How to Make an SVG Image in JavaScript ?
Last Updated :
19 Jan, 2024
The SVG images are vector images that do not lose their quality either in the case of Zoom in or Zoom out. These are the Scalable Vector Graphics that can be directly created using the <svg> tag in HTML or dynamically using JavaScript. Let us create an SVG using JavaScript.
We can use the below methods to create SVG images dynamically using JavaScript:
The createElement() method of the HTML DOM can be used to create an SVG and image element dynamically and then the appendChild() method can be used to append it to an element as a child.
Syntax:
const varName = document.createElement(nameOfElementToBeCreated);
Example: The below code example will explain the use of the createElement() method to create an SVG image using JavaScript.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >
How to make an SVG image
using JavaScript?
</ title >
< style >
.container{
text-align: center;
}
</ style >
</ head >
< body >
< div class = "container" >
< h2 style = "color: green;" >
GeeksforGeeks
</ h2 >
< h3 >
The below svg image is created dynamically
using JavaScript.
</ h3 >
</ div >
< script >
const container =
document.querySelector('.container');
const svg =
document.createElementNS
const image =
document.createElementNS
svg.setAttribute('height', '200');
svg.setAttribute('width', '200');
image.setAttribute
('href',
image.setAttribute('height', '200');
image.setAttribute('width', '200');
svg.appendChild(image);
container.appendChild(svg);
</ script >
</ body >
</ html >
|
Output:
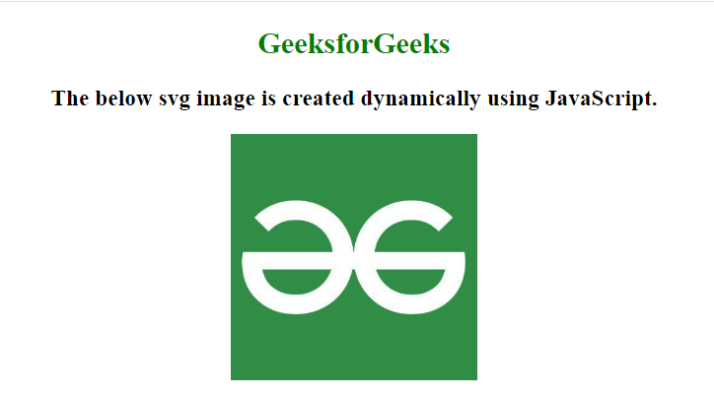
d3.js is a JavaScript library that can be included using the CDN Link inside a HTML document. It can be used to dynamically add a SVG image.
Syntax:
d3.select('selectedHTMLElement').append('svg').append('image');
Example: The below code will explain the use of d3.js to create a SVG image dynamically.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >
How to make an SVG image
using JavaScript?
</ title >
< style >
.container{
text-align: center;
}
</ style >
< script src =
integrity =
"sha512-M7nHCiNUOwFt6Us3r8alutZLm9qMt4s9951uo8jqO4UwJ1hziseL6O3ndFyigx6+LREfZqnhHxYjKRJ8ZQ69DQ=="
crossorigin = "anonymous"
referrerpolicy = "no-referrer" >
</ script >
</ head >
< body >
< div class = "container" >
< h2 style = "color: green;" >
GeeksforGeeks
</ h2 >
< h3 >
The below svg image is created dynamically
using d3.js.
</ h3 >
</ div >
< script >
const createdSvg =
d3.select('.container').
append('svg').
attr('height', '200').
attr('width', '200')
createdSvg.append('image').
attr('height', '200').
attr('width', '200').
attr('href',
</ script >
</ body >
</ html >
|
Output:
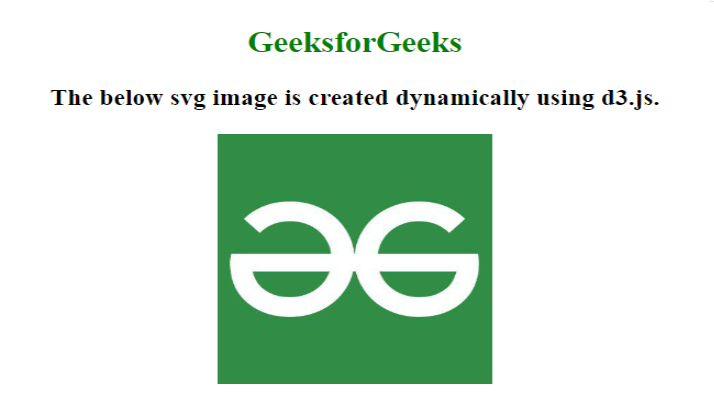
Share your thoughts in the comments
Please Login to comment...