How to Generate Random Number from Standard Distributions in R
Last Updated :
27 Sep, 2023
Random number generation is a crucial aspect of statistical simulations and data analysis. R languauge is a powerful statistical computing language, generating random numbers from standard distributions is a routine task.
Standard Distribution
A “standard” probability distribution refers to a specific type of probability distribution that has been standardized or normalized in a way that allows for easy comparison or analysis. For example, in the case of the normal distribution, a standard normal distribution has a mean (average) of 0 and a standard deviation of 1.
Standard distributions in R language basically five types like – Normal, Uniform ,Exponential,Binomial and Poisson.
Normal Distribution (Gaussian Distribution)
Normal Distribution is a continuous probability distribution that is symmetric and bell-shaped. It is characterized by its mean (μ) and standard deviation (σ). The standard normal distribution is a specific form of the normal distribution with μ = 0 and σ = 1.

Where:
Uniform Distribution
Uniform distribution is a probability distribution where all outcomes are equally likely. For example, rolling a fair six-sided die has a uniform distribution because each side has an equal chance of landing face up.
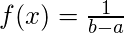
Where:
Exponential Distribution
Exponential distribution is a continuous probability distribution that describes the time between events in a Poisson process. It is often used in situations involving the modeling of time intervals between events.

Where:
Binomial Distribution
Binomial distribution is a discrete probability distribution that describes the number of successes in a fixed number of independent Bernoulli trials (experiments with two possible outcomes, typically success or failure).

Where:
- n: number of trials
- p: probability of success
- q: probability of failure (p=1-q)
- X: number of successes in n trials
Poisson Distribution
Poisson distribution is a discrete probability distribution that describes the number of events occurring in a fixed interval of time or space, given a constant average rate of occurrence.

