How to Display Three Bootstrap Cards in a Column ?
Last Updated :
16 Apr, 2024
In Bootstrap, aligning the three cards in a column is important to create a vertical layout for displaying content on smaller screen devices or to make the web page responsive and compatible for all devices.
Use the below approaches to display three Bootstrap cards in a column:
Using Grid Classes
In this approach, we are using Bootstrap’s grid system by wrapping the cards in a col-md-6 class, which creates a single column on medium-sized screens and above. The justify-content-center class aligns the column horizontally in the center of the row.
Example: The below example uses bootstrap grid classes to display three Bootstrap cards in a column.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 1</title>
<link rel="stylesheet" href=
"https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css">
</head>
<body>
<div class="container">
<h1 class="text-center mb-4">
Using Grid Classes
</h1>
<h2 class="text-center mb-4">
Aligning cards in a single column
</h2>
<div class="row justify-content-center">
<div class="col-md-6">
<div class="card mb-3 border-primary">
<div class="card-body">
<h5 class="card-title text-primary">
JavaScript
</h5>
<p class="card-text">
JavaScript is a versatile programming
language used for web development.
</p>
</div>
</div>
<div class="card mb-3 border-danger">
<div class="card-body">
<h5 class="card-title text-danger">
Python
</h5>
<p class="card-text">
Python is known for its simplicity and
readability, widely used in data
science and AI.
</p>
</div>
</div>
<div class="card mb-3 border-warning">
<div class="card-body">
<h5 class="card-title text-warning">
Java
</h5>
<p class="card-text">
Java is a robust, object-oriented
programming language used for building
enterprise-scale applications.
</p>
</div>
</div>
</div>
</div>
</div>
</body>
</html>
Output:
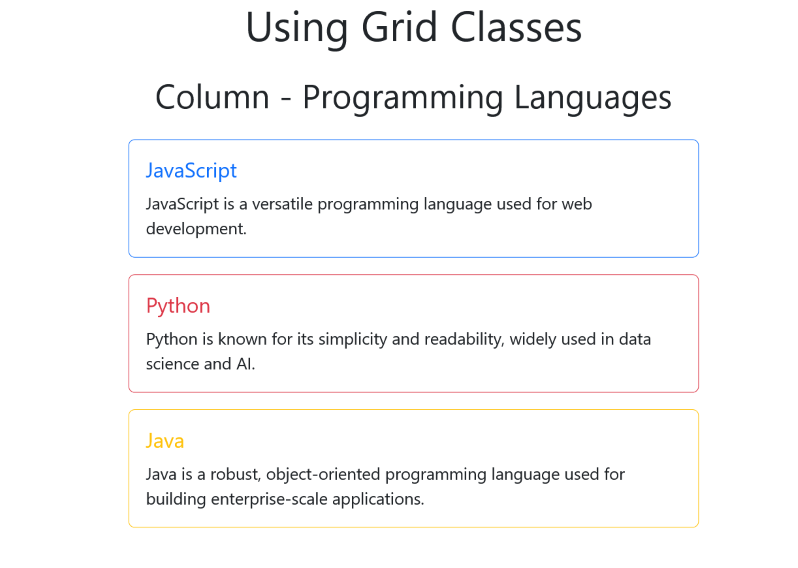
Using FlexBox Classes
In this approach, we are using Flexbox classes to arrange three Bootstrap cards vertically within a single column. There are multiple flexbox classes used to align cards in a single column.
Example: The below example uses Bootstrap FlexBox Classes to display three Bootstrap cards in a column.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 2</title>
<link rel="stylesheet" href=
"https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css">
</head>
<body>
<div class="container">
<h1 class="text-center mb-4">
Using FlexBox Classes
</h1>
<div class="row">
<div class="col-md-6 d-flex flex-column
justify-content-start">
<h2 class="text-center mb-3">
Column 1 - Programming Languages
</h2>
<div class="card border-primary mb-3">
<div class="card-body">
<h5 class="card-title text-primary">
JavaScript
</h5>
<p class="card-text">
JavaScript is a versatile programming
language used for web development.
</p>
</div>
</div>
<div class="card border-danger mb-3">
<div class="card-body">
<h5 class="card-title text-danger">
Python
</h5>
<p class="card-text">
Python is known for its simplicity and
readability, widely used in data
science and AI.
</p>
</div>
</div>
<div class="card border-warning mb-3">
<div class="card-body">
<h5 class="card-title text-warning">
Java
</h5>
<p class="card-text">
Java is a robust, object-oriented
programming language used for building
enterprise-scale applications.
</p>
</div>
</div>
</div>
<div class="col-md-6 d-flex flex-column
justify-content-start">
<h2 class="text-center mb-3">
Column 2 - Scripting Languages
</h2>
<div class="card border-success mb-3">
<div class="card-body">
<h5 class="card-title text-success">
Ruby
</h5>
<p class="card-text">
Ruby is a dynamic, reflective, object-oriented,
and general-purpose scripting language.
</p>
</div>
</div>
<div class="card border-info mb-3">
<div class="card-body">
<h5 class="card-title text-info">
PHP
</h5>
<p class="card-text">
PHP is widely used for web development and
can be embedded into HTML.
</p>
</div>
</div>
<div class="card border-secondary mb-3">
<div class="card-body">
<h5 class="card-title text-secondary">
Perl
</h5>
<p class="card-text">
Perl is known for its text processing
capabilities and used in system
administration tasks.
</p>
</div>
</div>
</div>
</div>
</div>
</body>
</html>
Output:
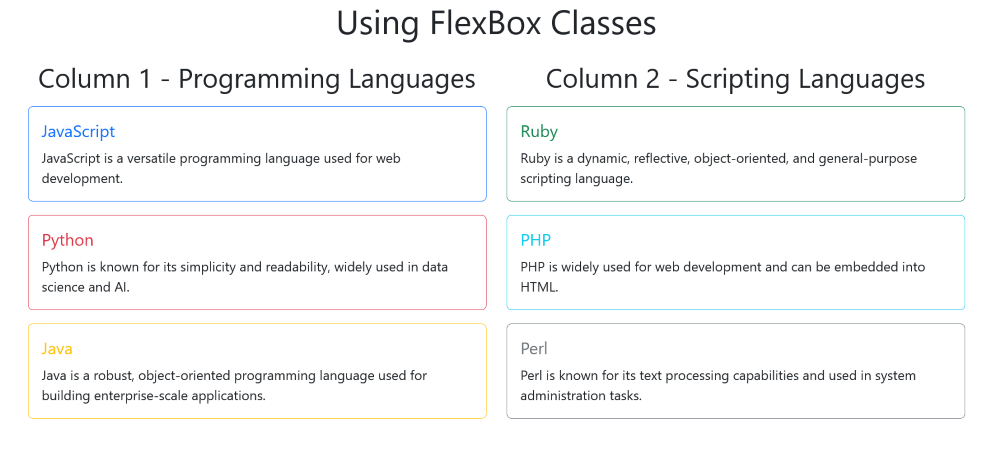
Share your thoughts in the comments
Please Login to comment...