How to access Ethereum data using Etherscan.io API?
Last Updated :
24 Aug, 2023
The easiest way to get started with Ethereum is by using an Application Programming Interface. Etherscan API is one of the famous blockchain explorers and a great decentralized smart contracts platform. The developers can directly access ether scan’s block explorer through the Etherscan Developer APIs and easily make GET/POST requests. In this article, we will see multiple examples of Etherscan API with Python.
Required Modules
pip install etherscan-python
Steps To Create an Account And Get API -KEY
Register an account
To access the API, the first step would be to navigate to their website and create an account. By signing up on their website, you can access various services provided by Etherscan API. Click on the below link to register an account:
https://etherscan.io/register
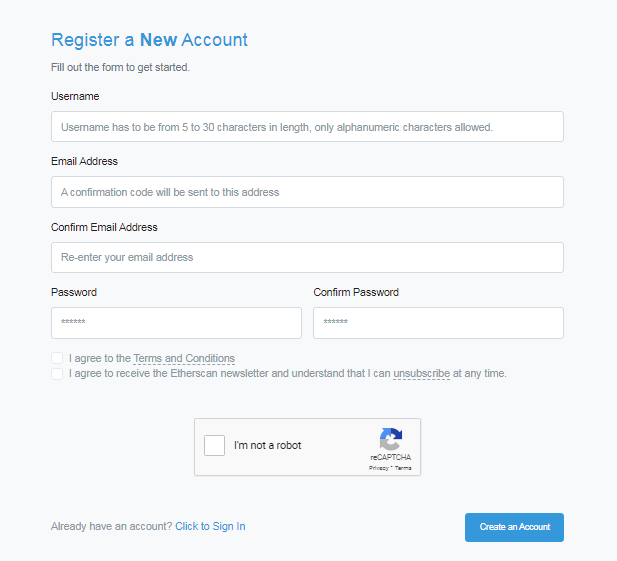
Account Create
You will receive a confirmation email after registration is done. Open the email account and complete the verification process. Next, log in to your account.
You will then be directed to the Etherscan dashboard as shown below.
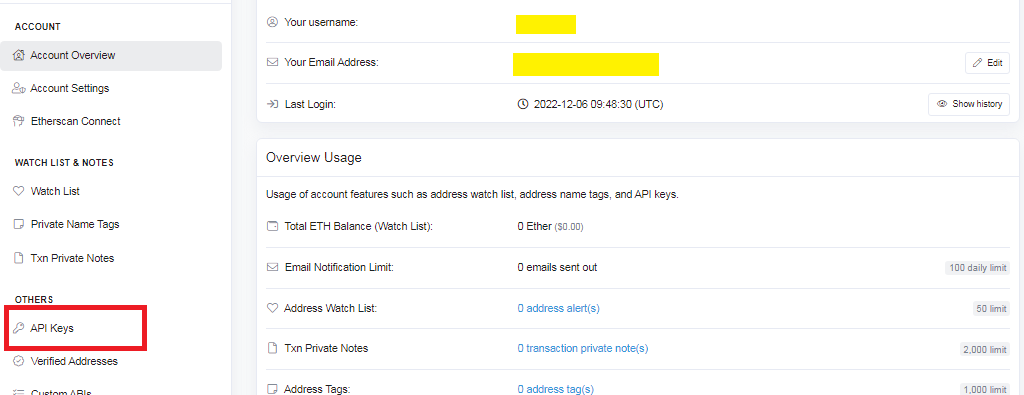
ether scan api keys
Generate an API key
On the left-hand side, you will find the menu with various items. Click on the option API Keys as highlighted in the above image. Next, click on the blue button on the right-hand side named ‘+Add’ and enter the name of the API Key. Save your API Key somewhere as it will be used in making get/post requests.
