How to Change SVG Icon Color on Click in JavaScript ?
Last Updated :
08 Feb, 2024
SVG stands for Scalable Vector Graphics and is a vector image format for two-dimensional images that may be used to generate animations. The XML format defines the SVG document. You can change the color of an SVG using the below methods:
Using the setAttribute()
The setAttribute() method is used to alter the SVG element’s fill attribute to dynamically change its color by passing the value for this attribute as the second parameter to this method.
Syntax:
element.setAttribute('attributeName', 'value');
Example: The below code implements the setAttribute() method to change color of SVG in JavaScript.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Change SVG color</ title >
< style >
h2 {
color: green;
}
#element{
text-align: center;
}
</ style >
</ head >
< body style = "text-align: center;" >
< h2 >GeeksforGeeks</ h2 >
< svg id = 'element' >
< rect id = 'rectangle' width = '100%'
height = '100%' fill = 'green' />
</ svg >< br />< br />
< button id = "btn" >Change Color</ button >
< script >
let rect =
document.getElementById('rectangle')
let btn =
document.getElementById('btn')
btn.addEventListener('click', () => {
rect.setAttribute('fill', 'red')
})
</ script >
</ body >
</ html >
|
Output:
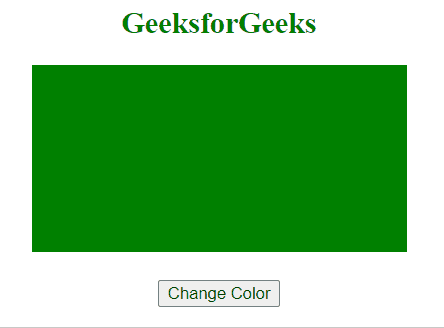
Using style.fill property
In this approach, the color of the SVG element can be changed by using style.fill property in JavaScript by assigning it a new color value using the assignment operator.
Syntax:
element.style.fill = 'value';
Example: The below code uses the style.fill property to change color of the SVG onclick in JavaScript.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Change SVG color</ title >
< style >
h2 {
color: green;
}
#element {
text-align: center;
}
</ style >
</ head >
< body style = "text-align: center;" >
< h2 >GeeksforGeeks</ h2 >
< svg id = 'element' >
< circle id = 'circle' cx = '50%' cy = '50%'
r = '30%' fill = 'green' />
</ svg >< br />< br />
< button id = "btn" >Change Color</ button >
< script >
let circle =
document.getElementById('circle')
let btn =
document.getElementById('btn')
btn.addEventListener('click', () => {
circle.style.fill = 'red'
})
</ script >
</ body >
</ html >
|
Output:
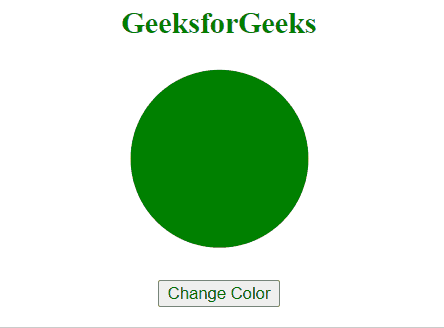
Share your thoughts in the comments
Please Login to comment...