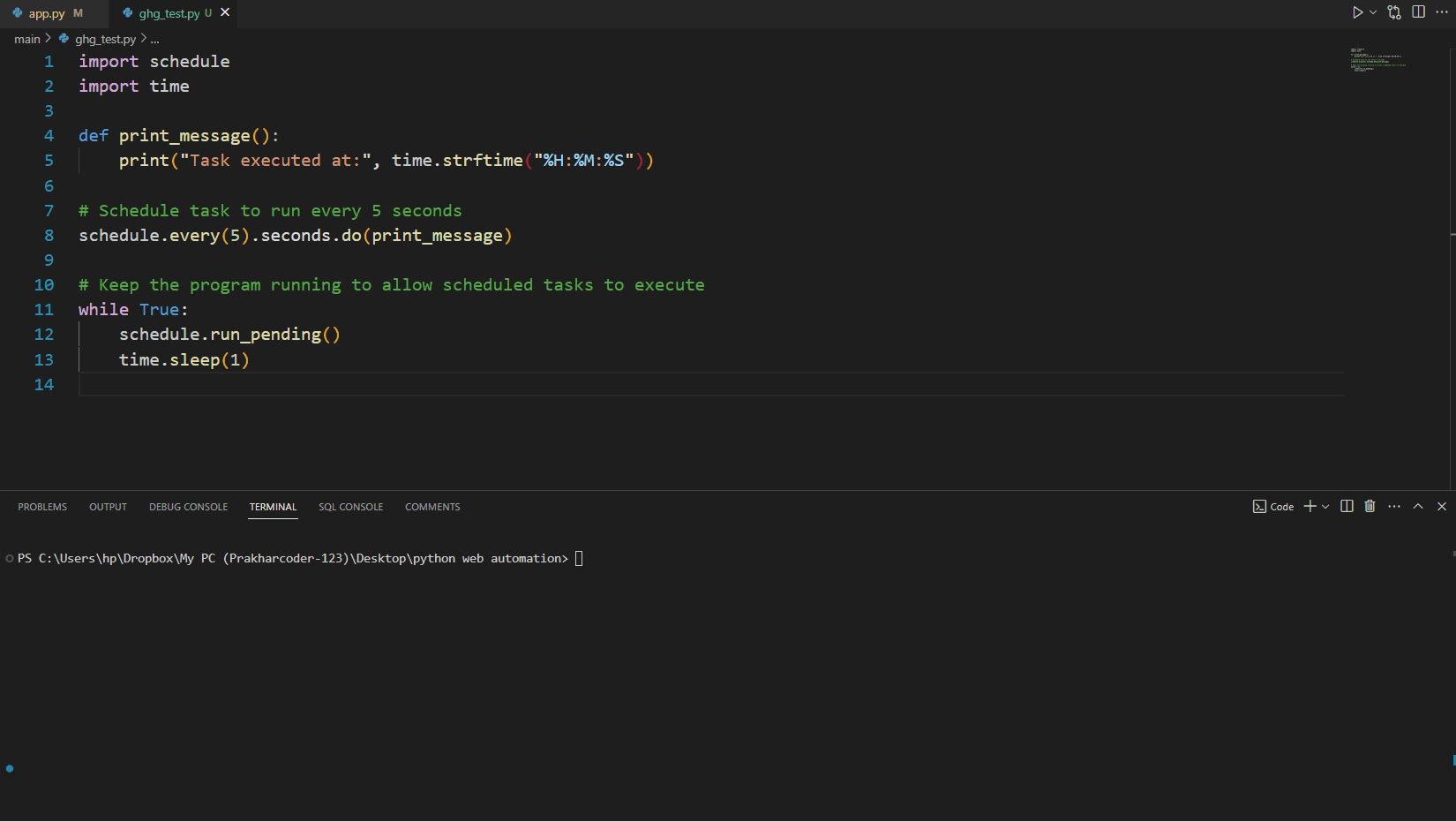
Python Automated Email Scheduler
In this example, In this Python code, the `schedule` library is utilized to schedule a task. The function `send_email()` is defined to simulate sending an email, and the task is scheduled to run every day at 11:53 PM using `schedule.every().day.at(“23:53”).do(send_email)`. below code runs continuously, checking and executing pending tasks while ensuring efficiency with a 1-second sleep interval.
