How to Build a Password Manager in Python
Last Updated :
18 Mar, 2024
We have a task to create a Password Manager in Python. In this article, we will see how to create a Password Manager in Python.
What is a Password Manager?
A Password Manager in Python is a tool in which we can add the username and password. Additionally, it allows us to retrieve the username and password. We also have the option to view all added usernames and passwords with just one click. Furthermore, if we want to delete a username and password, we can do so with a single click.
Password Manager in Python
Below is the step-by-step guide for creating a Password Manager in Python:
Create a Virtual Environment
Now, create the virtual environment using the below commands
python -m venv env
.\env\Scripts\activate.ps1
Installation
Here, we will install the following libraries and modules:
- Install Python
- Install Tkinter
Writing the Tkinter Code (app.py)
Below are the step-by-step explanation of the app.py code:
Step 1: User Input and Password Addition
In below code add()
function captures the username and password entered by the user in the Tkinter GUI. It checks if both fields are non-empty. If so, it appends the username and password to a file named “passwords.txt” and displays a success message using a Tkinter messagebox.
Python3
import tkinter as tk
from tkinter import messagebox
def add():
# accepting input from the user
username = entryName.get()
# accepting password input from the user
password = entryPassword.get()
if username and password:
with open("passwords.txt", 'a') as f:
f.write(f"{username} {password}\n")
messagebox.showinfo("Success", "Password added !!")
else:
messagebox.showerror("Error", "Please enter both the fields")
Step 2: Retrieve and Display Password
In below code get()
function retrieves a username entered by the user and reads a file named “passwords.txt” to create a dictionary of username-password pairs. If the entered username exists, it displays the corresponding password; otherwise, it indicates that the username doesn’t exist.
Python3
def get():
# accepting input from the user
username = entryName.get()
# creating a dictionary to store the data in the form of key-value pairs
passwords = {}
try:
# opening the text file
with open("passwords.txt", 'r') as f:
for k in f:
i = k.split(' ')
# creating the key-value pair of username and password.
passwords[i[0]] = i[1]
except:
# displaying the error message
print("ERROR !!")
if passwords:
mess = "Your passwords:\n"
for i in passwords:
if i == username:
mess += f"Password for {username} is {passwords[i]}\n"
break
else:
mess += "No Such Username Exists !!"
messagebox.showinfo("Passwords", mess)
else:
messagebox.showinfo("Passwords", "EMPTY LIST!!")
Step 3: Retrieve and Display All Passwords
In below code the getlist()
function reads the “passwords.txt” file, creates a dictionary of all username-password pairs, and displays a messagebox containing the entire list of passwords. If the file is empty, it notifies the user that the list is empty.
Python3
def getlist():
# creating a dictionary
passwords = {}
# adding a try block, this will catch errors such as an empty file or others
try:
with open("passwords.txt", 'r') as f:
for k in f:
i = k.split(' ')
passwords[i[0]] = i[1]
except:
print("No passwords found!!")
if passwords:
mess = "List of passwords:\n"
for name, password in passwords.items():
# generating a proper message
mess += f"Password for {name} is {password}\n"
# Showing the message
messagebox.showinfo("Passwords", mess)
else:
messagebox.showinfo("Passwords", "Empty List !!")
Step 4: Delete User and Update File
In below code the delete()
function takes a username input from the user, reads the “passwords.txt” file, excludes the specified username, and writes the modified data back to the file. If the deletion is successful, it displays a success message; otherwise, it shows an error message.
Python3
def delete():
# accepting input from the user
username = entryName.get()
# creating a temporary list to store the data
temp_passwords = []
# reading data from the file and excluding the specified username
try:
with open("passwords.txt", 'r') as f:
for k in f:
i = k.split(' ')
if i[0] != username:
temp_passwords.append(f"{i[0]} {i[1]}")
# writing the modified data back to the file
with open("passwords.txt", 'w') as f:
for line in temp_passwords:
f.write(line)
messagebox.showinfo(
"Success", f"User {username} deleted successfully!")
except Exception as e:
messagebox.showerror("Error", f"Error deleting user {username}: {e}")
Step 5: Tkinter GUI Setup
below code has main part of the code initializes a Tkinter GUI window, sets its dimensions and title, and creates input fields for username and password. Four buttons are provided for adding passwords (Add
), retrieving a password (Get
), listing all passwords (List
), and deleting a user (Delete
).
