How to implement sleep function in TypeScript?
Last Updated :
07 Nov, 2023
In this article, we will learn How to implement sleep function in TypeScript. Sleep allows the calling thread/program to pause its execution for a specified number of seconds. In TypeScript, you can’t use the traditional synchronous sleep function as you would in some other languages like Python or JavaScript. This is because JavaScript, and by extension TypeScript, is single-threaded, and blocking the main thread would freeze your entire application. However, you can implement a sleep-like function using asynchronous features, such as async/await and Promise.
Syntax:
async function sleep(ms: number): Promise<void> {
return new Promise(
(resolve)
=> setTimeout(resolve, ms));
}
Parameters:
- async: The async keyword indicates that this function will be asynchronous, allowing you to use await inside the function.
- sleep: This is the name of the function, and you can choose a different name if you prefer.
- ms: number: This is the parameter named ms, which represents the number of milliseconds you want to sleep or delay for and it is of type number.
- Promise<void>: The function returns a Promise<void>, indicating that it will asynchronously resolve with no specific value once the sleep period is over.
Example 1: This code defines an async function named sleep, which returns a promise to sleep for the given number of milliseconds. The run function demonstrates how to use the sleep function to introduce delays within an async program.
Javascript
async function sleep(ms: number): Promise<void> {
return new Promise(
(resolve) => setTimeout(resolve, ms));
}
async function run() {
console.log( "Start of the program" );
await sleep(2000);
console.log( "After 2 seconds" );
await sleep(3000);
console.log( "After another 3 seconds" );
}
run();
|
Output:
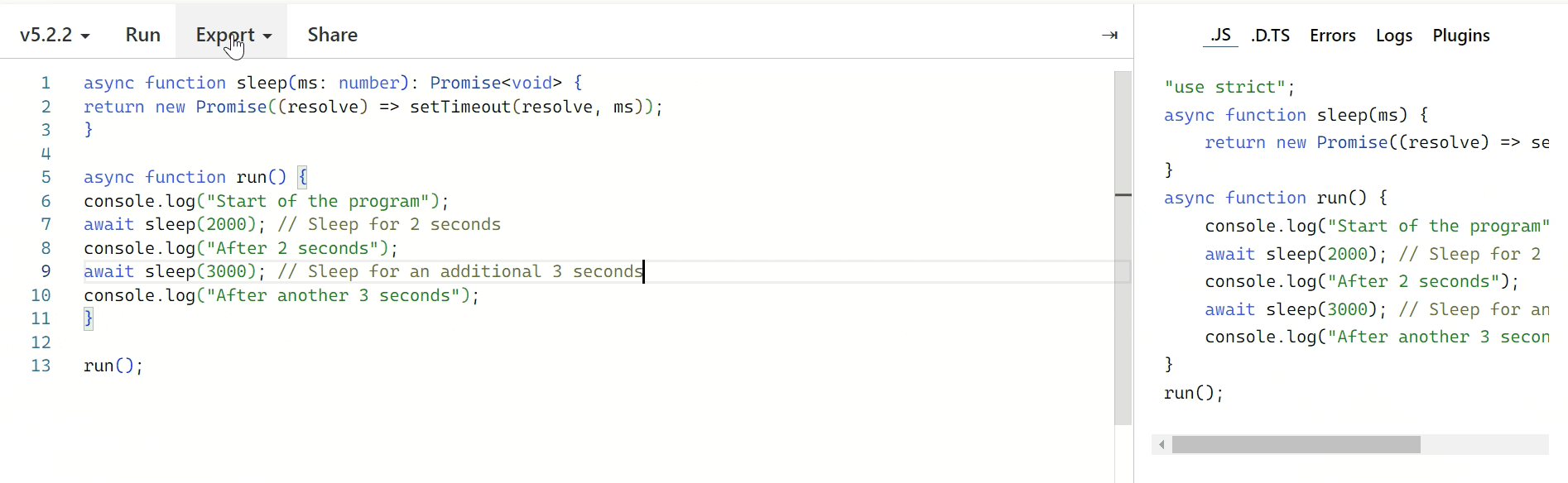
Example 2: This code defines a sleep function that returns a promise to sleep for a given number of milliseconds. The delayAndLog function logs a message, sleeps for a specified duration, and then logs another message when it wakes up.
Javascript
function sleep(ms: number): Promise<void> {
return new Promise(
(resolve) =>
setTimeout(resolve, ms));
}
async function delayAndLog(
message: string, delayMs: number) {
console.log(
`Sleeping for ${delayMs}
milliseconds...`);
await sleep(delayMs);
console.log(
`Woke up after sleeping for
${delayMs} milliseconds. Message: ${message}`);
}
delayAndLog( "GeeksforGeeks!" , 2000);
|
Output:
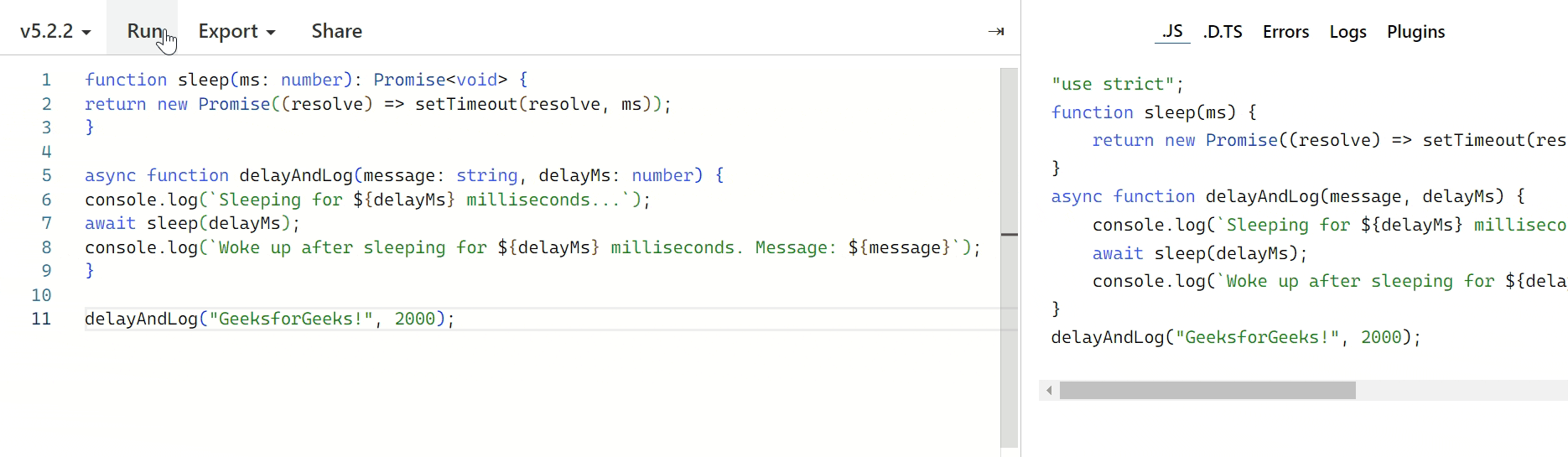
Share your thoughts in the comments
Please Login to comment...