How to Parse Data From JSON into Python?
Last Updated :
05 Jul, 2021
JSON (JavaScript Object Notation) is a lightweight data-interchange format. It is easy for humans to read and write for machines to parse and generate. Basically it is used to represent data in a specified format to access and work with data easily. Here we will learn, how to create and parse data from JSON and work with it.
Before starting the details of parsing data, We should know about ‘json’ module in Python. It provides an API that is similar to pickle for converting in-memory objects in Python to a serialized representation as well as makes it easy to parse JSON data and files. Here are some ways to parse data from JSON using Python below:
- Python JSON to Dictionary: With the help of json.loads() function, we can parse JSON objects to dictionary.
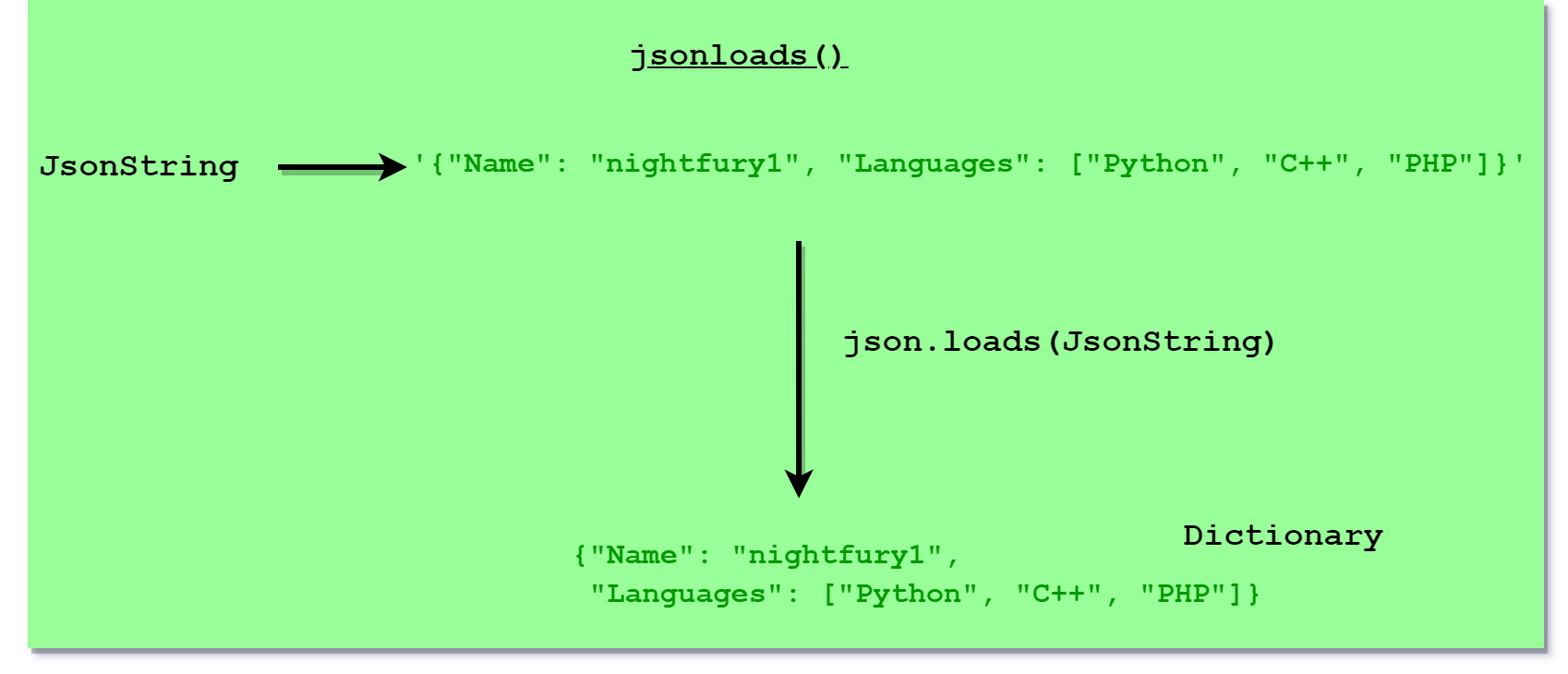
Python3
import json
geek = '{"Name": "nightfury1", "Languages": ["Python", "C++", "PHP"]}'
geek_dict = json.loads(geek)
print ( "Dictionary after parsing: " , geek_dict)
print ( "\nValues in Languages: " , geek_dict[ 'Languages' ])
|
Output:
Dictionary after parsing: {‘Name’: ‘nightfury1’, ‘Languages’: [‘Python’, ‘C++’, ‘PHP’]}
Values in Languages: [‘Python’, ‘C++’, ‘PHP’]
- Python JSON to Ordered Dictionary: We have to use same json.loads() function for parsing the objects, but for getting in ordered, we have to add keyword ‘object_pairs_hook=OrderedDict‘ from collections module.
Python3
import json
from collections import OrderedDict
data = json.loads( '{"GeeksforGeeks":1, "Gulshan": 2, "nightfury_1": 3, "Geek": 4}' ,
object_pairs_hook = OrderedDict)
print ( "Ordered Dictionary: " , data)
|
Output:
Ordered Dictionary: OrderedDict([(‘GeeksforGeeks’, 1), (‘Gulshan’, 2), (‘nightfury_1’, 3), (‘Geek’, 4)])
- Parse using JSON file: With the help of json.load() method, we can parse JSON objects to dictionary format by opening the required JSON file.
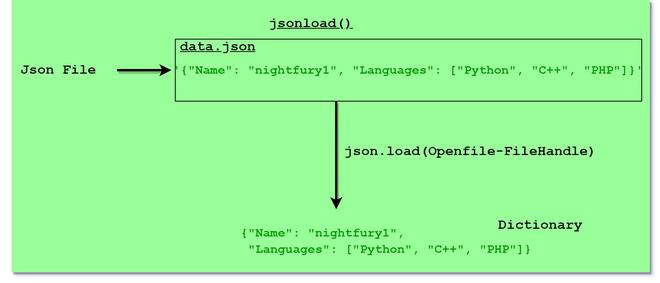
Python3
import json
with open ( 'data.json' ) as f:
data = json.load(f)
print (data)
|
Output:
{‘Name’: ‘nightfury1’, ‘Language’: [‘Python’, ‘C++’, ‘PHP’]}
Share your thoughts in the comments
Please Login to comment...