How to connect MySQL database using Scala?
Last Updated :
26 Mar, 2024
MySQL database connectivity using Scala
Introduction:
- Since Scala is interoperable with Java, we can directly work with databases using JDBC.
- JDBC – Java DataBase Connectivity is a Java API that allows Java code or programs to interact with the database.
- Scala code blocks or programs similarly use these JDBC APIs in Java programs.
- Using this JDBC API, we will establish Database connectivity using Scala.
To do the same, you need the jar file and for that, you can even locate the jar file in that directory where the file is located itself or provide the classpath while compiling.
For the above query, refer to this: JAR files in Java
Now let’s see simple database connectivity code using Scala.
Scala
import java.sql.{Connection, DriverManager, SQLException}
object DatabaseConnectivity extends App {
//JDBC url for connectivity
val jdbcUrl = "jdbc:mysql://localhost:3306/test"
val uname = "root"//MySQL username
val pwd = ""//MySQL password
val connection: Connection = null
// try block for connectivity and if error or
// exception occurs it will be catched by catch block
try{
//Load Driver Class
Class.forName("com.mysql.cj.jdbc.Driver")
// Establish connection using url,
// mysql username an password
connection = DriverManager.getConnection(jdbcUrl, uname, pwd)
println("Connection successful!!")
} catch{
case e : ClassNotFoundException =>
println("Error loading jdbc driver class!!")
e.printStackTrace()
case e: SQLException =>
println("Error Connecting to the database!!")
e.printStackTrace()
}
}
The above code is very simple and basic code for the Scala connectivity with the MySQL database using JDBC.
- Import all required or specific classes required for the connection and program execution. The line ‘import java.sql.{Connection, DriverManager, SQLException}’ imports the classes from java.sql package.
- Then we defined an object named ‘DatabaseConnectivity’ in which we wrote the entire code.
- Then we defined 4 immutable variables using val. Following are the variables. First variable ‘jdbcUrl’ for JDBC Url for connectivity. Here ‘test’ in the url is the name of the database. Variables ‘uname’ and ‘pwd’ are used to hold MySQl username and password for connectivity and the last variable ‘connection’ is of Connection type, this will hold the actual connectivity statement.
- Next we have try-catch block for executing block of code properly with exceptions handled in try-catch block.
- In try block, we have ‘Class.forName’ is used for loading the driver class. Next we have connectivity of the database assigned to this variable with the url, username and password. The connection is established using DriverManager.getConnection() method.
- Next, if the connection is successfully established, then println in try block is executed with output “Connection successfull”.
- If there was error in the connection then, the execution flow is carried out by catch block where it gives output as per the error or exception occurred.
- In first case returns the output when the JDBC driver class is not found. And the second case returns output when there was some SQL Exception. The ‘printStackTrace()’ prints a detailed information about the exception or error that occured during the execution.
Lets see the output of the code block:
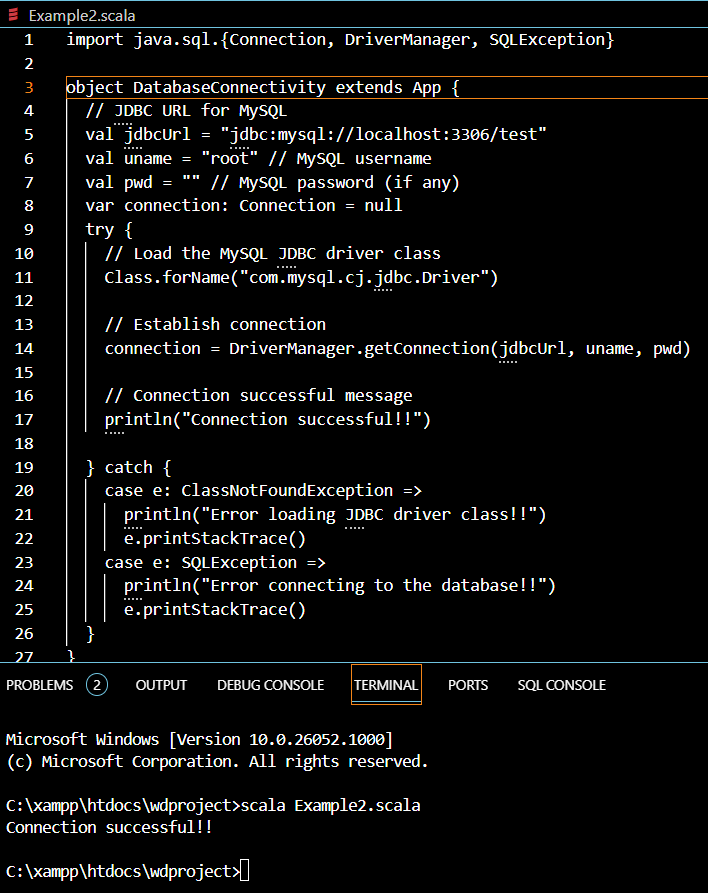
No error or Exception occurred during the execution and the connection was successful
For more details or to know more about the same you can refer to this database Connectivity with java for more clearance: Java Database Connectivity with MySQL
Share your thoughts in the comments
Please Login to comment...