How to Create Form Layouts with Bootstrap ?
Last Updated :
30 Apr, 2024
To create form layouts with Bootstrap, utilize the grid system to arrange form elements in rows and columns. Apply form control classes for styling inputs, and use spacing utilities for alignment. Customize with Bootstrap’s predefined form styles for consistency.
Below Bootstrap layouts can be used to create a form layout:
Vertical Form Layout
In a vertical form layout, form elements are stacked on top of each other, making efficient use of vertical space. This layout is suitable for forms with a small to moderate number of fields.
Example: The below code creates a vertical form layout using the Bootstrap classes.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width,
initial-scale=1.0">
<title>Sign In</title>
<link href=
"https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/css/bootstrap.min.css"
rel="stylesheet">
<style>
.is-invalid {
border-color: #dc3545;
}
.invalid-feedback {
display: none;
/* Hide validation messages by default */
color: #dc3545;
}
</style>
</head>
<body>
<div class="container">
<h1>Sign In</h1>
<form id="signInForm" novalidate>
<div class="mb-3">
<label for="username" class="form-label">Username</label>
<input type="text"
class="form-control"
id="username"
placeholder="Enter your username" required>
<div class="invalid-feedback">
Please enter a valid username.
</div>
</div>
<div class="mb-3">
<label for="password" class="form-label">Password</label>
<div class="input-group">
<input type="password"
class="form-control"
id="password"
placeholder="Enter your password" required
pattern="(?=.*[A-Z])(?=.*[0-9])(?=.*[^A-Za-z0-9]).{8,}"
title="invalid Password">
<button class="btn btn-outline-secondary"
type="button"
id="togglePassword">
Show
</button>
</div>
<div class="invalid-feedback">
Password must contain at least one
uppercase letter, one digit,
one special character, and be at
least 8 characters long.
</div>
</div>
<div class="mb-3">
<label for="email"
class="form-label">
Email
</label>
<input type="email"
class="form-control"
id="email"
placeholder="Enter your email address" required
pattern="[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$">
<div class="invalid-feedback">
Please enter a valid email address.
</div>
</div>
<button type="submit"
class="btn btn-primary">
Sign In
</button>
</form>
</div>
<script>
const signInForm = document.getElementById('signInForm');
const passwordField = document.getElementById('password');
const togglePasswordButton = document.getElementById('togglePassword');
const inputFields = document.querySelectorAll('input');
togglePasswordButton.addEventListener('click', function () {
if (passwordField.type === 'password') {
passwordField.type = 'text';
togglePasswordButton.textContent = 'Hide';
} else {
passwordField.type = 'password';
togglePasswordButton.textContent = 'Show';
}
});
signInForm.addEventListener('submit', function (event) {
if (!signInForm.checkValidity()) {
event.preventDefault();
event.stopPropagation();
}
signInForm.classList.add('was-validated');
// Show validation feedback messages after form submission attempt
const invalidInputs = signInForm.querySelectorAll(':invalid');
invalidInputs.forEach(function (input) {
input.classList.add('is-invalid');
input.nextElementSibling.style.display = 'block';
});
});
inputFields.forEach(function (input) {
input.addEventListener('input', function () {
if (input.checkValidity()) {
input.classList.remove('is-invalid');
input.nextElementSibling.style.display = 'none';
}
});
});
</script>
</body>
</html>
Output:
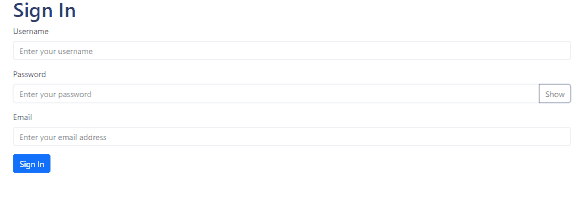
Form Layouts with Bootstrap Example Output
In a horizontal form layout, form elements are arranged side by side, with labels aligned to the left of their corresponding input fields. This layout is ideal for forms with longer labels or when horizontal space is abundant.
Example: The below code implements Bootstrap classes to create a Horizontal form.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,
initial-scale=1.0">
<title>Sign In</title>
<link href=
"https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/css/bootstrap.min.css"
rel="stylesheet">
<style>
.is-invalid {
border-color: #dc3545;
}
.invalid-feedback {
display: none;
/* Hide validation messages by default */
color: #dc3545;
}
</style>
</head>
<body>
<div class="container">
<h1>Horizontal Form Layout</h1>
<form id="signInForm" novalidate>
<div class="row mb-3">
<label for="username" class="col-sm-2 col-form-label">
Username
</label>
<div class="col-sm-10">
<input type="text"
class="form-control"
id="username"
placeholder="Enter your username" required>
<div class="invalid-feedback">
Please enter a valid username.
</div>
</div>
</div>
<div class="row mb-3">
<label for="password" class="col-sm-2 col-form-label">
Password
</label>
<div class="col-sm-10">
<div class="input-group">
<input type="password"
class="form-control"
id="password" placeholder="Enter your password"
required pattern="(?=.*[A-Z])(?=.*[0-9])(?=.*[^A-Za-z0-9]).{8,}"
title="Invalid Password.">
<button class="btn btn-outline-secondary"
type="button"
id="togglePassword">
Show
</button>
</div>
<div class="invalid-feedback">
Password must contain at least one uppercase letter,
one digit, one special character, and be at
least 8 characters long.
