How to Convert CSV to JSON in ReactJS ?
Last Updated :
18 Apr, 2024
Dealing with data in various formats is a common task in web development. CSV (Comma Separated Values) and JSON
(JavaScript Object Notation) are two widely used formats for storing and transmitting data. Converting CSV to JSON is often required when working with data in ReactJS applications.
Approach
- Begin by creating a new React application using the Create React App or any other method you prefer. This will give you the basic structure to work with.
- You’ll need to import the necessary dependencies. In this case, we’re using
React
for building the UI, useState
hook to manage state, and PapaParse
for parsing CSV files. - Build the UI components to display the CSV data and the converted JSON data. Use conditional rendering to display the data only when it’s available. Display the CSV data in a table format and the JSON data in a preformatted text.
- Create a function to handle CSV file uploads. This function will be triggered when a user selects a CSV file. Check if the file is a CSV file and then use
PapaParse
to parse the CSV data. - Write a function to convert the parsed CSV data into JSON format. You can use
JSON.stringify()
to convert the data.
Steps to Create a React Application
Step 1: Create a new React project with the following command.
npx create-react-app myproject
Step 2: Switch to the Project Directory
cd myproject
Step 3: Once you have your project set up, run the following command to install the papa parse package.
npm i papaparse
Project structure
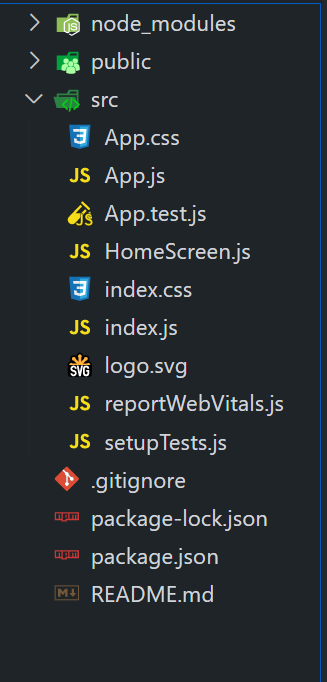
The updated dependencies in package.json file will look like:
{
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"papaparse": "^5.4.1",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-router": "^6.17.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
},
}
Example: In the below example, the Papaparse library is used to parse a CSV file uploaded by the user and convert it into JSON format, with proper file type validation and display of CSV and JSON data in a React.js application.
CSS
/*App.css*/
.container {
max-width: 800px;
margin: 0 auto;
padding: 20px;
background-color: #a8ffb6;
border-radius: 8px;
}
.header {
display: flex;
align-items: center;
justify-content: space-between;
margin-bottom: 20px;
}
.content {
display: grid;
grid-template-columns: repeat(2, 1fr);
gap: 20px;
}
.data-container {
background-color: #fff;
padding: 15px;
border-radius: 8px;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.1);
}
.button {
padding: 10px 20px;
background-color: #fb0000;
color: #fff;
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s ease;
}
table {
width: 100%;
border-collapse: collapse;
}
th,
td {
border: 1px solid rgb(255, 0, 0);
padding: 8px;
}
pre {
white-space: pre-wrap;
}
JavaScript
//App.js
import React, { useState } from 'react';
import Papa from 'papaparse';
import './App.css';
function App() {
const [csvData, setCsvData] = useState([]);
const [jsonData, setJsonData] = useState([]);
const csvUploadFn = (event) => {
const file = event.target.files[0];
const fileType =
file.name.split('.').pop().toLowerCase();
if (fileType !== 'csv') {
alert('Please upload a CSV file.');
return;
}
Papa.parse(file, {
complete: (result) => {
setCsvData(result.data);
},
header: true,
});
};
const conversionFn = () => {
const res = JSON.stringify(csvData, null, 2);
setJsonData(res);
};
return (
<div className="container">
<div className="header">
<h1>CSV to JSON Converter</h1>
<input type="file"
onChange={csvUploadFn}
accept=".csv" />
<button className="button"
onClick={conversionFn}>
Convert to JSON
</button>
</div>
<div className="content">
{csvData.length > 0 && (
<div className="data-container">
<h2>CSV Data</h2>
<table>
<thead>
<tr>
{Object.keys(csvData[0]).map(
(header, index) => (
<th key={index}>
{header}
</th>
))}
</tr>
</thead>
<tbody>
{csvData.map((row, rowIndex) => (
<tr key={rowIndex}>
{Object.values(row).map(
(cell, cellIndex) => (
<td key={cellIndex}>
{cell}
</td>
))}
</tr>
))}
</tbody>
</table>
</div>
)}
{jsonData && (
<div className="data-container">
<h2>JSON Data</h2>
<pre>{jsonData}</pre>
</div>
)}
</div>
</div>
);
}
export default App;
Step to run the application: Open the terminal and type the following command.
npm start
Output:Â This output will be visible on http://localhost:3000/ on browser window.
