How to resolve req.body is empty in posts error in Express?
Last Updated :
26 Dec, 2023
In Express the req.body is empty error poses a critical challenge in web development, particularly in the context of processing POST requests on the server side. This issue arises when the server encounters difficulties parsing the request body, resulting in an empty or undefined req.body object. Developers looking for dependable data handling mechanisms may become frustrated by this obstacle, which can impede the smooth flow of data between clients and servers.
Causes of req.body is empty Error:
- Missing middleware configuration for parsing the body during post request.
- An incorrect header is used when calling a post request from the client.
How to Resolve req.body is empty error:
Approach 1: Middleware Configuration
Ensure you have implemented the necessary middlewares for parsing the data for post requirements . In express, express.json and express.urlencoded are built-in functions for parsing post request.
Approach 2: Correct Header for Calling Post request from client side.
When calling the APIs using fetch make sure the Content-Header has application/json or application/x-www-form-urlencoded in the payload. We will now see how to implement with the example below
Steps to create Express Application
Step 1: Create a folder using the command and initialize the node project using the command
mkdir foldername
cd foldername
npm init -y
Step 2: Create the necessary files
touch index.js
Step 3: Installing the necessary modules
npm i express cors
Example 1: We will create a simple express server that will take data using post request and we will show how to use the express.json() function and express.urlencoded() function and will use postman to call the api
Javascript
const express = require( 'express' );
const app = express();
const port = 3000;
app.use(express.urlencoded({ extended: true }));
app.use(express.json());
app.post( '/api/data' , (req, res) => {
const data = req.body;
res.status(200).json({ data: data, message: 'Data received successfully' });
});
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
|
Steps to run the project
Step 1: Enter the following command to run the project
node index.js
Step 2: Open postman and open a new request and call the api using post and send the data in json and urlencoded format
Output:
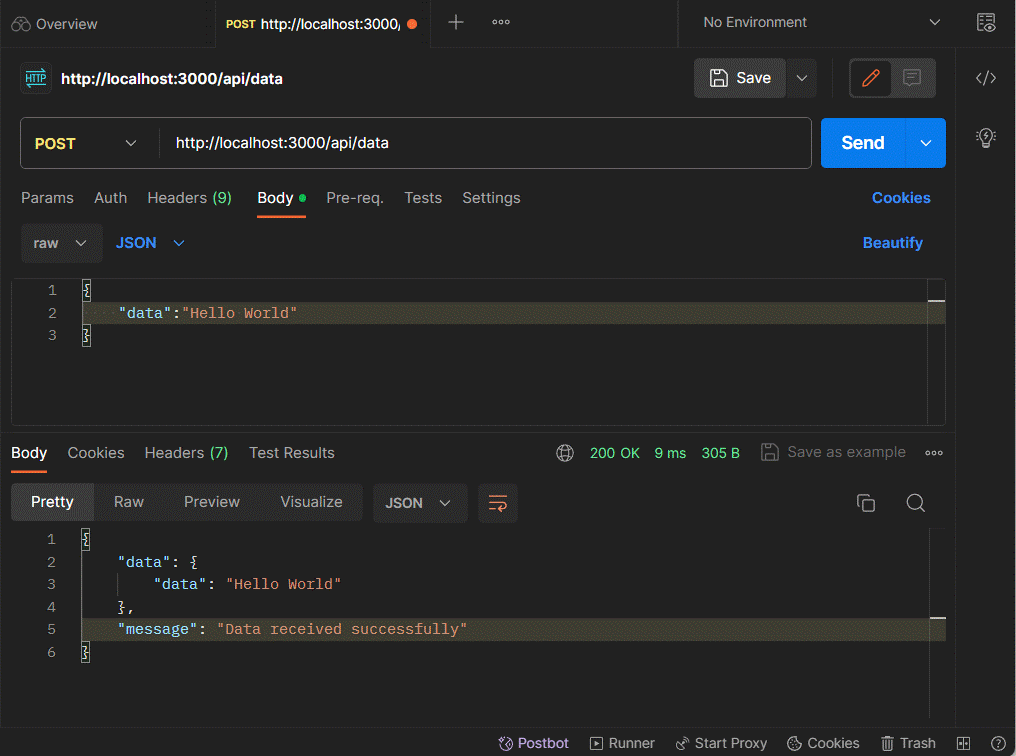
Example 2: We will create client (html page) to call our api data using fetch function and will show how to use content/application-json to call the post request
Javascript
const express = require( 'express' );
const app = express();
const port = 3000;
const cors = require( "cors" );
app.use(express.urlencoded({ extended: true }));
