How to create Accordion in ReactJS ?
Last Updated :
22 Mar, 2024
Accordions contain creation flows and allow lightweight editing of an element. Material UI for React has this component available for us, and it is very easy to integrate. We can create it in React using the following approach.
Approach to create Accordion in React JS
To create accordion in react we will
- use the MUI Accordion component.
- use the expand icons, AccordionSummary and AccrodionDetail component from MUI along with related content for a complete working accordion.
- Style the accrodion using CSS and inline styling.
Steps to Create the React Application And Installing Module
Step 1: Initialize and setup the react app using the CRA command.
Step 2: After creating the ReactJS application, Install the material-ui modules using the following command.
npm i @material-ui/core
npm i @material-ui/icons
The updated dependencies in package.json file will look like:
"dependencies": {
"@material-ui/core": "^4.12.4",
"@material-ui/icons": "^4.11.3",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
}
Example: This example demonstrate how to create accordion in react with the help of MUI accordion compoenet. Now write down the following code in the App.js file.
Javascript
// Filename - App.js
import React from "react";
import ExpandMoreIcon from "@material-ui/icons/ExpandMore";
import Accordion from "@material-ui/core/Accordion";
import AccordionDetails from "@material-ui/core/AccordionDetails";
import Typography from "@material-ui/core/Typography";
import AccordionSummary from "@material-ui/core/AccordionSummary";
export default function App() {
return (
<div style={{}}>
<h4>How to create Accordion in ReactJS?</h4>
<Accordion style={{ width: 400 }}>
<AccordionSummary
expandIcon={<ExpandMoreIcon />}
aria-controls="panel1a-content">
<Typography
style={{
fontWeight: 10,
}}>
Accordion Demo
</Typography>
</AccordionSummary>
<AccordionDetails>
<Typography>
Greetings of the day :)
</Typography>
</AccordionDetails>
</Accordion>
</div>
);
}
Step to Run Application: Run the application using the following command from the root directory of the project.
npm start
Output: Now open your browser and go to http://localhost:3000
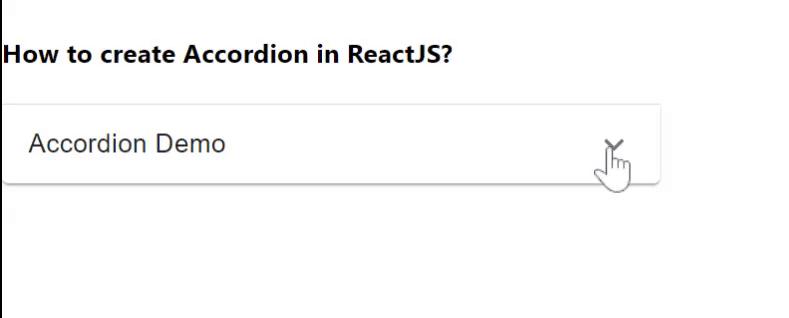
Share your thoughts in the comments
Please Login to comment...