How to Create, Push, and Delete Topics in Amazon SNS with PHP?
Last Updated :
19 Oct, 2023
Amazon SNS is an asynchronous message-passing system that solves the drawback above. The sender is called publisher whereas the receiver is called subscriber. The main advantage of pub-sub is that it decouples the subsystem which means all the components can work independently.AWS Credentials(AWS access key ID & AWS secret key): You need to be an IAM user or make an IAM user and then generate a new access key for that IAM user. Copy all the credentials for future use.
Setting up PHP SDK
There are 3 ways to install a PHP AWS SDK:
- Using a composer
- Through .par file
- Through a zip file
Steps to Create Push Message To A Topic, Delete A Topic In PHP – Amazon SNS
To know how to create an AWS freetier account refer to Amazon Web Services (AWS) – Free Tier Account Set up.
Step 1: Configure AWS Credentials
In order to authenticate SDK with aws account, you need to be an IAM user or set up an IAM user. Now, before moving to our tutorial, we will make a Keys.php file wherein we will define all our keys. You can do it as follows:
PHP
<?php
define( 'AWS_ACCESS_KEY_ID' , '' );
define( 'AWS_SECRET_ACCESS_KEY' , '' );
?>
|
Step 2: Set Up PHP SDK
After this we will make a new index.php file wherein we will write our code. Before that, we need to include our Keys.php file so that we can use our credentials.
PHP
<?php
include_once 'Keys.php' ;
?>
|
Now we need to include the aws sdk so that we can create a sns client. For this we need to install the aws php sdk in the same folder as that of index.php.
PHP
<?php
require 'vendor/aws-autoloader.php' ;
?>
|
Step 3: Include the AWS SDK
I have installed php sdk through zip file that is why I have used this snippet. You can read aws docs if you have used other methods.Now its time to use some of the classes from the SDK which we will need. First, we need to use SnsClient class to create a sns client and AwsException class.
The snippet is as follows:
PHP
<?php
use Aws\Sns\SnsClient;
use Aws\Exception\AwsException;php
?>
|
Step 4: Create an SNS Client
We are all set ! Now we will make a sns client which we will use further to create topic. We need to give our AWS credentials to this client so that our sdk is authenticated.
PHP
<?php
$SnSclient = new SnsClient([
'region' => 'us-east-2' ,
'version' => '2010-03-31' ,
'credentials' => [
'key' => AWS_ACCESS_KEY_ID,
'secret' => AWS_SECRET_ACCESS_KEY
]
]);
?>
|
Replace region with your region. Our Keys.php will take care of the credentials,so no need to change anything there. Next step would be calling a function createTopic on sns client.
PHP
<?php
$topicname = 'myTopic' ;
try {
$result = $SnSclient ->createTopic([
'Name' => $topicname ,
]);
var_dump( $result );
} catch (AwsException $e ) {
error_log ( $e ->getMessage());
}
?>
|
Congratulations, your topic has been created!
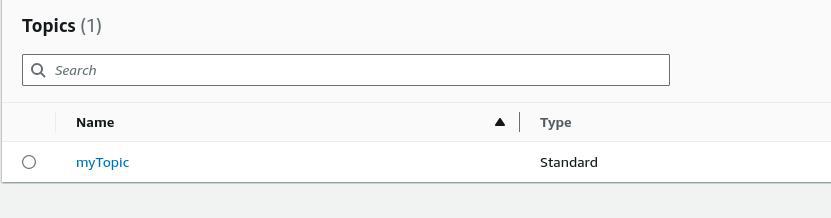
Step 6: Publish a Message To The Topic
Next, we will publish a message to this topic using php. We have already created a sns client, we will call publish function on the client.
PHP
<?php
$message = 'This message is sent from a Amazon SNS code sample.' ;
$topic = ' ' ;
try {
$result = $SnSclient ->publish([
'Message' => $message ,
'TopicArn' => $topic ,
]);
var_dump( $result );
} catch (AwsException $e ) {
error_log ( $e ->getMessage());
}
?>
|
Substitute your topic arn in the topic variable. You can see your topic arn with your topic name as showed in the above image. And now your message is published, you cant see it anywhere on the aws console as its not stored there. For now, if you see a 200 in your terminal after executing the code,that means your message is published. We will talk about seeing the messages when we will talk about making subscriptions. Our last step would be deleting a topic. The snippet for the same is as follows:
PHP
<?php
$topic = ' ' ;
try {
$result = $SnSclient ->deleteTopic([
'TopicArn' => $topic ,
]);
var_dump( $result );
} catch (AwsException $e ) {
error_log ( $e ->getMessage());
}
?>
|
Again we will need a topic arn! You exactly know were to find it. We successfully created a topic, publised message to a topic and deleted a topic using php script.
Conclusion
In this tutorial, we’ve covered the steps to create an Amazon SNS topic, publish a message to it, and delete the topic using PHP. Amazon SNS is a powerful service for sending notifications and messages across distributed systems, and integrating it with PHP provides flexibility in managing your messaging infrastructure.
FAQs On Create, Push, and Delete Topics in Amazon SNS with PHP
1. Can Amazon SNS Be Used With Other AWS Services?
Amazon SNS can be used with other AWS services such as Amazon SQS, Amazon EC2 and Amazon S3.
2. Can Amazon SNS be used with other AWS services?
A topic owner can set explicit permissions to allow more than one user (with a valid AWS ID) to publish to a topic. By default, only topic owners have permissions to publish to a topic.
Share your thoughts in the comments
Please Login to comment...