app.use(express.json());
app.use(cors( "*" ))
app.post( '/api/data' , (req, res) => {
const data = req.body;
res.status(200).json(
{ data: data, message: 'Data received successfully' });
});
app.post( '/api/content' , (req, res) => {
const {name,email,age} = req.body;
data = {name:name,email:email,age:age};
res.status(200).json(
{ data: data, message: 'Data received successfully' });
});
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
|
HTML
<!DOCTYPE html>
< html >
< head >
< title >API Request</ title >
< style >
body {
font-family: Arial, sans-serif;
background-color: #f2f2f2;
}
h1 {
text-align: center;
color: #333;
}
div {
max-width: 400px;
margin: 0 auto;
padding: 20px;
background-color: #fff;
border-radius: 5px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
label {
display: block;
margin-bottom: 10px;
color: #333;
}
input[type="text"],
input[type="email"],
input[type="number"] {
width: 100%;
padding: 10px;
border: 1px solid #ccc;
border-radius: 4px;
box-sizing: border-box;
margin-bottom: 20px;
}
button {
background-color: #4CAF50;
color: white;
padding: 10px 20px;
border: none;
border-radius: 4px;
cursor: pointer;
}
#result {
margin-top: 20px;
padding: 10px;
background-color: #f9f9f9;
border: 1px solid #ccc;
border-radius: 4px;
}
</ style >
</ head >
< body >
< h1 >API Request</ h1 >
< div id = "myForm" >
< label for = "name" >Name:</ label >
< input type = "text" id = "name" name = "name" required>< br >< br >
< label for = "email" >Email:</ label >
< input type = "email" id = "email" name = "email" required>< br >< br >
< label for = "age" >Age:</ label >
< input type = "number" id = "age" name = "age" required>< br >< br >
< button onclick = "getData()" >Submit</ button >
</ div >
< div id = "result" ></ div >
< script >
function getData() {
console.log("getData() called");
const name = document.getElementById("name").value;
const email = document.getElementById("email").value;
const age = document.getElementById("age").value;
const data = { name, email, age };
method: "POST",
headers: {
"Content-Type": "application/json"
},
body: JSON.stringify(data)
})
.then(response => response.json())
.then(result => {
console.log(result);
document.getElementById("result").innerHTML
= JSON.stringify(result);
// Display the API response in the "result" div
})
.catch(error => {
console.error(error);
// Handle any errors here
});
};
</ script >
</ body >
</ html >
|
Steps to run the project
Step 1: Enter the following command to run the project
node index.js
Step 2: Open html page in the browser and enter the data and click on button then it will display the output
Output:
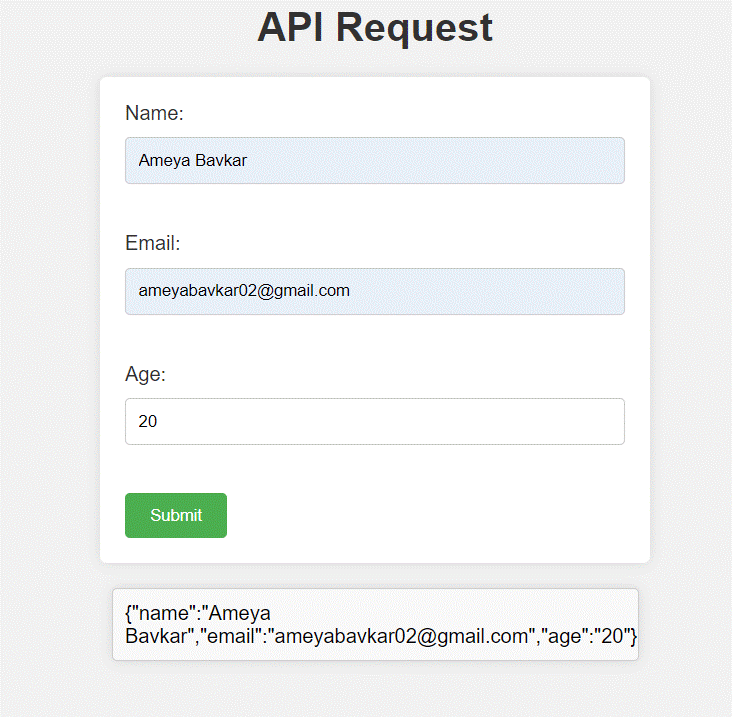
Share your thoughts in the comments
Please Login to comment...