How to Convert HTML Form Field Values to JSON Object using JavaScript?
Last Updated :
18 Apr, 2024
Storing HTML input data into JSON format using JavaScript can be useful in various web development scenarios such as form submissions, data processing, and AJAX requests. Here we will explore how to convert the HTML form input data into the JSON format using JavaScript.
Approach
- The HTML file contains a form with input fields for name, email, and message, and a submit button.
- Upon clicking the submit button, the convertToJson() function is called, defined in an external JavaScript file.
- Inside convertToJson(), it selects the form element and initializes an empty object formData to store input data.
- It iterates over each element in the form using a for loop, excluding the submit button and adds key-value pairs to formData where the key is the element’s name attribute and the value is its value attribute.
- After converting formData to a JSON string using JSON.stringify(), it updates the content of the jsonOutput div with the JSON string wrapped in <pre> tags for better readability.
Example: This example shows the implementation of the above-explained approach.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>The HTML Input to JSON</title>
</head>
<body>
<form id="dataForm">
<label for="name">
Name:
</label>
<input type="text" id="name"
name="name"><br><br>
<label for="email">
Email:
</label>
<input type="email" id="email"
name="email"><br><br>
<label for="message">
Message:
</label><br>
<textarea id="message" name="message"
rows="4" cols="50">
</textarea><br><br>
<button type="button"
onclick="convertToJson()">
Submit
</button>
</form>
<div id="jsonOutput"></div>
<script src="script.js"></script>
</body>
</html>
JavaScript
function convertToJson() {
let form = document.getElementById("dataForm");
let formData = {};
for (let i = 0; i < form.elements.length; i++) {
let element = form.elements[i];
if (element.type !== "submit") {
formData[element.name] = element.value;
}
}
let jsonData = JSON.stringify(formData);
let jsonOutput = document.getElementById("jsonOutput");
jsonOutput.innerHTML = "<pre>" + jsonData + "</pre>";
}
Output:
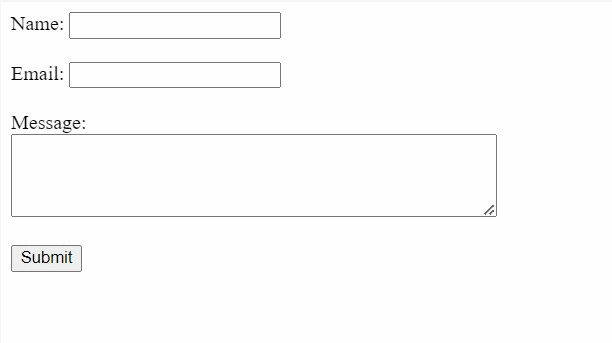
Share your thoughts in the comments
Please Login to comment...