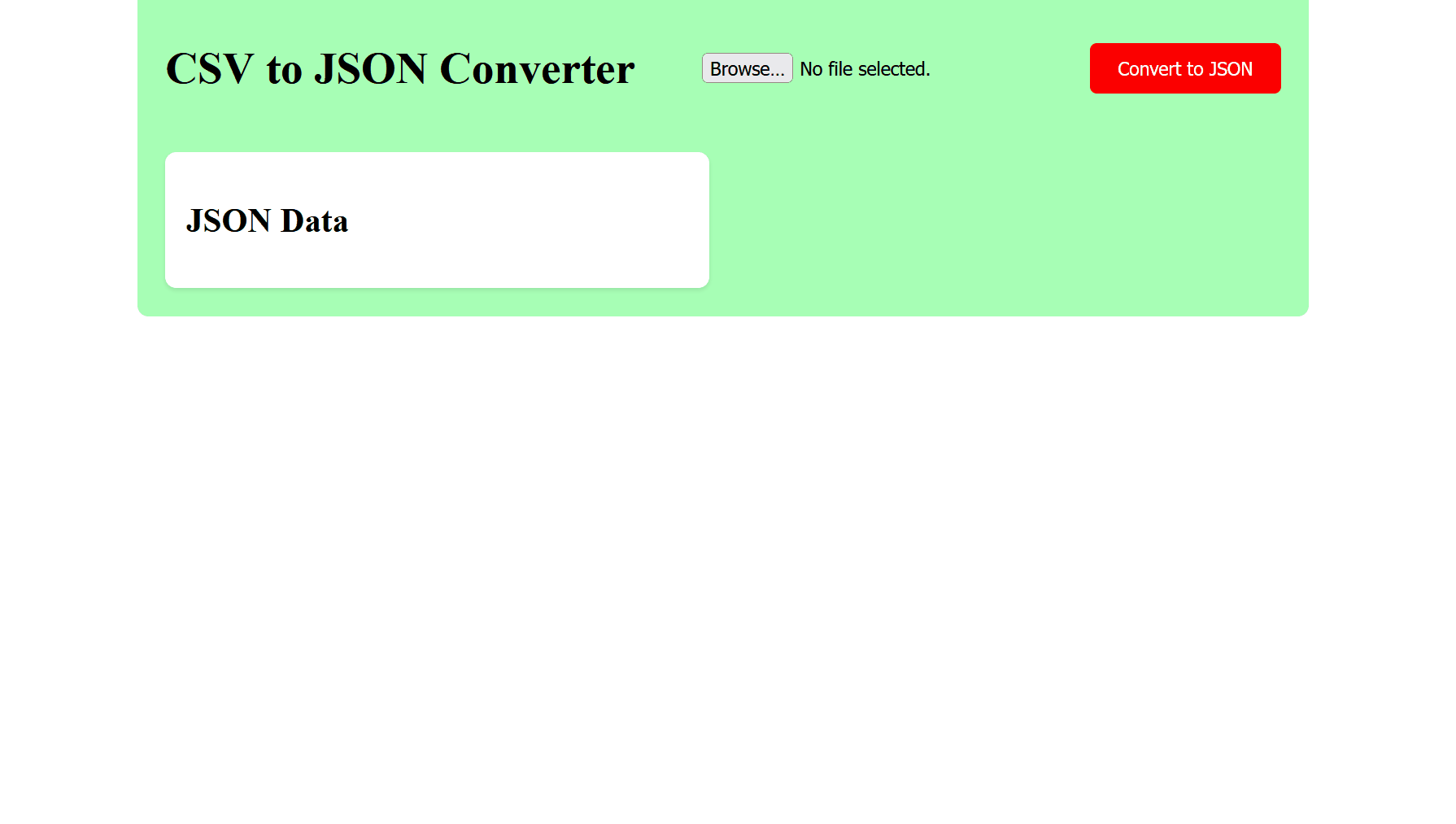
Share your thoughts in the comments
Please Login to comment...