Where:
To get started, follow these necessary steps:-
Install and Load R Packages
R
install.packages ( 'stats' )
library (stats)
|
First ensure you have R installed. Load the necessary packages using the “install.packages()” and “library()”commands for specific distributions like ‘stats’ for Normal distribution, Uniform distribution, and Exponential distribution.
Generate Random Numbers
Normal Distribution
Syntax:
rnorm(n, mean, sd)
where
- n is the number of observations,
- mean is the mean of the distribution, and
- sd is the standard deviation.
R
print ( rnorm (100, mean = 0, sd = 1))
|
Output:
[1] 0.758942529 -0.453723834 0.912949296 0.717898789 0.791543559 -0.550075758 0.870248179 -0.466166451
[9] -0.895218780 -0.337561284 0.717595419 0.133850963 0.681790129 1.255716716 0.108504918 -1.370314518
[17] -1.191296124 -0.073101164 -1.737569385 0.183472925 0.880325791 0.040857115 1.241827081 0.196701663
[25] 2.040058850 1.526720480 -0.141765992 0.301710157 -0.328031397 0.764369404 0.258604662 -0.198845302
[33] 0.003747265 -0.107614661 -1.621863906 -0.660088485 -1.459307096 -0.564311991 1.316207435 -0.062338832
[41] 0.443187066 0.172675253 0.261057092 2.154863589 -1.040186863 0.922992861 -1.040073052 -1.925036595
[49] -0.764804674 1.878344170 -1.779070294 1.273924001 -0.343380947 0.069711619 1.106903432 -0.155138403
[57] 0.832997857 0.383870496 -0.141183939 0.559139232 -0.344832450 2.457222849 1.108045131 1.865635601
[65] 1.243450462 0.563266845 0.806369221 -1.309192943 -1.290963436 0.700363377 0.658688551 -0.188743862
[73] 0.245341003 -0.698437649 -1.414918391 0.217500414 0.418729014 0.735709031 -0.549660892 0.874029993
[81] 0.628098569 -0.584199721 -0.380086985 0.665529536 0.476385817 0.663277136 -1.177093758 0.237022601
[89] 0.548552073 -0.978999404 -0.578105278 0.110176802 0.855077220 -0.711799781 -0.710650271 1.163810670
[97] 0.901417598 -0.788738636 -0.376138918 -0.141028442
We generated 100 random numbers from normal distribution
Uniform Distribution
Syntax:
runif(n, min, max)
where:
- n is the number of observations,
- min is the lower bound, and
- max is the upper bound.
R
random_uniform <- runif (50, min = 0, max = 1)
print (random_uniform)
|
Output:
[1] 0.50676992 0.69605063 0.29005553 0.34869535 0.90484370 0.85910707 0.70495214 0.79182671 0.01119734 0.40899106
[11] 0.81495474 0.05490031 0.74002386 0.07122289 0.78516947 0.85784466 0.42315433 0.62709236 0.80041710 0.91264925
[21] 0.94827721 0.22207699 0.38470803 0.09788792 0.15572991 0.73780769 0.11070697 0.68107977 0.05804994 0.73271743
[31] 0.04849818 0.60440056 0.15566900 0.51244807 0.79079711 0.77810000 0.61108455 0.07438912 0.05738890 0.52954094
[41] 0.58058220 0.79062618 0.04315235 0.36860145 0.57379876 0.79154094 0.74178902 0.90239004 0.49193354 0.49266193
Generating 50 random numbers from a Uniform distribution between 0 and 1
Exponential Distribution:
Syntax:
rexp(n, rate)
where:
- rate: represents the shape.
- n: Specify sample size
R
random_exponential <- rexp (75, rate = 0.5)
print (random_exponential)
|
Output:
[1] 0.599724626 2.372824108 1.814010210 4.975601312 6.970033478 0.960039768 1.507752137 1.145074962 1.181036658
[10] 0.219497249 5.237886768 1.807545520 1.423358488 2.338900728 4.136314439 0.654886615 2.485255983 0.481323684
[19] 5.305394348 2.327880467 1.278035887 1.140645239 0.712455931 1.401187526 0.544935975 3.360479098 0.758573133
[28] 0.796037794 0.206771806 3.823335115 1.081069200 0.968634650 5.143709499 3.140314169 0.070044713 6.066294968
[37] 0.344958772 0.925376899 0.534956788 1.658513910 0.805072598 0.167028401 3.483325886 1.798591476 4.677147258
[46] 0.675503581 5.517120767 1.864244714 1.922077855 0.505001211 0.179893075 0.859651250 2.106099440 0.656650158
[55] 2.977580065 2.396910693 0.332511657 0.368489315 0.479358466 0.001085686 1.294693221 3.342880194 1.212357279
[64] 0.283231467 3.109467693 1.061213733 0.047089372 0.978645205 2.109215490 1.196788696 0.355254514 3.545218974
[73] 2.073593198 2.528796159 0.131268121
We are generating 75 random numbers from an Exponential distribution with rate parameter 0.5
Binomial Distribution
Syntax:
rbinom(n, size, prob)
Where:
- n is number of observations.
- size is the number of trials.
- prob is the probability of success of each trial.
The “rbinom” function generates random numbers following a binomial distribution.
R
p_success <- 0.5
n_trials <- 10
random_binomial <- rbinom (1, n = n_trials, prob = p_success)
print (random_binomial)
|
Output :
[1] 0 0 0 1 1 0 0 0 0 0
The output, which is stored in random_binomial, represents the number of times you obtained heads (success) in 10 simulated coin flips. Since you are generating only one random value, it will be either 0 (no heads) or 1 (one head) based on the probability of success. For a fair coin (p_success = 0.5), the output will vary between 0 and 1 with roughly equal probability.
Poisson Distribution
Syntax:
rpois(n, lambda)
Where:
- n: number of random variables to generate
- lambda: average rate of success
R
lambda <- 10
time_period <- 1
random_poisson <- rpois (10, lambda = lambda * time_period)
print (random_poisson)
|
Output:
[1] 9 10 8 10 9 13 6 14 12 17
The average rate of calls per hour (lambda) is set at 10. The “rpois” function generates 10 random numbers following a Poisson distribution.
Share your thoughts in the comments
Please Login to comment...