How to edit a JavaScript Alert Box Title in Bootstrap ?
Last Updated :
01 May, 2024
JavaScript alert boxes are an essential component, frequently used to deliver important messages or notifications to users. There are two methods to edit the title of a JavaScript alert box.
First, you can create a custom modal dialog, offering complete control over appearance and functionality. Alternatively, you can use a library like SweetAlert, providing more customization options than the default alert function.
Creating a Custom Alert Function
In this approach, HTML, CSS, and JavaScript are used to create an alert box with a custom title and message. The HTML creates a custom alert with a title, message, and a close button. CSS styles make it look good and position it in the middle. JavaScript functions control when to show or hide the alert. When you click Show Alert, it pops up clicking OK hides it.
Example: The example below shows how to Edit a JavaScript Alert Box Title using the Custom Alert Function.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Custom Alert Box</title>
<link href=
"https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/css/bootstrap.min.css"
rel="stylesheet">
</head>
<body>
<div class="text-center mt-5">
<h2 class="text-success">
GeeksforGeeks
</h2>
<p>
How to edit a JavaScript
alert box title?
</p>
<button onclick="showAlert()"
class="btn btn-success">
Show Alert
</button>
</div>
<div id="customAlert" class="p-5 bg-light mx-auto
w-50 mt-5 text-center
d-none">
<div>
<div>
<h4>GeeksforGeeks</h4>
</div>
<div>
Welcome to GeeksforGeeks! Explore our
vast collection of articles, tutorials,
and coding challenges to enhance your
programming skills.
</div>
<button onclick="closeAlert()"
class="mt-2 btn btn-success">
OK
</button>
</div>
</div>
<script>
function showAlert() {
document.getElementById('customAlert')
.classList.remove('d-none');
}
function closeAlert() {
document.getElementById('customAlert')
.classList.add('d-none');
}
</script>
</body>
</html>
Output:
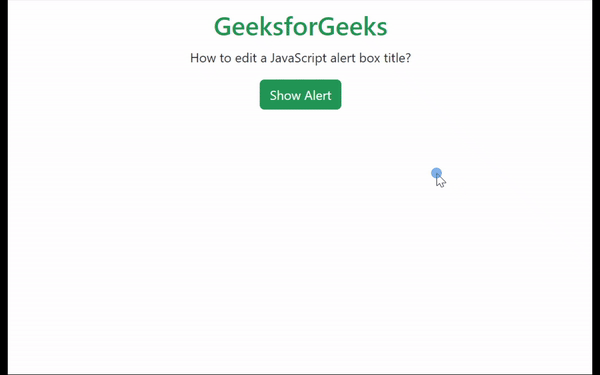
Output
Using SweetAlert Library
In this approach, use SweetAlert to make cool pop-up boxes in your web project. Get it by adding its files to your HTML, using npm/yarn, or linking to a CDN. The HTML creates a page with a title, paragraph, and a button. CSS styles position everything nicely, especially the button. When you click the button, JavaScript makes a cool pop-up using SweetAlert with a custom message.
SweetAlert CDN link:
<script src="https://cdn.jsdelivr.net/npm/sweetalert2@11"></script>
Example: The example below shows how to Edit a JavaScript Alert Box Title using SweetAlert Library.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Alert Box</title>
<link href=
"https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/css/bootstrap.min.css"
rel="stylesheet" integrity=
"sha384-QWTKZyjpPEjISv5WaRU9OFeRpok6YctnYmDr5pNlyT2bRjXh0JMhjY6hW+ALEwIH"
crossorigin="anonymous">
<script src="https://cdn.jsdelivr.net/npm/sweetalert2@11"></script>
</head>
<body>
<div>
<div>
<div class="text-center mt-5">
<h2 class="text-success">
GeeksforGeeks
</h2>
<p>
How to edit a JavaScript
alert box title?
</p>
<button onclick="showAlert()"
class="btn btn-success">
Show Alert
</button>
</div>
</div>
</div>
<script>
function showAlert() {
Swal.fire({
title: 'GeeksforGeeks',
text: 'Explore our vast collection of articles, tutorials, and coding challenges to enhance your programming skills.',
icon: 'info',
confirmButtonText: 'Close'
});
}
</script>
</body>
</html>
Output:
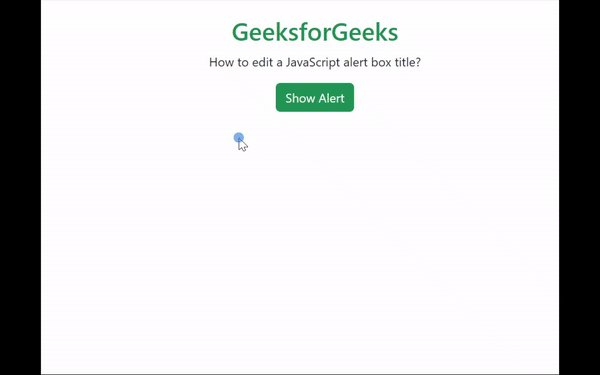
Output
Share your thoughts in the comments
Please Login to comment...