</div>
</div>
</div>
<div class="row mb-3">
<label for="email"
class="col-sm-2 col-form-label">
Email
</label>
<div class="col-sm-10">
<input type="email"
class="form-control"
id="email"
placeholder="Enter your email address" required
pattern="[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$">
<div class="invalid-feedback">
Please enter a valid email address.
</div>
</div>
</div>
<div class="row mb-3">
<div class="col-sm-10 offset-sm-2">
<button type="submit" class="btn btn-primary">
Sign In
</button>
</div>
</div>
</form>
</div>
<script>
const signInForm = document.getElementById('signInForm');
const passwordField = document.getElementById('password');
const togglePasswordButton = document.getElementById('togglePassword');
const inputFields = document.querySelectorAll('input');
togglePasswordButton.addEventListener('click', function () {
if (passwordField.type === 'password') {
passwordField.type = 'text';
togglePasswordButton.textContent = 'Hide';
} else {
passwordField.type = 'password';
togglePasswordButton.textContent = 'Show';
}
});
signInForm.addEventListener('submit', function (event) {
if (!signInForm.checkValidity()) {
event.preventDefault();
event.stopPropagation();
}
signInForm.classList.add('was-validated');
// Show validation feedback messages after form submission attempt
const invalidInputs = signInForm.querySelectorAll(':invalid');
invalidInputs.forEach(function (input) {
input.classList.add('is-invalid');
input.nextElementSibling.style.display = 'block';
});
});
inputFields.forEach(function (input) {
input.addEventListener('input', function () {
if (input.checkValidity()) {
input.classList.remove('is-invalid');
input.nextElementSibling.style.display = 'none';
}
});
});
</script>
</body>
</html>
Output:
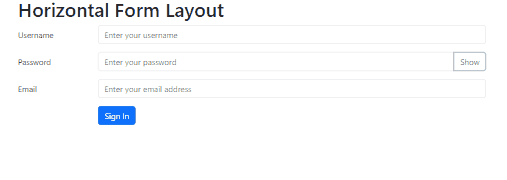
Form Layouts with Bootstrap Example Output
In an inline form layout, form elements are displayed horizontally in a single line, with labels and input fields inline. This layout is useful for compact forms or when horizontal space is limited.
Example: The below code creates a inline form using the Bootstrap form classes.
HTML
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport"
content="width=device-width,
initial-scale=1">
<title>Inline Form</title>
<link rel="stylesheet"
href=
"https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/css/bootstrap.min.css"
integrity=
"sha384-QWTKZyjpPEjISv5WaRU9OFeRpok6YctnYmDr5pNlyT2bRjXh0JMhjY6hW+ALEwIH"
crossorigin="anonymous">
</head>
<body>
<div class="container-fluid bg-success
d-flex justify-content-center
align-items-center"
style="min-height: 100vh;">
<form id="inlineForm"
class="row row-cols-lg-auto
g-3 align-items-center"
onsubmit="return validateForm()">
<div class="col-12">
<label class="visually-hidden"
for="inlineFormInputGroupUsername">
Username
</label>
<div class="input-group">
<div class="input-group-text">@</div>
<input type="text"
class="form-control"
id="inlineFormInputGroupUsername"
placeholder="Username" required>
</div>
</div>
<div class="col-12">
<label class="visually-hidden"
for="inlineFormInputPassword">
Password
</label>
<div class="input-group">
<input type="password"
class="form-control"
id="inlineFormInputPassword"
placeholder="Password" required>
<button type="button"
class="btn btn-outline-secondary
bg-primary
text-light
border-primary"
onclick="togglePassword()">
Show/Hide
</button>
</div>
</div>
<div class="col-12">
<label class="visually-hidden"
for="inlineFormSelectPref">
Preference
</label>
<select class="form-select"
id="inlineFormSelectPref" required>
<option selected disabled>Choose...</option>
<option value="1">Mumbai</option>
<option value="2">Delhi</option>
<option value="3">Noida</option>
</select>
</div>
<div class="col-12">
<button type="submit"
class="btn btn-primary">
Submit
</button>
</div>
</form>
</div>
<script src=
"https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/js/bootstrap.bundle.min.js"
integrity=
"sha384-YvpcrYf0tY3lHB60NNkmXc5s9fDVZLESaAA55NDzOxhy9GkcIdslK1eN7N6jIeHz"
crossorigin="anonymous"></script>
<script>
function validateForm() {
const username = document.getElementById(
"inlineFormInputGroupUsername").value;
const password = document.getElementById(
"inlineFormInputPassword").value;
const preference = document.getElementById(
"inlineFormSelectPref").value;
if (!username || !password || !preference) {
alert("Please fill in all fields");
return false;
}
if (password.length < 8) {
alert("Password must be at least 8 characters long");
return false;
}
return true;
}
function togglePassword() {
const passwordField = document.getElementById("inlineFormInputPassword");
if (passwordField.type === "password") {
passwordField.type = "text";
} else {
passwordField.type = "password";
}
}
</script>
</body>
</html>
Output:
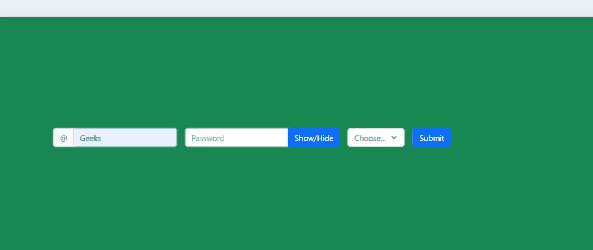
Form Layouts with Bootstrap Example Output
Share your thoughts in the comments
Please Login to comment...