How to Append Data in Excel Using Python
Last Updated :
04 Mar, 2024
We are given an Excel file and our task is to append the data into this excel file using Python. In this article, we’ll explore different approaches to append data to an Excel file using Python.
Append Data in Excel Using Python
Below, are some examples to understand how to append data in excel using Python:
excel1.xlsx: Let’s say we have an existing Excel file named ‘excel1.xlsx’ with the following data:
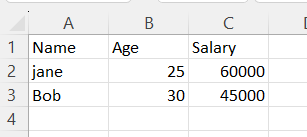
excel1.xlsx
Example 1: Append Data In Excel Using Pandas Library
Before getting started, ensure that the pandas
library is installed. If not, you can install it using:
pip install pandas
In this example, below Python code uses the Pandas library to append new data (e.g., {‘Name’: [‘Bob’], ‘Age’: [28], ‘Salary’: [55000]}) to an existing Excel file (‘excel1.xlsx’). It reads the existing data into a DataFrame, appends the new data, and then saves the combined data back to the original Excel file.
Python3
import pandas as pd
existing_file = 'excel1.xlsx'
new_data = { 'Name' : [ 'Bob' ], 'Age' : [ 28 ], 'Salary' : [ 55000 ]}
df_new = pd.DataFrame(new_data)
df_existing = pd.read_excel(existing_file)
df_combined = df_existing.append(df_new, ignore_index = True )
df_combined.to_excel(existing_file, index = False )
|
Output:
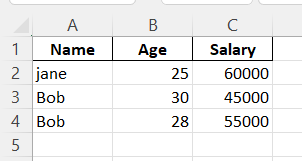
updated
Example 2: Append Data In Excel Using Openpyxl Library
If you don’t have the openpyxl
library installed, you can install it using
pip install openpyxl
In this example, below Python code utilizes the openpyxl
library to load an existing Excel file (‘existing_data.xlsx’), appends new data (e.g., [‘Bob’, 28, 55000]) to its active sheet, and saves the modified workbook back to the original file.
Python3
from openpyxl import load_workbook
existing_file = 'excel1.xlsx'
new_data = [[ 'Bob' , 28 , 55000 ]]
wb = load_workbook(existing_file)
ws = wb.active
for row in new_data:
ws.append(row)
wb.save(existing_file)
|
Output:
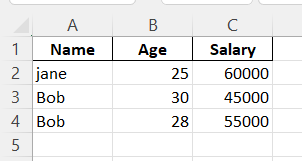
updated
Conclusion
In conlcusion, Appending data to an existing Excel file using Python is a straightforward process with the help of libraries like pandas
and openpyxl
. Whether you prefer the convenience of pandas
for its DataFrame operations or the direct manipulation capabilities of openpyxl
, Python provides versatile tools for efficiently managing and updating Excel data. Choose the approach that best fits your workflow and data manipulation needs.
Share your thoughts in the comments
Please Login to comment...