How to Pass a Serializable Object from One Activity to Another Activity in Android?
Last Updated :
02 Feb, 2023
While developing an application in Android, the developer can create more than one activity in the application. So, there can be many activities and can be a case to transfer data from one activity to the other. In such cases, Intents are used. Intents let the user jump from one activity to the other, or go from the current activity to the next activity. While going from one activity to the other, we can pass parameters like strings, integers, etc from the current activity and fetch them in the next activity.
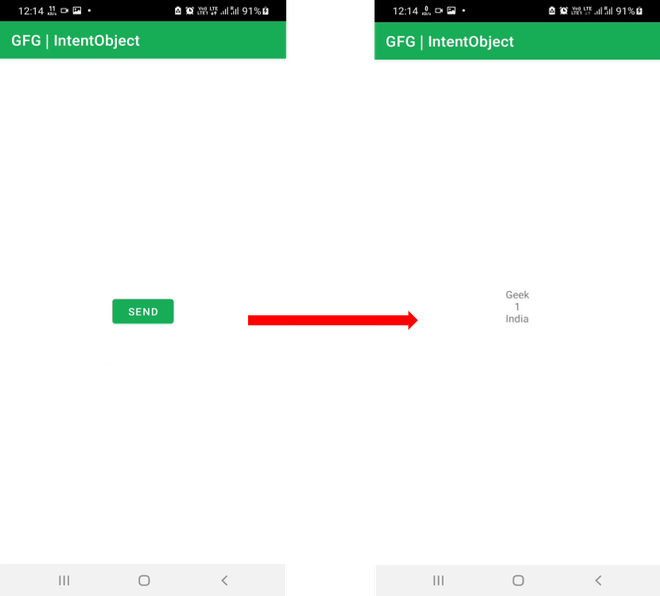
In this article, we will show you how you can create a serializable object, pass it from one activity and fetch it in the next activity.
Step by Step Implementation
Step 1: Create a New Project in Android Studio
To create a new project in Android Studio please refer to How to Create/Start a New Project in Android Studio. We demonstrated the application in Kotlin, so make sure you select Kotlin as the primary language while creating a New Project.
Step 2: Create a serializable object (MyCustomObject.kt)
Kotlin
import java.io.Serializable
class MyCustomObject: Serializable {
var name = ""
var id = 0
var place = ""
constructor(mName: String, mId: Int, mPlace: String){
name = mName
id = mId
place = mPlace
}
constructor()
}
|
Java
import java.io.Serializable;
class MyCustomObject implements Serializable {
private String name = "" ;
private int id = 0 ;
private String place = "" ;
public MyCustomObject(String mName, int mId, String mPlace){
name = mName;
id = mId;
place = mPlace;
}
public MyCustomObject() {}
}
|
Step 3: Add a button in the layout of the first activity (activity_main.xml)
XML
<? xml version = "1.0" encoding = "utf-8" ?>
< RelativeLayout
android:layout_width = "match_parent"
android:layout_height = "match_parent"
tools:context = ".MainActivity" >
< Button
android:id = "@+id/btnSend"
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
android:layout_centerInParent = "true"
android:text = "Send" />
</ RelativeLayout >
|
Step 4: Program to pass the object to a new activity (MainActivity.kt)
Kotlin
import android.content.Intent
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.Button
import android.widget.EditText
import java.io.Serializable
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super .onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val btn = findViewById<Button>(R.id.btnSend)
btn.setOnClickListener {
val intent = Intent( this , SecondActivity:: class .java)
val passingObject = MyCustomObject()
passingObject.name = "Geek"
passingObject.id = 1
passingObject.place = "India"
intent.putExtra( "object" , passingObject)
startActivity(intent)
}
}
}
|
Java
import android.content.Intent;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import java.io.Serializable;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super .onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button btn = (Button) findViewById(R.id.btnSend);
btn.setOnClickListener( new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(MainActivity. this , SecondActivity. class );
MyCustomObject passingObject = new MyCustomObject();
passingObject.name = "Geek" ;
passingObject.id = 1 ;
passingObject.place = "India" ;
intent.putExtra( "object" , (Serializable) passingObject);
startActivity(intent);
}
});
}
}
|
Step 5: Create a new activity SecondActivity.kt to catch the passed object and add a TextView in the layout of the second activity activity_second.xml
Kotlin
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.TextView
class SecondActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super .onCreate(savedInstanceState)
setContentView(R.layout.activity_second)
val myIntent = intent
val derivedObject = myIntent.getSerializableExtra( "object" ) as MyCustomObject
val myTextView = findViewById<TextView>(R.id.tv1)
myTextView.append(derivedObject.name + "\n" )
myTextView.append(derivedObject.id.toString() + "\n" )
myTextView.append(derivedObject.place + "\n" )
}
}
|
XML
<? xml version = "1.0" encoding = "utf-8" ?>
< RelativeLayout
android:layout_width = "match_parent"
android:layout_height = "match_parent" >
< TextView
android:id = "@+id/tv1"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:gravity = "center"
android:layout_centerInParent = "true" />
</ RelativeLayout >
|
Java
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.widget.TextView;
public class SecondActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super .onCreate(savedInstanceState);
setContentView(R.layout.activity_second);
Intent myIntent = getIntent();
MyCustomObject derivedObject = (MyCustomObject) myIntent.getSerializableExtra( "object" );
TextView myTextView = (TextView) findViewById(R.id.tv1);
myTextView.append(derivedObject.getName() + "\n" );
myTextView.append(Integer.toString(derivedObject.getId()) + "\n" );
myTextView.append(derivedObject.getPlace() + "\n" );
}
}
|
Output:
Share your thoughts in the comments
Please Login to comment...