How to create a simple web server that can read a given file on a given disk via “readFile” function ?
Last Updated :
10 Mar, 2022
To create a web server we need to import the ‘http’ module using require() method and create a server using the createServer() method of the HTTP module.
Syntax:
const http = require('http')
const server = createServer(callback).listen(port,hostname);
Now in the callback in the createServer() method, we need to read the file from our local directory and serve it using our server. For this, we need to import the ‘fs’ module and use its readFile() method.
Syntax:
Importing ‘fs’ module:
const fs = require('fs')
fs.readFile():
fs.readFile( filename, encoding, callback_function)
Once we are done reading the file we need to render it on our server.
Project Setup: Create a file serving_files.js and set its directory as your current directory in your terminal.
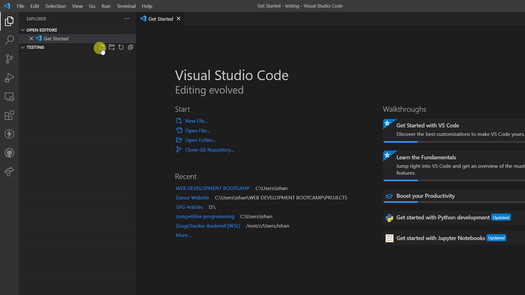
Project setup
Example 1: In this example, we are specifying in our code our filename serving_files.js so when we will run http://localhost/ in our browser, our file will be rendered.
serving_files.js
const fs=require( 'fs' )
const http = require( 'http' )
http.createServer((req, res) =>{
fs.readFile(`serving_files.js`, function (err,filedata) {
if (err) {
res.writeHead(404);
res.end(JSON.stringify(err));
return ;
}
res.writeHead(200);
res.end(filedata);
});
}).listen(80, 'localhost' );
|
Step to run the application: Run the following command in the terminal to start the server:
node serving_files.js
Output:
// Import http and fs
const fs=require('fs')
const http = require( 'http')
// Creating Server
http.createServer((req, res) =>{
// Reading file
fs.readFile(`serving_files.js`, function (err,filedata){
if (err) {
// Handling error
res.writeHead(404);
res.end(JSON.stringify(err));
return;
}
// serving file to the server
res.writeHead(200);
res.end(filedata);
});
}).listen(80,'localhost');
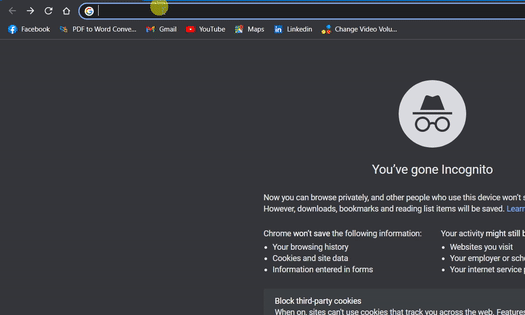
In-Browser Output
Example 2: In this example instead of specifying the file name in our code, we will do it by passing our filename in our request URL. So this way we can access any file from our server by specifying the file name in our request URL and we will not be limited to retrieving only one file.
So to open any file from our server we need to pass our request URL in the following manner:
http://hostname:port/file_name
In our example below our request URL would be http://localhost/serving_files.js
serving_files.js
const fs=require( 'fs' )
const http = require( 'http' )
http.createServer((req, res) =>{
fs.readFile(__dirname + req.url, function (err,filedata) {
if (err) {
res.writeHead(404);
res.end(JSON.stringify(err));
return ;
}
res.writeHead(200);
res.end(filedata);
});
}).listen(80, 'localhost' );
|
Step to run the application: Run the following command in the terminal to start the server:
node serving_files.js
Output:
// Import http and fs
const fs=require('fs')
const http = require( 'http')
// Creating Server
http.createServer((req, res) =>{
// Reading file
fs.readFile(__dirname + req.url, function (err,filedata) {
if (err) {
// Handling error
res.writeHead(404);
res.end(JSON.stringify(err));
return;
}
// serving file to the server
res.writeHead(200);
res.end(filedata);
});
}).listen(80,'localhost');
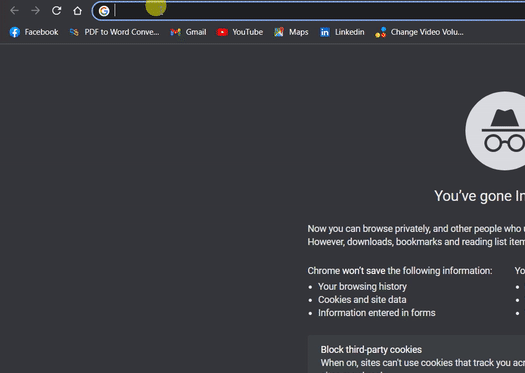
Serving files through request URL
NOTE: In the above examples, we have used port 80 which is the default HTTP port. So even if we do not specify the port in our request URL then it is by default taken as 80.
In case you are using any other port number, specify it in your request URL. For example, if you are using port 8000, then your request URL would be
http://localhost:8000/serving_files.js
Share your thoughts in the comments
Please Login to comment...