How to Check Datatype in Scala?
Last Updated :
07 May, 2024
In this article, we will learn to check data types in Scala. Data types in Scala represent the type of values that variables can hold, aiding in type safety and program correctness.
Checking Datatype in Scala
Below are the possible approaches to check datatype in Scala.
Approach 1: Use Pattern Matching in Scala
- In this approach, we are using Pattern Matching in Scala to dynamically check the type of an object and return a corresponding string representing the detected data type.
- The approach1Fn method matches the object against different patterns (String, Int, List[_]), providing a flexible way to handle different data types in a concise and readable manner.
In the below example, Pattern Matching is used to check the datatype in Scala.
Scala
object GFG {
def main(args: Array[String]): Unit = {
val str: Any = "Hello"
val num: Any = 42
val lst: Any = List(1, 2, 3)
println(approach1Fn(str))
println(approach1Fn(num))
println(approach1Fn(lst))
}
def approach1Fn(obj: Any): String = obj match {
case _: String => "String"
case _: Int => "Int"
case _: List[_] => "List[Int]"
case _ => "Unknown"
}
}
Output:
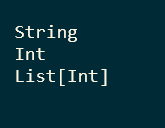
Approach 2: Use the getClass Method in Scala
- In this approach, we use the getClass method in Scala to retrieve the runtime class of an object as a string.
- The approach2Fn method takes any object (Any) as input and returns the string representation of its runtime class.
In the below example, the getClass Method is used to check the datatype in Scala.
Scala
object GFG {
def main(args: Array[String]): Unit = {
val str: Any = "Hello"
val num: Any = 42
val lst: Any = List(1, 2, 3)
println(approach2Fn(str))
println(approach2Fn(num))
println(approach2Fn(lst))
}
def approach2Fn(obj: Any): String = obj.getClass.toString
}
Output:
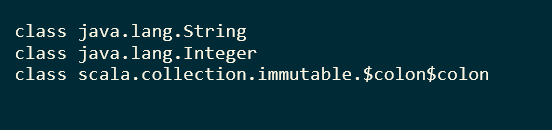
Approach 3: Use Manifest in Scala
- In this approach, we are using Manifest in Scala to obtain type information at runtime.
- The approach3Fn method takes a type parameter T with a Manifest context-bounded.
- It uses manifest[T].toString to get the string representation of the type T.
In the below example, Manifest is used to check the datatype in Scala.
Scala
object GFG {
def main(args: Array[String]): Unit = {
val str: Any = "Hello"
val num: Any = 42
val lst: Any = List(1, 2, 3)
println(approach3Fn[Int](str))
println(approach3Fn[Int](num))
println(approach3Fn[List[Int]](lst))
}
def approach3Fn[T: Manifest](obj: Any): String = manifest[T].toString
}
Output:
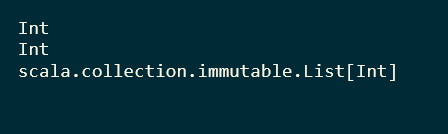
Approach 4: Use Type Checking (isInstanceOf) in Scala
- In this approach, we are using Type Checking (isInstanceOf) in Scala to determine the type of an object at runtime.
- The approach4Fn method takes an Any object as input and checks its type using isInstanceOf.
- It returns a string representing the detected data type (“String”, “Int”, “List[Int]”, or “Unknown”).
In the below example, Type Checking (isInstanceOf) is used to check the datatype in Scala.
Scala
object GFG {
def main(args: Array[String]): Unit = {
val str: Any = "Hello"
val num: Any = 42
val lst: Any = List(1, 2, 3)
println(approach4Fn(str))
println(approach4Fn(num))
println(approach4Fn(lst))
}
def approach4Fn(obj: Any): String = {
if (obj.isInstanceOf[String]) "String"
else if (obj.isInstanceOf[Int]) "Int"
else if (obj.isInstanceOf[List[_]]) "List[Int]"
else "Unknown"
}
}
Output:
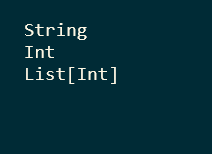
Share your thoughts in the comments
Please Login to comment...