How to Load SVG from URL in Android ImageView?
Last Updated :
08 Jun, 2022
It is seen that many Android apps require to use of high-quality images that will not get blur while zooming. So we have to use high-quality images. But if we are using PNG images then they will get blur after zooming because PNG images are made up of pixels and they will reduce their quality after zooming. So SVG images are preferable to use because SVG images are made up of vectors and they don’t reduce their quality even after zooming. Now we will look at How we can load SVG from its URL in our Android App.
Steps for Loading SVG Image from URL
Step 1: Create a new Android Studio Project
For creating a new Android Studio project just click on File > New > New Project. Make sure to choose your language as JAVA. You can refer to this post on How to Create New Android Studio Project.
Step 2: Before moving to the coding part add these two dependencies in your build.gradle
Go to Gradle Scripts > build.gradle (Module: app) section and add the following dependencies and click the “Sync Now” on the above pop-up. Add these two dependencies.
- implementation ‘com.squareup.okhttp3:okhttp:3.10.0’
- implementation ‘com.pixplicity.sharp:library:1.1.0’
and, Add google repository in the build.gradle file of the application project if by default it is not there
buildscript {
repositories {
google()
mavenCentral()
}
All Jetpack components are available in the Google Maven repository, include them in the build.gradle file
allprojects {
repositories {
google()
mavenCentral()
}
}
Step 3: Now we will move toward the design part
Navigate to the app > res > layout > activity_main.xml. Below is the code for the activity_main.xml file.
Note: Drawables are added in app > res > drawable folder.
Example
XML
<? xml version = "1.0" encoding = "utf-8" ?>
< RelativeLayout
android:layout_width = "match_parent"
android:layout_height = "match_parent"
tools:context = ".MainActivity" >
< ImageView
android:id = "@+id/imageview"
android:layout_width = "match_parent"
android:layout_height = "200dp"
android:layout_centerHorizontal = "true"
android:layout_marginLeft = "10dp"
android:layout_marginTop = "40dp"
android:layout_marginRight = "10dp"
android:contentDescription = "@string/app_name"
android:src = "@drawable/gfgimage" />
</ RelativeLayout >
|
Step 4: Now create a new Java class as Utils
In this JAVA class, we are loading data from the URL in the form of the byte stream. The sharp library will convert this byte stream and will load the SVG image in our target ImageView. To create a new JAVA class navigate to the app > java > your apps package name >> Right-click on it and then click on it and click New > Java class. Give a name to your JAVA class. Below is the code for the Utils.java file. Comments are added inside the code to understand the code in more detail.
Example
Java
import android.content.Context;
import android.widget.ImageView;
import com.pixplicity.sharp.Sharp;
import java.io.IOException;
import java.io.InputStream;
import okhttp3.Cache;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
public class Utils {
private static OkHttpClient httpClient;
public static void fetchSvg(Context context, String url,
final ImageView target)
{
if (httpClient == null ) {
httpClient = new OkHttpClient.Builder()
.cache( new Cache(
context.getCacheDir(),
5 * 1024 * 1014 ))
.build();
}
Request request
= new Request.Builder().url(url).build();
httpClient.newCall(request).enqueue( new Callback() {
@Override
public void onFailure(Call call, IOException e)
{
target.setImageResource(
R.drawable.gfgimage);
}
@Override
public void onResponse(Call call,
Response response)
throws IOException
{
InputStream stream
= response.body().byteStream();
Sharp.loadInputStream(stream).into(target);
stream.close();
}
});
}
}
|
Step 5: Now we will use this Utils class in our MainActivity.java file to load images from the URL
Navigate to the app > java > your apps package name > MainActivity.java file. Below is the code for the MainActivity.java file. Comments are added inside the code to understand the code in more detail.
Java
import android.os.Bundle;
import android.widget.ImageView;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
ImageView imageView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super .onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
imageView = findViewById(R.id.imageview);
String url = " " ;
Utils.fetchSvg( this , url, imageView);
}
}
|
Step 6: Add internet permission in your AndroidManifest file
Navigate to the app > manifest and add internet permission.
