How to Escape Double Quotes in Scala?
Last Updated :
26 Mar, 2024
In this article, we will learn how to escape double quotes in Scala. Escaping double quotes means adding a special character or sequence before a double quote within a string to prevent it from being interpreted as the end of the string.
Escape Double Quotes in Scala
Below are the possible approaches to escape double quotes in Scala.
Approach 1: Using Backslashes
- In this approach, we’re using backslashes to escape double quotes within a string.
- This makes sure that the double quotes inside the string are treated as literal characters and not as delimiters for the string.
- For example, the string “GeeksforGeeks is “awesome” Portal” will be printed as “GeeksforGeeks is “awesome” Portal” with the double quotes included in the output.
In the below example, backslashes are used to escape double quotes.
Scala
// Creating object
object GFG {
// Main method
def main(args: Array[String]): Unit = {
// Input String
val str = "GeeksforGeeks is \"awesome\" Portal"
// Output
println(str)
}
}
Output:
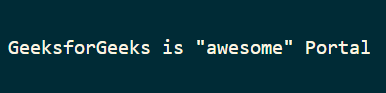
Approach 2: Using Triple Quotes
- In this approach, we’re using triple quotes (“””) to enclose the string.
- Triple quotes allow the string to contain special characters like double quotes without the need for escaping.
- Therefore, the string “GeeksforGeeks is “awesome” Portal” will be printed as it is, including the double quotes, without requiring explicit escaping.
In the below example, triple quotes are used to escape double quotes.
Scala
// Creating object
object GFG {
// Main method
def main(args: Array[String]): Unit = {
// Input String
val str = """GeeksforGeeks is "awesome" Portal"""
// Output
println(str)
}
}
Output:
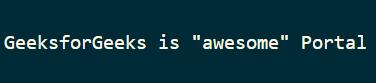
Approach 3: Using String Interpolation
- In this approach, we are using string interpolation with the “s” prefix.
- By using ${‘”‘} within the string, we can directly insert double quotes without the need for escaping.
In the below example, string interpolation with ${‘”‘} are used to escape double quotes.
Scala
// Creating object
object GFG {
// Main method
def main(args: Array[String]): Unit = {
// Input String
val str = s"GeeksforGeeks is ${'"'}awesome${'"'} Portal"
// Output
println(str)
}
}
Output:
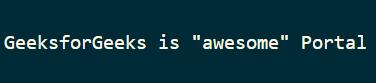
Share your thoughts in the comments
Please Login to comment...