How to Create a Chat App using socket.io Node.js ?
Last Updated :
17 Jan, 2023
Socket IO is an event-driven framework used for bidirectional communication between a client and a server. It is mainly used for broadcasting messages to all connected clients in a particular server just like WhatsApp group chats. This framework is event-driven, which means that the client and server will respond to events sent by each other. In this article, we will see how to build a chat app using socket.io and Node JS. As Node JS can handle events, socket.io serves as a great framework for integration with Node JS.
Approach: We will build a web interface for the user to chat through. We will provide text boxes to the user to provide his name and the message that he wants. Then, through the use of socket.io events in Node JS, we will broadcast the messages using our server and display them in the command line or terminal.
Setting up our project:
Step 1: We will start off by creating a new folder called socket-chat-app. Open your command prompt and navigate to the folder we just created.
mkdir socket-chat-app
cd socket-chat-app
Step 2: Now, Initialize the node package by typing in the following command in the terminal:
npm init -y
Step 3: This will create a package.json file for us so that we can install the necessary libraries. Now type in the command:
npm install express socket.io
Step 4: Now, in the socket-chat-app folder, create two files – index.html and index.js:
touch index.html index.js
Project Structure: Finally, your folder structure should look like this:
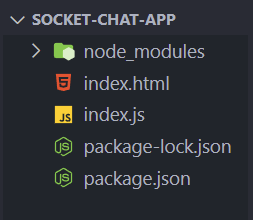
Folder Structure
Implementation: Below is the implementation of the above approach to creating a real-time chat application using socket.io and NodeJs:
- index.html: Inside index.html, we are simply creating a form with two inputs. The user’s name and his message. Inside the script tag, we are using the socket instance to send the user message to our express server and also listen for incoming events from the server to display the username and message on the browser.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Chat app using Socket IO and Node JS</ title >
</ head >
< body >
< h1 class="font-bold text-green-500
text-3xl text-center mt-5">
GeeksforGeeks
</ h1 >
< div >
< h2 class="font-semibold text-xl
text-center mt-5"
id = "logo" >
Chat App using Socket io
</ h2 >
</ div >
< form class="flex flex-col justify-center
items-center mt-5"
id = "form" >
< input class="border border-gray-400
rounded-md mt-5 p-1"
type = "text"
placeholder = "Name"
id = "myname" >
< input class="border border-gray-400
rounded-md mt-5 p-1"
type = "text"
placeholder = "Message"
id = "message" >
< button class="bg-blue-500 rounded-md p-2
text-white mt-5">
Send
</ button >
</ form >
< div class="flex flex-col justify-center
items-center mt-5"
id = "messageArea" >
</ div >
</ body >
< script src = "/socket.io/socket.io.js" ></ script >
< script >
let socket = io();
let form = document.getElementById('form');
let myname = document.getElementById('myname');
let message = document.getElementById('message');
let messageArea = document.getElementById("messageArea");
form.addEventListener("submit", (e) => {
e.preventDefault();
if (message.value) {
socket.emit('send name', myname.value);
socket.emit('send message', message.value);
message.value = "";
}
});
socket.on("send name", (username) => {
let name = document.createElement("p");
name.style.backgroundColor = "grey";
name.style.width = "100%";
name.style.textAlign = "center";
name.style.color = "white";
name.textContent = username + ":";
messageArea.appendChild(name);
});
socket.on("send message", (chat) => {
let chatContent = document.createElement("p");
chatContent.textContent = chat;
messageArea.appendChild(chatContent);
});
</ script >
</ html >
|
- index.js: Inside the index.js file, we are creating an express app and serving our HTML file on the server. We are also creating a socket io instance that listens for the incoming event from index.html and emits events to index.html.
Javascript
const express = require( 'express' );
const app = express();
const { Server } = require( 'socket.io' );
const http = require( 'http' );
const server = http.createServer(app);
const io = new Server(server);
const port = 5000;
app.get( '/' , (req, res) => {
res.sendFile(__dirname + '/index.html' );
});
io.on( 'connection' , (socket) => {
socket.on( 'send name' , (username) => {
io.emit( 'send name' , (username));
});
socket.on( 'send message' , (chat) => {
io.emit( 'send message' , (chat));
});
});
server.listen(port, () => {
console.log(`Server is listening at the port: ${port}`);
});
|
Steps to run the application: Write the below code in the terminal to run the server:
node index.js
Output: Open your browser and open two tabs with the URL: http://localhost:5000 so that we have two clients that can chat with each other. Following is the output of our chat app:
Share your thoughts in the comments
Please Login to comment...