How to pass JavaScript variables to PHP ?
Last Updated :
26 Dec, 2023
JavaScript is the client side and PHP is the server-side script language. The way to pass a JavaScript variable to PHP is through a request.
Below are the methods to pass JavaScript variables to PHP:
Method 1: Using GET/POST method
This example uses the form element and GET/POST method to pass JavaScript variables to PHP. The form of contents can be accessed through the GET and POST actions in PHP. When the form is submitted, the client sends the form data in the form of a URL such as:
https://example.com?name=value
This type of URL is only visible if we use the GET action, the POST action hides the information in the URL.
Client Side:
html
<!DOCTYPE html>
< html >
< head >
< title >
Passing JavaScript variables to PHP
</ title >
</ head >
< body >
< h1 style = "color:green;" >
GeeksforGeeks
</ h1 >
< form method = "get" name = "form" action = "destination.php" >
< input type = "text" placeholder = "Enter Data" name = "data" >
< input type = "submit" value = "Submit" >
</ form >
</ body >
</ html >
|
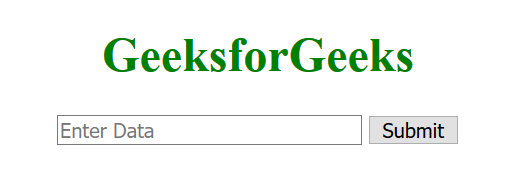
Server Side(PHP):
On the server side PHP page, we request for the data submitted by the form and display the result.
php
<?php
$result = $_GET [ 'data' ];
echo $result ;
?>
|
Output:

Client Side: Use Cookie to store the information, which is then requested in the PHP page. A cookie named gfg is created in the code below and the value GeeksforGeeks is stored. While creating a cookie, an expire time should also be specified, which is 10 days for this case.
Javascript
$(document).ready( function () {
createCookie( "gfg" , "GeeksforGeeks" , "10" );
});
function createCookie(name, value, days) {
let expires;
if (days) {
let date = new Date();
date.setTime(date.getTime() + (days * 24 * 60 * 60 * 1000));
expires = "; expires=" + date.toGMTString();
}
else {
expires = "" ;
}
document.cookie = escape(name) + "=" +
escape(value) + expires + "; path=/" ;
}
|
Server Side(PHP): On the server side, we request for the cookie by specifying the name gfg and extract the data to display it on the screen.
php
<?php
echo $_COOKIE [ "gfg" ];
?>
|
Output:

JavaScript is best known for web page development but it is also used in a variety of non-browser environments. You can learn JavaScript from the ground up by following this JavaScript Tutorial and JavaScript Examples.
PHP is a server-side scripting language designed specifically for web development. You can learn PHP from the ground up by following this PHP Tutorial and PHP Examples.
Share your thoughts in the comments
Please Login to comment...