How to Hide the VueJS Syntax While the Page is Loading ?
Last Updated :
10 Jan, 2024
Vue.js is a JavaScript framework used in building powerful and elegant user interfaces. In this article, we will learn how to hide the VueJS syntax while the page is loading. This approach ensures that users don’t see the uncompiled Vue.js syntax during the initial page load, providing a smoother user experience.
The following approaches can be used to accomplish this task:
Steps to Create the Vue App
Step 1: Install Vue modules using the below npm command
npm install vue
Step 2: Use Vue JS through CLI. Open your terminal or command prompt and run the below command.
npm install --global vue-cli
Step 3: Create the new project using the below command
vue init webpack myproject
Step 4: Change the directory to the newly created project
cd myproject
Project Structure
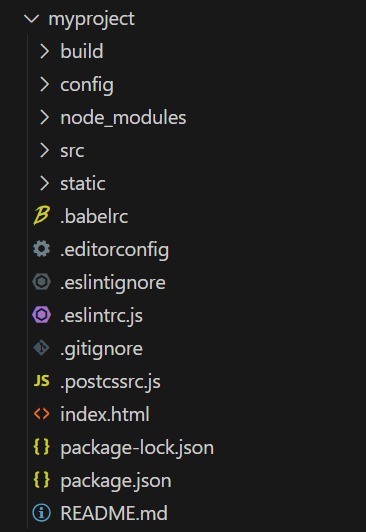
Approach 1: Using the v-cloak directive
In this approach, we will use the v-cloak directive provided by Vue.js to hide the Vue.js syntax until the page has loaded. This directive is designed to prevent the Vue.js template from being visible until Vue.js has compiled and mounted the associated component.
Syntax:
<span v-cloak>{{textMessage}}</span>
Example: The following example demonstrates the usage of the v-cloak directive in Vue.js to hide the Vue.js syntax while the page is loading. Remember to define a stylesheet rule for v-cloak to set the display to none.
HTML
<!DOCTYPE html>
< html >
< head >
< meta charset = "utf-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< style >
body {
height: 90vh;
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
}
h1 {
color: green;
}
[v-cloak] {
display: none;
}
#app {
padding: 10px;
font-size: 1.25rem;
}
</ style >
< title >vue-geeksforgeeks</ title >
</ head >
< body >
< h1 >
GeeksforGeeks v-cloak directive
</ h1 >
< div id = "app" >
< div >
Without v-cloak: {{ message }}
</ div >
< div >With v-cloak:
< span v-cloak>{{ message }}</ span >
</ div >
</ div >
< script src =
</ script >
< script >
setTimeout(() => {
const app = Vue.createApp({
data() {
return {
message:
"This is a sample message"
}
}
})
app.mount('#app')
}, 2000);
</ script >
</ body >
</ html >
|
Output:
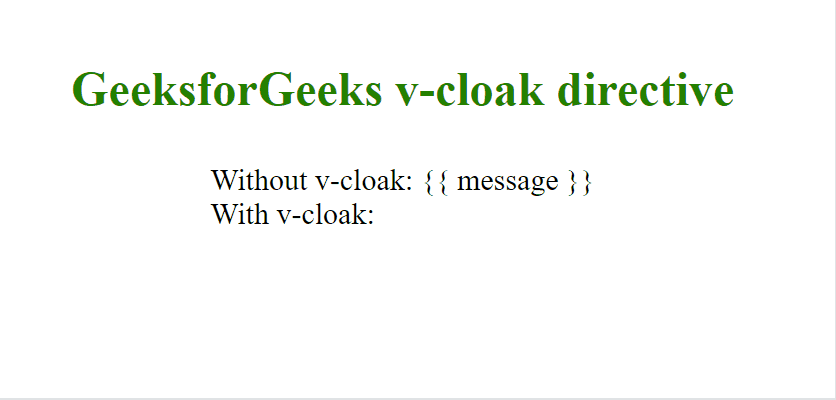
Approach 2: Using a combination of CSS and Vue.js lifecycle hooks
Another method to achieve a similar effect of hiding Vue.js syntax during page load is to use the mounted lifecycle hook along with some CSS in your Vue instance. It conditionally renders the Vue.js template based on the value of the display property in the CSS stylesheet rule, allowing you to control the visibility of the content during the initial page load.
Syntax:
<span class="CSSClassToHideMessageTillContentLoads">{{textMessage}}</span>
Example: The following example demonstrates the usage of the Vue.js mounted lifecycle hook in conjuction with CSS classes and rules in Vue.js to hide the Vue.js syntax while the page is loading.
HTML
<!DOCTYPE html>
< html >
< head >
< meta charset = "utf-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< style >
body {
height: 90vh;
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
}
h1 {
color: green;
}
.hidden {
display: none;
}
#app {
padding: 10px;
font-size: 1.25rem;
}
</ style >
< title >vue-geeksforgeeks</ title >
</ head >
< body >
< h1 >
GeeksforGeeks mounted
lifecycle hook in Vue.js
</ h1 >
< div id = "app" >
< div >
Without CSS and mounted
hook: {{ message }}
</ div >
< div >
With CSS and mounted hook:
< span class = "hidden" >
{{ message }}
</ span >
</ div >
</ div >
< script src =
</ script >
< script >
setTimeout(() => {
const app = Vue.createApp({
data() {
return {
message:
'This is a sample message'
};
},
mounted() {
// Select the element to hide
// till page has loaded
document.querySelector('#app div span')
.classList.remove('hidden');
}
});
const vm = app.mount('#app');
}, 2000)
</ script >
</ body >
</ html >
|
Output:
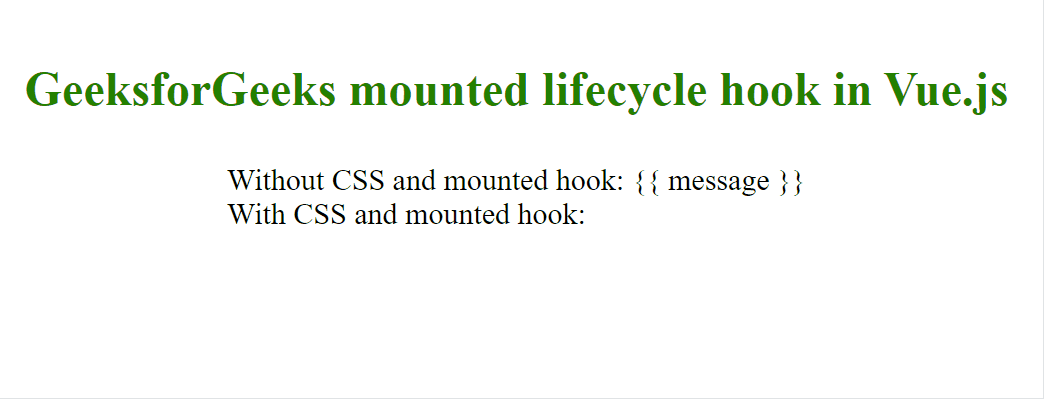
Share your thoughts in the comments
Please Login to comment...