Python3
import schedule
import time
# Define a function to send email
def send_email():
print("Email sent at:", time.strftime("%H:%M:%S"))
# Schedule the task to run every day at 11:53 pm
schedule.every().day.at("23:53").do(send_email)
# Keep the program running to allow scheduled tasks to execute
while True:
schedule.run_pending()
time.sleep(1)
Output
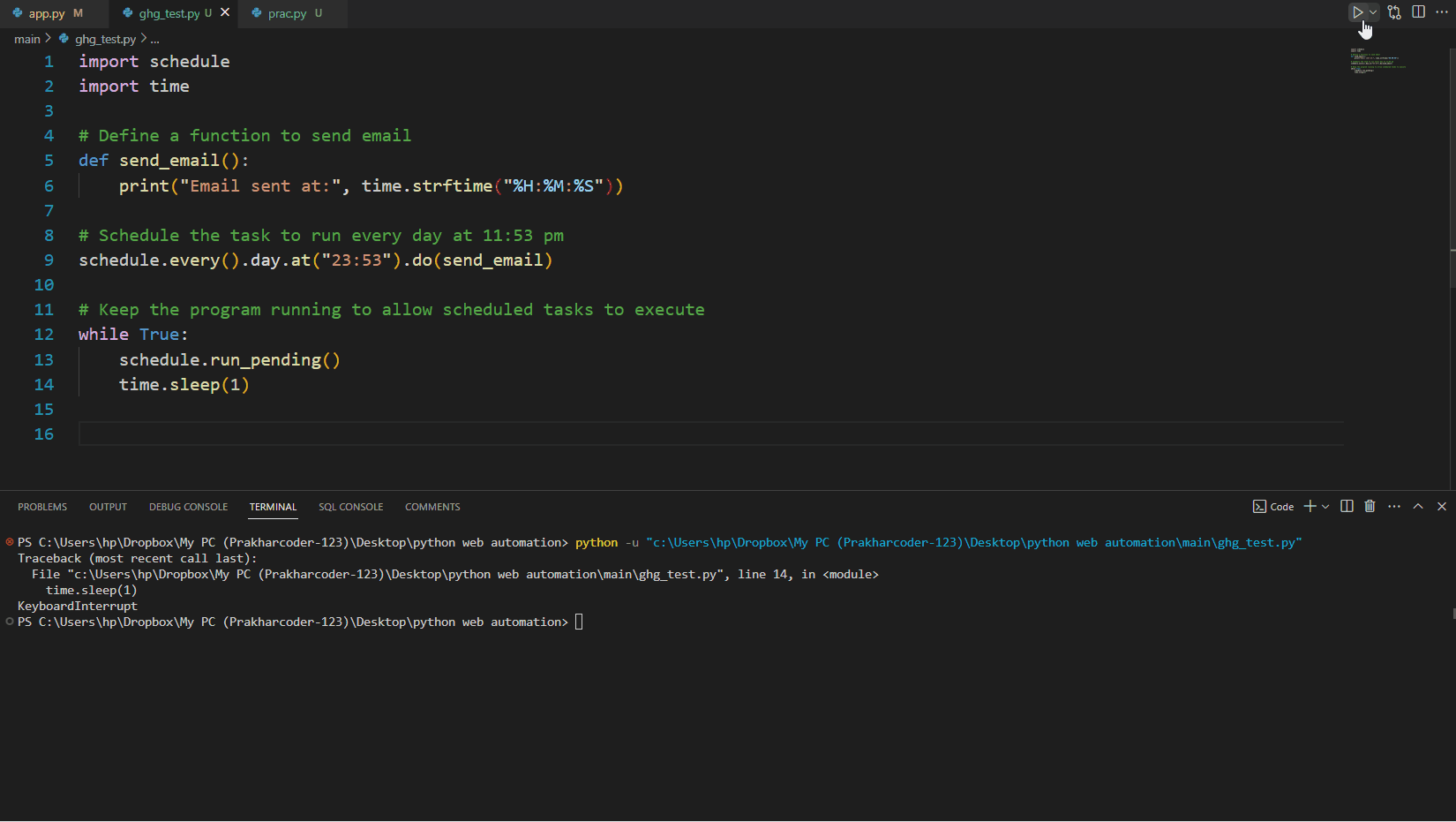
email_scheduling
Share your thoughts in the comments
Please Login to comment...