How to catch all JavaScript errors and send them to server ?
Last Updated :
15 Feb, 2023
Today there are a large number of Web APIs available. One of which is GlobalEventHandlers, an OnErrorEventHandler to be called when the error is raised.
The onerror property of the GlobalEventHandlers is an EventHandler that processes error events. This is great for catching exceptions that never occur while testing. The different error events are :
- When a JavaScript runtime error occurs, window.onerror() is invoked.
- When a resource fails to load, the onerror() handler on the element is invoked. These error events can be handled with a window.addEventListener configured with useCapture set to True.
All JavaScript errors can be caught by this browser event onerror. It is one of the easiest ways to log client-side errors and report them to your servers. A function is assigned to the window.onerror as such:
Syntax:
window.onerror = function (msg, source, lineNo, columnNo, error) {
// function to execute error handling
}
The following arguments are passed to the function that’s assigned to the window.onerror:
- msg : The message related to the error.
- source : The URL of the script or the document related to the error.
- lineNo: The line number.
- columnNo: The column number.
- error: The Error object related with this error.
If the return value is true, then only the error is handled.
Example:
Catching error: By using the below code we can catch all the errors.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
Catch all JavaScript errors
and send them to server
</ title >
</ head >
< body >
< center >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< b >
Catch all JavaScript errors
and send them to server
</ b >
< a href = "javascript:myalert()" >click here</ a >
< script >
window.onerror =
function (msg, source, lineNo, columnNo, error) {
alert("Error: " + msg +
"\nScript: " + source +
"\nLine: " + lineNo +
"\nColumn: " + columnNo +
"\nStackTrace: " + error);
return true;
};
var myalert = function () {
alert(gfg);
};
</ script >
</ center >
</ body >
</ html >
|
Output :
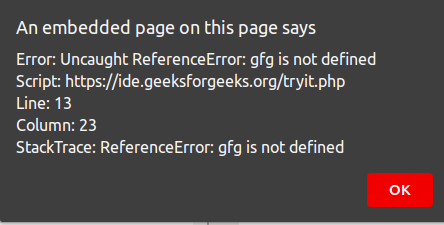
Errors sending on server: After you’ve plugged into window.onerror in order to catch as much error information as possible,there’s just one last step, i.e. transmitting the error information to your servers. In the below example below, we use jQuery’s AJAX function to transmit the data to the servers:
Javascript
function captureError(err) {
var errorData = {
name: err.name,
message: err.line,
url: document.location.href,
stack: err.stack
};
$.post( '/logger/js/' , {
data: errorData
});
}
|
Share your thoughts in the comments
Please Login to comment...