How to Combine Multiple Node.js Web Applications ?
Last Updated :
04 Aug, 2023
As web applications become increasingly complex, it’s not uncommon for organizations to have multiple Node.js applications serving different purposes. However, managing and integrating these applications can present challenges. In this article, we will explore various approaches and best practices for combining multiple Node.js web applications effectively, ensuring seamless functionality and enhanced user experience.
Approach to Combine Multiple Node.js Web Applications
Create a new directory for the combined application and initialize a new Node.js project.
mkdir CombinedApp
cd CombinedApp
npm init -y
2. Install necessary dependencies
Install the required dependencies, including Express.js.
npm install express
3. Create the entry point for the combined application
In the root of the CombinedApp directory, create a new file named index.js
.
Javascript
const express = require( 'express' );
const app = express();
const app1 = require( './App1' );
app.use( '/app1' , app1);
const app2 = require( './App2' );
app.use( '/app2' , app2);
const port = 3000;
app.listen(port, () => {
console.log(`CombinedApp is listening on port ${port}`);
});
|
4. Create the directories and entry point files for each application
Create separate directories for App1 and App2 within the CombinedApp directory. Inside each directory, create a index.js
file.
For example, in the App1 directory, create a new file named index.js
:
Javascript
const express = require( 'express' );
const app = express();
app.get( '/' , (req, res) => {
res.send( 'Hello Geeks for Geeks from App1!' );
});
module.exports = app;
|
Similarly, create a similar index.js
file for App2:
Javascript
const express = require( 'express' );
const app = express();
app.get( '/' , (req, res) => {
res.send( 'Hello Geeks for Geeks from App2!' );
});
module.exports = app;
|
5. Run the combined application
Start the combined application by executing the following command in the terminal:
node index.js
6. Access the combined application
The combined application will be accessible at http://localhost:3000/app1
and http://localhost:3000/app2
. Each application will respond with their respective messages.
Output:
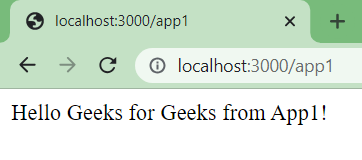
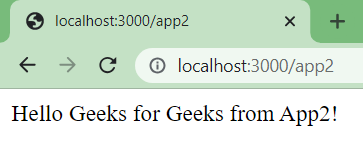
This output demonstrates accessing the combined application at http://localhost:3000/app1 and http://localhost:3000/app2, where each application responds with its respective messages.
Please note that the code provided is a simplified example, and in a real-world scenario, you may have more complex routes, middleware, and shared resources to handle. The key concept is to mount each application as middleware within the combined application, using different base URL paths to distinguish between them.
Share your thoughts in the comments
Please Login to comment...