XML
<? xml version = "1.0" encoding = "utf-8" ?>
package = "com.gtappdevelopers.frescoimageloading" >
< uses-permission android:name = "android.permission.INTERNET" />
< application
android:allowBackup = "true"
android:icon = "@mipmap/ic_launcher"
android:label = "@string/app_name"
android:roundIcon = "@mipmap/ic_launcher_round"
android:supportsRtl = "true"
android:theme = "@style/Theme.FrescoImageLoading" >
< activity android:name = ".MainActivity" >
< intent-filter >
< action android:name = "android.intent.action.MAIN" />
< category android:name = "android.intent.category.LAUNCHER" />
</ intent-filter >
</ activity >
</ application >
</ manifest >
|
Output:
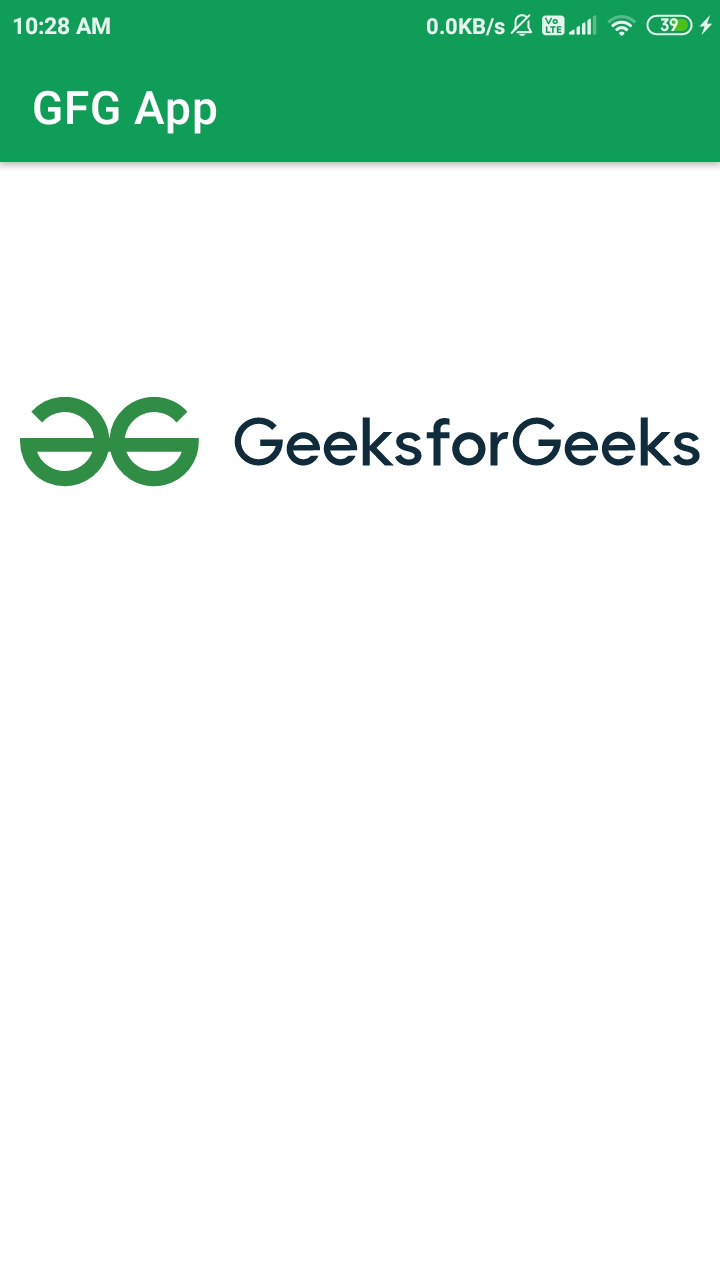
Share your thoughts in the comments
Please Login to comment...