How to create a Snackbar in react native using material design ?
Last Updated :
28 Mar, 2022
React Native is a framework developed by Facebook for creating native-style apps for iOS & Android under one common language, JavaScript. Initially, Facebook only developed React Native to support iOS. However, with its recent support of the Android operating system, the library can now render mobile UI’s for both platforms.
Prerequisites:
- Basic knowledge of ReactJS.
- HTML, CSS and JavaScript with ES6 syntax.
- Node.js should be installed in your system (install).
- Jdk and android studio for testing your app on emulator (install).
Approach: In this article, we will see how to create a snackbar in react native. To create a snackbar, we will use material design library i.e. react-native-paper. Snackbars are messages or feedback about an operation except they provide action to interact with. Snackbar will be displayed at the bottom of the screen and can be swiped off in order to dismiss them.
In our project , we will simply display a button and on click of button ,snackbar will appear at the bottom of the screen. we will the implementation step by step.
Below is the step by step implementation:
Step 1: Create a project in react-native using the following command:
npx react-native init DemoProject
Step 2: Install react-native paper using the following command:
npm install react-native-paper
Step 3: Create a components folder inside your project. Inside the components folder create a file Snackbar.js
Project Structure: It will look like this.
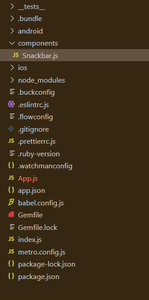
Implementation: Write down the code in respective files.
In Snackbar.js, we have imported Snackbar items directly from the react-native-paper library. It uses the following props :
- visible: It can be true or false to show or hide the Snackbar
- action: Label and press callback for the action button. It should contain the following properties:
- label – Label of the action button
- onPress – Callback that is called when action button is pressed.
- onDismiss: It is a Callback function called when Snackbar is dismissed. The visible prop needs to be updated when this is called.
Filename: Snackbar.js
Javascript
import React, { useState } from "react" ;
import { Text, View, StyleSheet, Dimensions } from 'react-native' ;
import { Snackbar, Button } from "react-native-paper" ;
const screenHeight = Dimensions.get( 'window' ).height;
const SnackbarComponent = () => {
const [snackVisible, setSnackVisible] = useState( false );
return (
<View style={styles.container}>
<View style={styles.btn}>
<Button mode= "contained"
onPress={() => setSnackVisible(!snackVisible)}>
Press
</Button>
</View>
<Snackbar
visible={snackVisible}
onDismiss={() => setSnackVisible( false )}
action={{ label: 'Proceed' }}>
Welcome to GeeksForGeeks
</Snackbar>
</View>
)
}
export default SnackbarComponent;
const styles = StyleSheet.create({
container: {
flexDirection: "column-reverse" ,
justifyContent: "flex-end" ,
height: screenHeight,
},
btn: {
marginVertical: 50,
alignItems: "center"
}
})
|
Now, import this file to your App.js
Filename: App.js
Javascript
import React from 'react' ;
import type { Node } from 'react' ;
import { View } from 'react-native' ;
import SnackbarComponent from './components/Snackbar' ;
const App: () => Node = () => {
return (
<View>
<SnackbarComponent />
</View>
);
};
export default App;
|
Step to run the application: Run the application using the following command :
npx react-native run-android
Output:
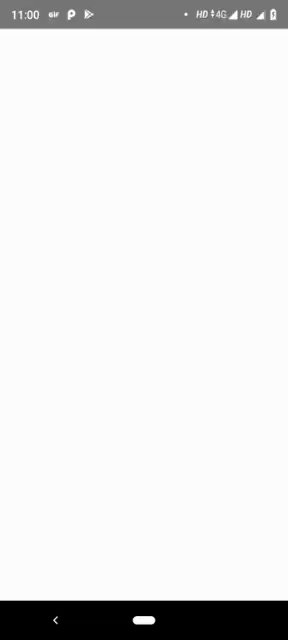
Share your thoughts in the comments
Please Login to comment...