How to detect if a dropdown list is a multi-select using jQuery?
Last Updated :
15 May, 2023
In this article, we will learn how to detect if a dropdown list or an HTML select element is a multi-select one or not using jQuery. There is one main approach with which this can be implemented.
Approach: Using the prop() method in the jQuery library. There is one select element with an id of dropdown defined in the following example which also has an attribute of multiple attached to it. Also, a button element with a class of check-multi-select is created which on click checks whether the given dropdown is a multi-select one or not. We use the prop() method to check the existence of the multiple attributes bypassing the same as a string parameter. If the method returns the boolean true, then the dropdown list is a multi-select. Otherwise, the dropdown list is not a multi-select since the method returns the boolean false which indicates the absence of the multiple attributes.
Example 1: In this example, we will use the above approach.
HTML
<!DOCTYPE html>
< html >
< head >
< style >
body {
text-align: center;
}
p {
font-size: 25px;
font-weight: bold;
}
select {
display: block;
margin: 0 auto;
}
button {
margin-top: 1rem;
cursor: pointer;
}
</ style >
</ head >
< body >
< h1 style = "color: green" >GeeksforGeeks</ h1 >
< p >jQuery - Detect if a dropdown is a multi-select</ p >
< select id = "dropdown" multiple>
< option value = "Geek 1" >Geek 1</ option >
< option value = "Geek 2" >Geek 2</ option >
< option value = "Geek 3" >Geek 3</ option >
< option value = "Geek 4" >Geek 4</ option >
</ select >
< button class = "check-multi-select" >
Click me to check if dropdown is a multi-select
</ button >
< script src =
</ script >
< script type = "text/javascript" >
$(".check-multi-select").click(function () {
if ($("#dropdown").prop("multiple")) {
alert(
"The given dropdown IS a multi-select"
);
} else {
alert(
"The given dropdown IS NOT a multi-select"
);
}
});
</ script >
</ body >
</ html >
|
Output:
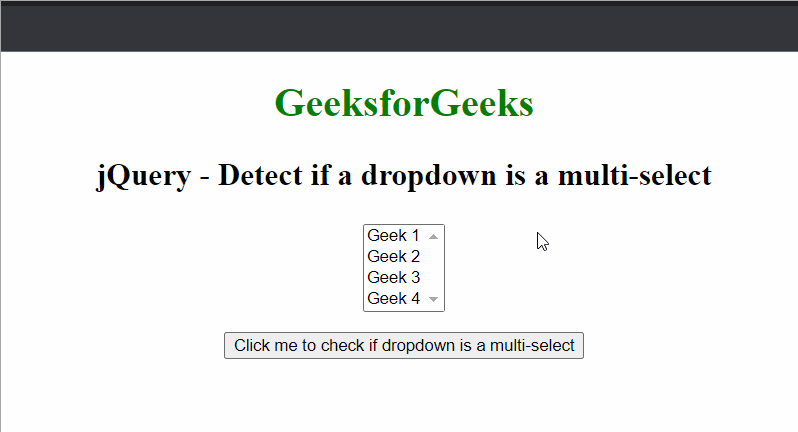
Example 2: This example is quite similar to the previous example but the only difference is that the dropdown is defined as not a multi-select which means there are no multiple attributes attached to it. Hence, the alert message states that the given dropdown list is not a multi-select.
HTML
<!DOCTYPE html>
< html >
< head >
< style >
body {
text-align: center;
}
p {
font-size: 25px;
font-weight: bold;
}
select {
display: block;
margin: 0 auto;
}
button {
margin-top: 5rem;
cursor: pointer;
}
</ style >
</ head >
< body >
< h1 style = "color: green" >GeeksforGeeks</ h1 >
< p >jQuery - Detect if a dropdown is a multi-select</ p >
< select id = "dropdown" >
< option value = "Geek 1" >Geek 1</ option >
< option value = "Geek 2" >Geek 2</ option >
< option value = "Geek 3" >Geek 3</ option >
< option value = "Geek 4" >Geek 4</ option >
</ select >
< button class = "check-multi-select" >
Click me to check if dropdown is a multi-select
</ button >
< script src =
</ script >
< script type = "text/javascript" >
$(".check-multi-select").click(function () {
if ($("#dropdown").prop("multiple")) {
alert(
"The given dropdown IS a multi-select"
);
} else {
alert(
"The given dropdown IS NOT a multi-select"
);
}
});
</ script >
</ body >
</ html >
|
Output:
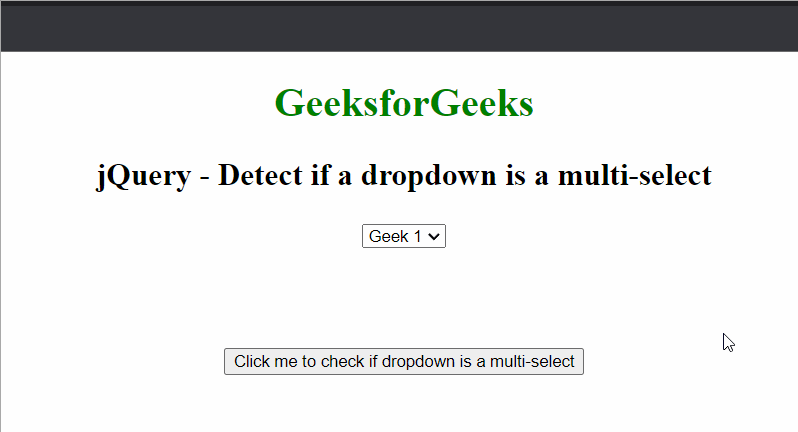
Share your thoughts in the comments
Please Login to comment...