How to Customize the Position of an Alert Box using JavaScript ?
Last Updated :
03 Apr, 2024
In web development, alert boxes are frequently used to inform users of important information. However, alert boxes always appear in the center of the screen by default, which might not be the best arrangement for some layouts. This article explores various methods for achieving this personalization.
Approach: Using CSS
In this approach, the alert box is positioned in relation to its parent element and styled using CSS. The alert box’s location on the screen can be changed by modifying its top and left properties.
Example: This example showcases a custom alert box styled with CSS, triggered by clicking a button.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,
initial-scale=1.0">
<title>Custom Alert Box</title>
<style>
body {
display: flex;
justify-content: center;
width: 100vw;
margin-top: 20px;
}
.alert {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background-color: #f8d7da;
color: #721c24;
padding: 15px 20px;
border: 1px solid #f5c6cb;
border-radius: 5px;
}
</style>
</head>
<body>
<button onclick="showAlert()">Show Alert</button>
<script>
function showAlert() {
const alertBox = document.createElement('div');
alertBox.className = 'alert';
alertBox.textContent = 'This is a custom alert box.';
document.body.appendChild(alertBox);
}
</script>
</body>
</html>
Output:
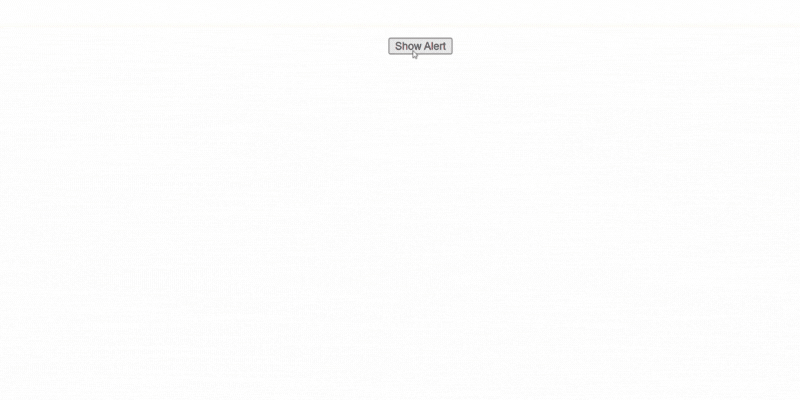
Output
We can also create a custom Alert function for showing the alert box. The custom alert function that opens a modal dialog box in place of using JavaScript’s default alert function. This gives you more options for how to style and arrange the alert box.
Example: This creates a custom alert box, appearing as a modal overlay with a message when the “Show Alert” button is clicked.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,
initial-scale=1.0">
<title>Custom Alert Box</title>
<style>
.modal {
display: none;
position: fixed;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background-color: rgba(0, 0, 0, 0.5);
padding: 20px;
color: #fff;
}
</style>
</head>
<body>
<button onclick="customAlert()">Show Alert</button>
<div id="modal" class="modal"></div>
<script>
function customAlert() {
const modal = document.getElementById('modal');
modal.innerHTML = '<p>This is a custom alert box.</p>';
modal.style.display = 'block';
}
</script>
</body>
</html>
Output:
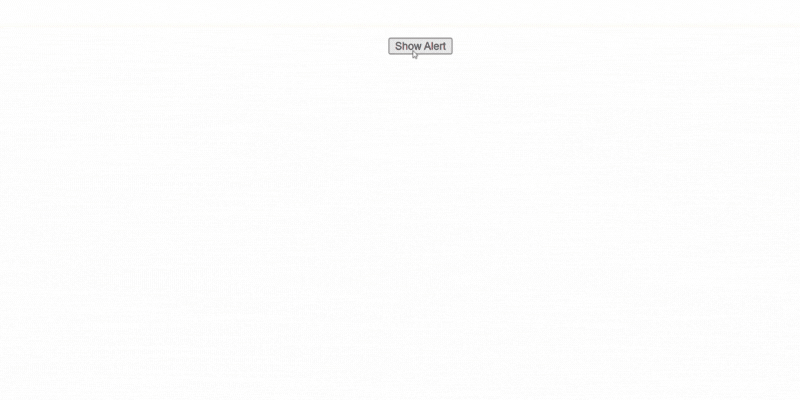
Output
We can also create an Alert using window.alert method. using this method, you can override the alert function’s default behavior and intercept it with your own custom implementation. This gives you the ability to modify the alert box’s position and, if necessary, add new features.
Example: This code implements a custom alert box styled with CSS, appearing centered on the screen when triggered by the “Show Alert” button.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,
initial-scale=1.0">
<title>Custom Alert Box</title>
<style>
.alert {
position: fixed;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background-color: #007bff;
color: #fff;
padding: 15px 20px;
border-radius: 5px;
}
</style>
</head>
<body>
<button onclick="customAlert()">Show Alert</button>
<script>
window.alert = function (message) {
const alertBox = document.createElement('div');
alertBox.className = 'alert';
alertBox.textContent = message;
document.body.appendChild(alertBox);
};
function customAlert() {
alert('This is a custom alert box.');
}
</script>
</body>
</html>
Output:
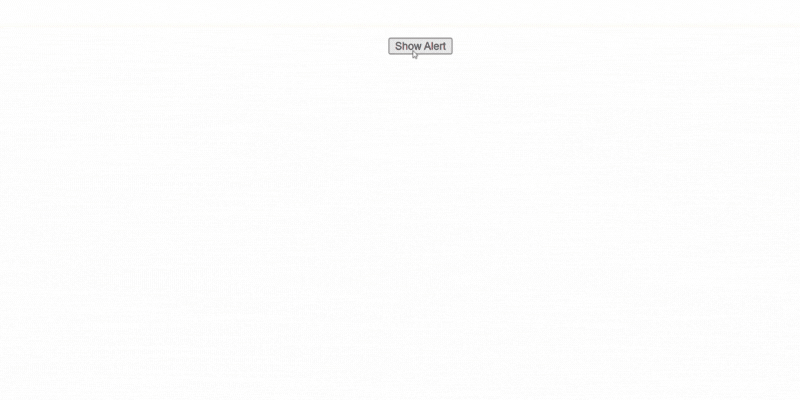
Output
Share your thoughts in the comments
Please Login to comment...