How to display XML data in web page using PHP ?
Last Updated :
31 Mar, 2021
In this article, we are going to display data present in an XML file on a web page using PHP through the XAMPP server. PHP is a server-side scripting language that is mainly for processing web data. The XML stands for an extensible markup language.
Requirements:
Syntax:
<root>
<child>
<subchild>.....</subchild>
</child>
</root>
Approach: We are going to use mainly two functions in our PHP code. The simplexml_load_file() function is used to convert an XML document to an object.
Steps to execute:
Output: Open your browser and type localhost/geek/code.php to see the output.
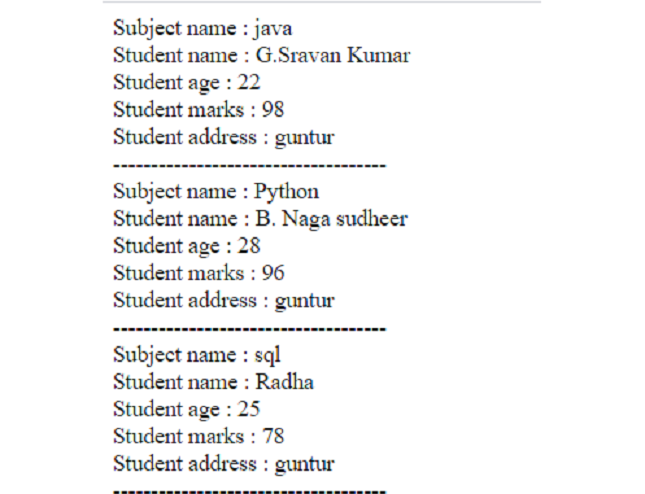
XML data
Share your thoughts in the comments
Please Login to comment...