How to operate callback-based fs.readFile() method with promises in Node.js ?
Last Updated :
18 Jul, 2020
The fs.readFile() method is defined in the File System module of Node.js. The File System module is basically to interact with the hard disk of the user’s computer. The readFile() method is used to asynchronously read the entire contents of a file and returns the buffer form of the data.
The fs.readFile() method is based on callback. Using callback method leads to a great chance of callback nesting or callback hell problems. Thus to avoid it we almost always like to work with a promise-based method. Using some extra node.js methods we can operate a callback-based method in promise way.
Syntax:
fs.readFile(path, options)
Note: Callback not required since we operate the method with promises.
Parameters: This method accept two parameters as mentioned above and described below:
- path: It is a String, Buffer or URL that specifies the path to the file whose contents we try to read.
- options: It is an optional parameter which affects the output in someway accordingly we provide it to the function call or not.
- encoding: It is a string that specifies the encoding technique, default is null.
- flag: It is a string that specifies the file system flags. Its default value is ‘r’.
Approach: The fs.readFile() method based on callback. To operate it with promises, first, we use promisify() method defined in the utilities module to convert it into a promise based method.
Example 1: Filename: index.js
const fs = require( 'fs' )
const util = require( 'util' )
const readFileContent = util.promisify(fs.readFile)
readFileContent( './testFile.txt' )
.then(buff => {
const contents = buff.toString()
console.log(`\nContents of the file :\n${contents}`)
})
. catch (err => {
console.log(`Error occurs, Error code -> ${err.code},
Error No -> ${err.errno}`);
});
|
Implementing the same functionality using async-await.
const fs = require( 'fs' )
const util = require( 'util' )
const readFileContent = util.promisify(fs.readFile)
const fetchFile = async (path) => {
const buff = await readFileContent(path)
const contents = buff.toString()
console.log(`\nContents of the file :\n${contents}`)
}
fetchFile( './testFile.txt' )
. catch (err => {
console.log(`Error Occurs, Error code -> ${err.code},
Error NO -> ${err.errno}`);
});
|
Run the index.js file using the following command:
node index.js
Output:
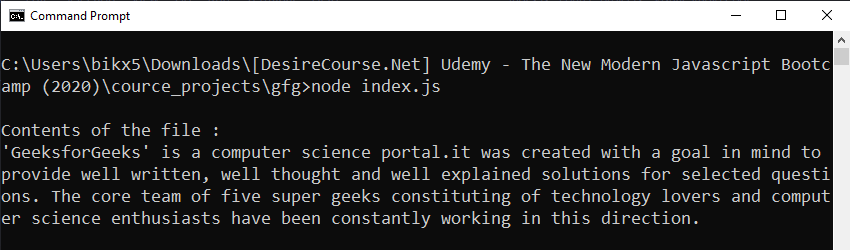
Example 2: If given path to the file does not exist.
Filename: index.js
const fs = require( 'fs' )
const util = require( 'util' )
const readFileContent = util.promisify(fs.readFile)
readFileContent( './false/path.txt' )
.then(buff => {
const contents = buff.toString()
console.log(`\nContents of the file :\n${contents}`)
})
. catch (err => {
console.log(`\nError occurs, Error code -> ${err.code},
Error No -> ${err.errno}`);
})
|
Implementing the same functionality using async-await.
const fs = require( 'fs' )
const util = require( 'util' )
const readFileContent = util.promisify(fs.readFile)
const fetchFile = async (path) => {
const buff = await readFileContent(path)
const contents = buff.toString()
console.log(`\nContents of the file :\n${contents}`)
}
fetchFile( './false/path' )
. catch (err => {
console.log(`\nError Occurs, Error code -> ${err.code},
Error NO -> ${err.errno}`);
});
|
Run the index.js file using the following command:
node index.js
Output:

Explanation: The fs.readFile() method reads the contents of the file and hence it expected a path to the file that exist. Since the given path to the file does not exist, an error occurs with error code ‘ENOENT’ and error number ‘-4058’. The ‘ENOENT’ error occurs when a specified pathname does not exist.
Example 3: When given path is path to a folder not file.
Filename: index.js
const fs = require( 'fs' )
const util = require( 'util' )
const readFileContent = util.promisify(fs.readFile)
readFileContent( './testFolder' )
.then(buff => {
const contents = buff.toString()
console.log(`\nContents of the file :\n${contents}`)
})
. catch (err => {
console.log(`\nError occurs, Error code -> ${err.code},
Error No -> ${err.errno}`);
});
|
Implementing the same functionality using async-await.
const fs = require( 'fs' )
const util = require( 'util' )
const readFileContent = util.promisify(fs.readFile)
const fetchFile = async (path) => {
const buff = await readFileContent(path)
const contents = buff.toString()
console.log(`\nContents of the file :\n${contents}`)
}
fetchFile( './testFolder' )
. catch (err => {
console.log(`\nError Occurs, Error code -> ${err.code},
Error NO -> ${err.errno}`);
});
|
Run the index.js file using the following command:
node index.js
Output:

Explanation: The fs.readFile() method reads the contents of the file and hence it expected path to a file. Since the given path is a path to a folder, an error occurs with error code ‘EISDIR’ and error number ‘-4068’. The ‘EISDIR’ error occurs when an operation expected a file, but the pathname of directory is given.
Share your thoughts in the comments
Please Login to comment...