How to Return an Array that Contains an Array and Hash in Ruby Method?
Last Updated :
02 Apr, 2024
In this article, we will learn how to return an array that contains an array and hash. We can return an array that contains an array and hash using the methods mentioned below:
Using an Explicit Return Statement
The return
the keyword is used to explicitly return the array, which contains both the array and the hash.
Syntax:
return [inner_array, inner_hash]
Example:
In this example, we create an array and a hash and then use return
keyword to explicitly return an array containing both inner array and inner hash. The
result of calling the method is stored in a variable result
, and it’s printed.
Ruby
# Method to return an array containing an array and a hash
def return_array
# Define an array
inner_array = [1, 2, 3]
# Define a hash
inner_hash = { name: "John", age: 30 }
# Return an array containing the array and hash
return [inner_array, inner_hash]
end
# Call the method and store the result
result = return_array
# Print the result
p result
Output:
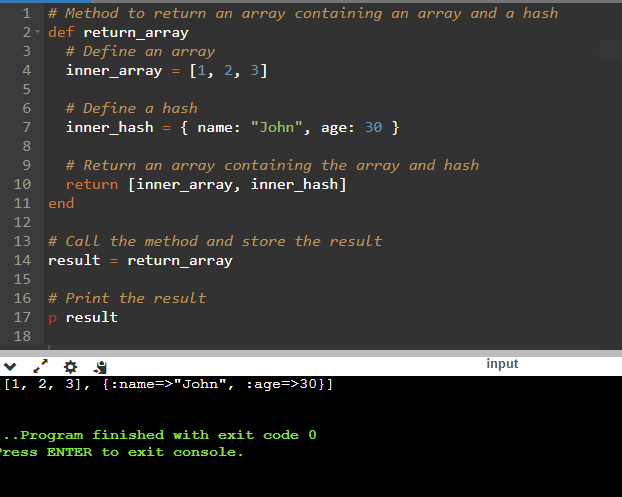
Using Implicit Return Statement
Return statemnet is not used here instead we directly define and implicitly returns the array, allowing the last expression to be the return value.
Syntax:
[inner_array, inner_hash]
Example:
In this example we create an array and a hash and then the method implicitly returns an array containing both inner array and inner hash. The result is stored in a variable result
and printed
Ruby
# Method to return an array containing an array and a hash
def return_array
# Define an array
inner_array = [1, 2, 3]
# Define a hash
inner_hash = { name: "John", age: 30 }
# Implicit return of an array containing the array and hash
[inner_array, inner_hash]
end
# Call the method and store the result
result = return_array
# Print the result
p result
Output:
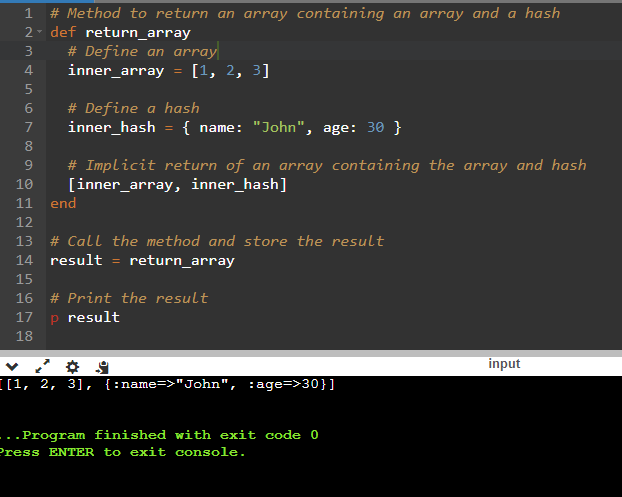
Using Array Literal and Hash Constructor
the array and hash are directly constructed within the return statemen to return an array containing an inner array and a hash using literals.
Syntax:
[[array_value1, array_value2], {key1: “value 1”, key 2: value 2 }];
Example:
In this example both the array and hash are constructed using literals directly within the array.The result is stored in a variable result
and printed.
Ruby
# Method to return an array containing an array and a hash
def return_array
# Return an array containing an array and hash using literals
[[1, 2, 3], { name: "John", age: 30 }]
end
# Call the method and store the result
result = return_array
# Print the result
p result
Output:
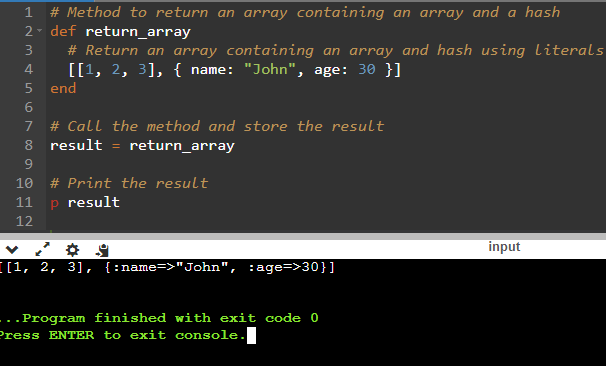
Share your thoughts in the comments
Please Login to comment...