Python3
if __name__ == "__main__":
app = tk.Tk()
app.geometry("560x270")
app.title("GeeksForGeeks Password Manager")
# Username block
labelName = tk.Label(app, text="USERNAME:")
labelName.grid(row=0, column=0, padx=15, pady=15)
entryName = tk.Entry(app)
entryName.grid(row=0, column=1, padx=15, pady=15)
# Password block
labelPassword = tk.Label(app, text="PASSWORD:")
labelPassword.grid(row=1, column=0, padx=10, pady=5)
entryPassword = tk.Entry(app)
entryPassword.grid(row=1, column=1, padx=10, pady=5)
# Add button
buttonAdd = tk.Button(app, text="Add", command=add)
buttonAdd.grid(row=2, column=0, padx=15, pady=8, sticky="we")
# Get button
buttonGet = tk.Button(app, text="Get", command=get)
buttonGet.grid(row=2, column=1, padx=15, pady=8, sticky="we")
# List Button
buttonList = tk.Button(app, text="List", command=getlist)
buttonList.grid(row=3, column=0, padx=15, pady=8, sticky="we")
# Delete button
buttonDelete = tk.Button(app, text="Delete", command=delete)
buttonDelete.grid(row=3, column=1, padx=15, pady=8, sticky="we")
app.mainloop()
Complete Code
Below is the complete code for main.py file that we have used in our project.
main.py
Python3
import tkinter as tk
from tkinter import messagebox
def add():
# accepting input from the user
username = entryName.get()
# accepting password input from the user
password = entryPassword.get()
if username and password:
with open("passwords.txt", 'a') as f:
f.write(f"{username} {password}\n")
messagebox.showinfo("Success", "Password added !!")
else:
messagebox.showerror("Error", "Please enter both the fields")
def get():
# accepting input from the user
username = entryName.get()
# creating a dictionary to store the data in the form of key-value pairs
passwords = {}
try:
# opening the text file
with open("passwords.txt", 'r') as f:
for k in f:
i = k.split(' ')
# creating the key-value pair of username and password.
passwords[i[0]] = i[1]
except:
# displaying the error message
print("ERROR !!")
if passwords:
mess = "Your passwords:\n"
for i in passwords:
if i == username:
mess += f"Password for {username} is {passwords[i]}\n"
break
else:
mess += "No Such Username Exists !!"
messagebox.showinfo("Passwords", mess)
else:
messagebox.showinfo("Passwords", "EMPTY LIST!!")
def getlist():
# creating a dictionary
passwords = {}
# adding a try block, this will catch errors such as an empty file or others
try:
with open("passwords.txt", 'r') as f:
for k in f:
i = k.split(' ')
passwords[i[0]] = i[1]
except:
print("No passwords found!!")
if passwords:
mess = "List of passwords:\n"
for name, password in passwords.items():
# generating a proper message
mess += f"Password for {name} is {password}\n"
# Showing the message
messagebox.showinfo("Passwords", mess)
else:
messagebox.showinfo("Passwords", "Empty List !!")
def delete():
# accepting input from the user
username = entryName.get()
# creating a temporary list to store the data
temp_passwords = []
# reading data from the file and excluding the specified username
try:
with open("passwords.txt", 'r') as f:
for k in f:
i = k.split(' ')
if i[0] != username:
temp_passwords.append(f"{i[0]} {i[1]}")
# writing the modified data back to the file
with open("passwords.txt", 'w') as f:
for line in temp_passwords:
f.write(line)
messagebox.showinfo(
"Success", f"User {username} deleted successfully!")
except Exception as e:
messagebox.showerror("Error", f"Error deleting user {username}: {e}")
if __name__ == "__main__":
app = tk.Tk()
app.geometry("560x270")
app.title("GeeksForGeeks Password Manager")
# Username block
labelName = tk.Label(app, text="USERNAME:")
labelName.grid(row=0, column=0, padx=15, pady=15)
entryName = tk.Entry(app)
entryName.grid(row=0, column=1, padx=15, pady=15)
# Password block
labelPassword = tk.Label(app, text="PASSWORD:")
labelPassword.grid(row=1, column=0, padx=10, pady=5)
entryPassword = tk.Entry(app)
entryPassword.grid(row=1, column=1, padx=10, pady=5)
# Add button
buttonAdd = tk.Button(app, text="Add", command=add)
buttonAdd.grid(row=2, column=0, padx=15, pady=8, sticky="we")
# Get button
buttonGet = tk.Button(app, text="Get", command=get)
buttonGet.grid(row=2, column=1, padx=15, pady=8, sticky="we")
# List Button
buttonList = tk.Button(app, text="List", command=getlist)
buttonList.grid(row=3, column=0, padx=15, pady=8, sticky="we")
# Delete button
buttonDelete = tk.Button(app, text="Delete", command=delete)
buttonDelete.grid(row=3, column=1, padx=15, pady=8, sticky="we")
app.mainloop()
Output
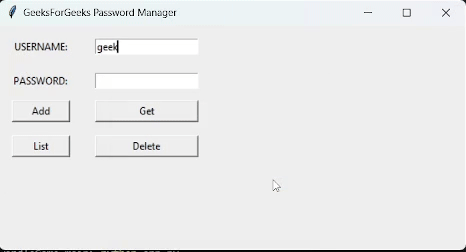
Password Manager In Python
Share your thoughts in the comments
Please Login to comment...