How to Generate and Validate OTPs in Node.js with ‘Speakeasy’ Module ?
Last Updated :
10 May, 2023
Speakeasy is a very important and useful npm module that can generate and validate OTPs (One Time Passwords). OTPs are mainly used for security-related purposes. This module basically supports two types of OTPs: TOTP and HOTP. The main difference between these two types of OTPs is TOTP generates a time-based OTP code based on a secret key and current time, on the other hand, HOTP generates a counter-based OTP code based on a secret key and a counter value.
Applications of this module:
- Generating a secret key: Using ‘speakeasy’ you can generate a secret key. You can do this using the speakeasy.generateSecret() function. This function returns an object that contains a base32-encoded secret key and a QR code URL that you can use to display the QR code to the user.
- Generating a one-time password: After you have generated a secret key, you can use the speakeasy.totp() function to generate a one-time password (OTP) based on the current time.
- Verifying a one-time password: To verify a one-time password that the user has entered, you can use the speakeasy.totp.verify() function.
How to Generate and Validate OTPs in Node.js with ‘Speakeasy’ module?
Installation: To use any package or module, we have to install it first. We can install the ‘speakeasy’ module using the command given below. After successful installation, a JSON file is added to the directory which contains all the details of the installed packages or modules.
$ npm install speakeasy
-300.png)
Installation
Use the module: After successful installation, we can use this module in our project. To use this package, firstly we have to import the installed package into the project using the require function. Then we have generated a secret key using the generateSecret() function by passing the length in it and TOTP code using the secret key generated by passing the encoding format in it. At last show the output of secret and code in the console.
Javascript
const speakeasy = require( 'speakeasy' );
const secret = speakeasy.generateSecret({ length: 20 });
const code = speakeasy.totp({
secret: secret.base32,
encoding: 'base32'
});
console.log( 'Secret: ' , secret.base32);
console.log( 'Code: ' , code);
|
Run the code: After successfully using this package in the code, we have to run our javascript code file using the given command in the terminal.
$ node index.js
where index.js is the javascript file name.
Output:
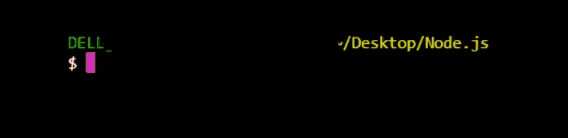
output
We can see the output, a six-digit code has been generated using this module.
Conclusion: This is how we can use the ‘speakeasy’ module in Node.js for the generation and validation of OTPs. We can say that ‘Speakeasy’ is a great choice for developers who want to add an extra layer of security to their web applications which support two types of One time Passwords: TOTP and HOTP. Speakeasy uses industry-standard algorithms and protocols to ensure that the authentication process is secure and reliable.
Share your thoughts in the comments
Please Login to comment...