How to Build a Search Filter using React Hooks ?
Last Updated :
27 Feb, 2024
In modern web development, implementing a search feature is a common requirement for various applications. With React Hooks, developers can efficiently manage state and lifecycle events, making it an ideal choice for building interactive user interfaces, including search functionalities. In this article, we’ll explore how to implement a search feature using React Hooks.
We will discuss the following two approaches for implementing Search Filter with React Hooks
useState Hook:
React useState Hook allows to store and access of the state in the functional components. State refers to data or properties that need to be stored in an application.
Syntax of useState Hook:
const [state, setState] = useState(initialState);
useEffect Hook:
React useEffect hook handles the effects of the dependency array. It is called every time any state if the dependency array is modified or updated.
Syntax of useEffect Hook:
useEffect(() => {
// Effect code here
return () => {
// Cleanup code here (optional)
};
}, [dependencies]);
Approach to Implement Search Feature with React Hooks:
We will utilize the useState hook to manage the search query state and useEffect hook to trigger the search functionality whenever the search query changes. The search functionality will filter through a list of items based on the search query.
Basic Search Implementation:
This example demonstrates a simple search feature where users can input a search query, and the application filters a list of items based on that query.
Example: Below is an example of implementing a basic search feature with React Hooks.
Javascript
import React, {
useState,
useEffect
} from 'react' ;
function SearchFeature() {
const [query, setQuery] = useState( '' );
const [filteredItems, setFilteredItems] = useState([]);
const items = [ 'apple' , 'banana' , 'orange' ,
'grape' , 'watermelon' ];
useEffect(() => {
const filtered = items.filter(item =>
item.toLowerCase().includes(query.toLowerCase())
);
setFilteredItems(filtered);
}, [query]);
return (
<div>
<input
type= "text"
placeholder= "Search..."
value={query}
onChange={(e) => setQuery(e.target.value)}
/>
<ul>
{filteredItems.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
</div>
);
}
export default SearchFeature;
|
Output:
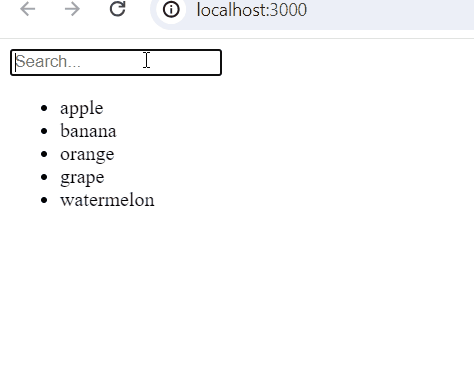
Ouput
Explanation of Output:
- We declare state variables query and filteredItems using the useState hook.
- Inside the useEffect hook, we filter the items array based on the current value of the query state.
- The filtered items are updated whenever the query state changes.
- The input element allows users to input their search query, which updates the query state accordingly.
- The filtered items are displayed as a list below the search input.
Search Feature with API Integration:
In this example, we enhance the search functionality by fetching user data from the Random User API and filtering the results based on the user’s search query.
Example: Below is an example to implementing a search feature with API integration and React Hooks:
Javascript
import React, {
useState,
useEffect
} from 'react' ;
function SearchFeatureWithRandomUserAPI() {
const [query, setQuery] = useState( '' );
const [results, setResults] = useState([]);
useEffect(() => {
async function fetchData() {
try {
const response = await
fetch(`https:
const data = await response.json();
setResults(data.results);
} catch (error) {
console.error( 'Error fetching data:' , error);
}
}
fetchData();
}, []);
const filteredResults = results.filter(user =>
user.name.first.toLowerCase().includes(query.toLowerCase()) ||
user.name.last.toLowerCase().includes(query.toLowerCase())
);
return (
<div>
<input
type= "text"
placeholder= "Search..."
value={query}
onChange={(e) => setQuery(e.target.value)}
/>
<ul>
{filteredResults.map((user, index) => (
<li key={index}>
{user.name.first} {user.name.last}
</li>
))}
</ul>
</div>
);
}
export default SearchFeatureWithRandomUserAPI;
|
Output:
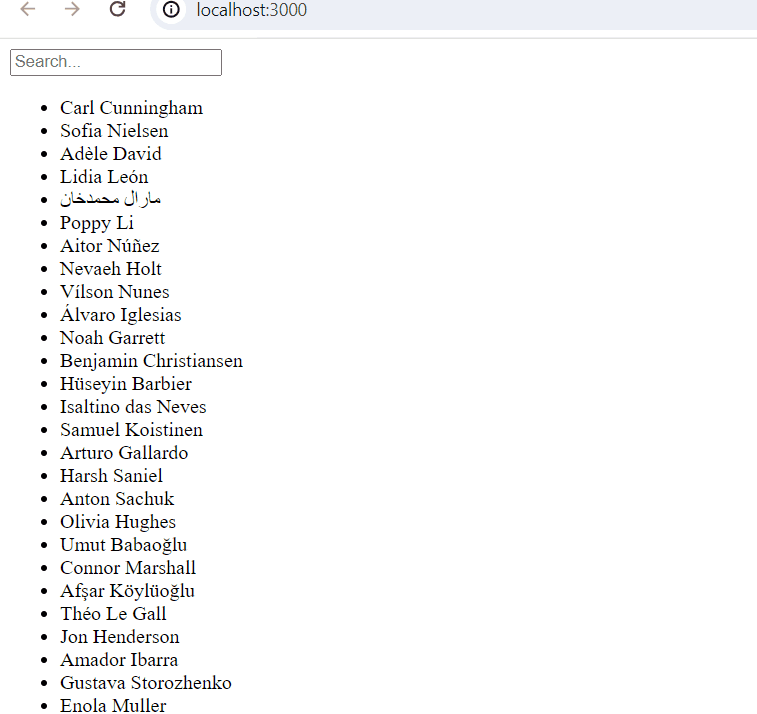
Output
Explanation of Output:
- We declare state variables query and results using the useState hook. query stores the search query entered by the user, and results stores the fetched user data.
- Inside the useEffect hook, we use the fetch API to make an asynchronous call to the Random User API endpoint. We specify the number of results we want to fetch (100 in this case). The fetched data is then stored in the results state.
- The useEffect hook is set to run once when the component mounts, as indicated by the empty dependency array [ ].
- As the user types in the search input, the query state is updated accordingly. We filter the results array based on the search query using the filter method.
- The filtered user data is displayed as a list below the search input, showing the first and last names of the users that match the search query.
Share your thoughts in the comments
Please Login to comment...