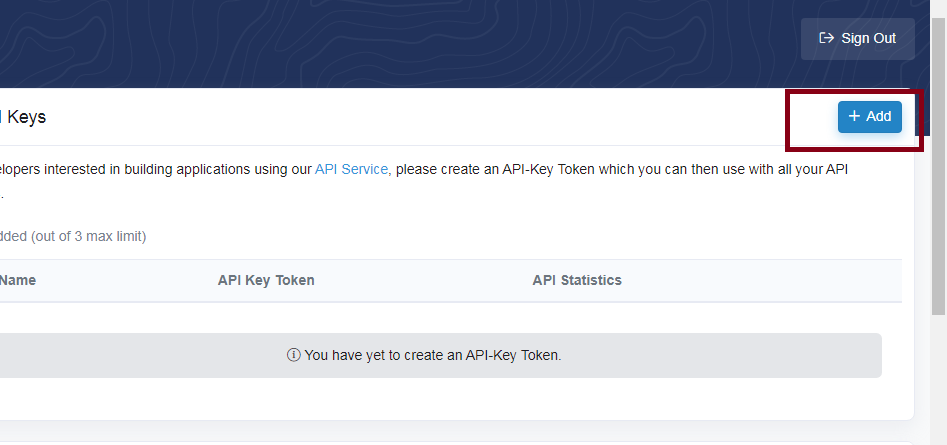
Generate api token
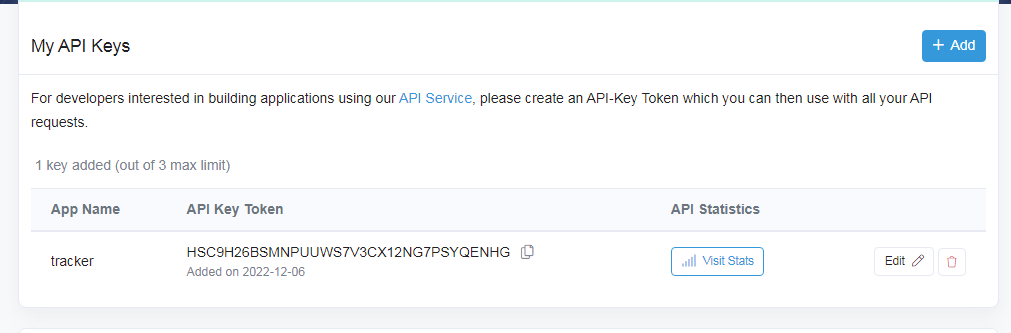
MY api keys
Choose an endpoint
You can refer to the documentation https://docs.etherscan.io/ to have a look at various endpoints and their corresponding URLs.
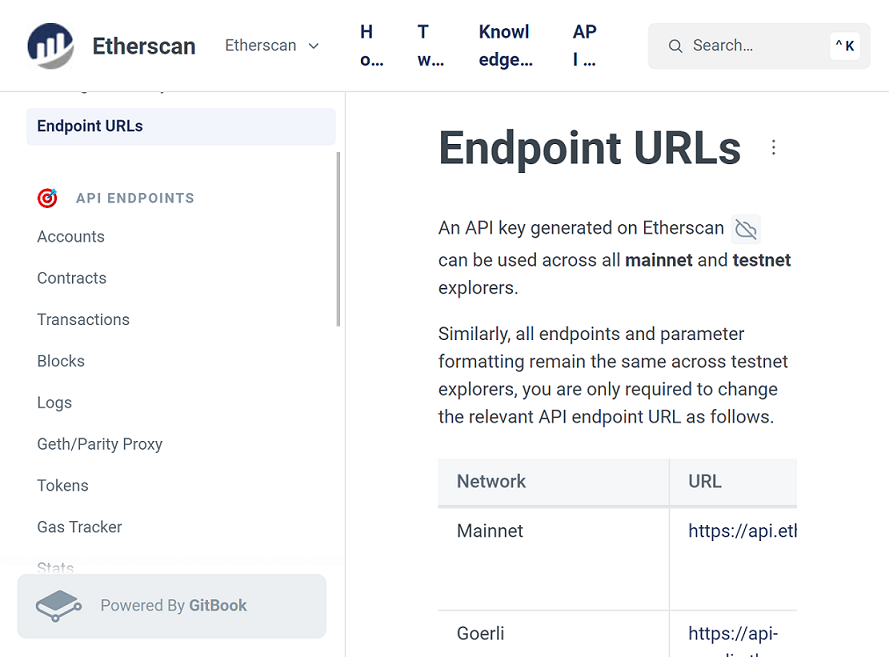
URL Endpoint
Gas Oracle
If we click on the Gas Tracker item from the menu we will be directed to various URLs in the gas category. In this tutorial, I will be using the Gas Oracle URL.
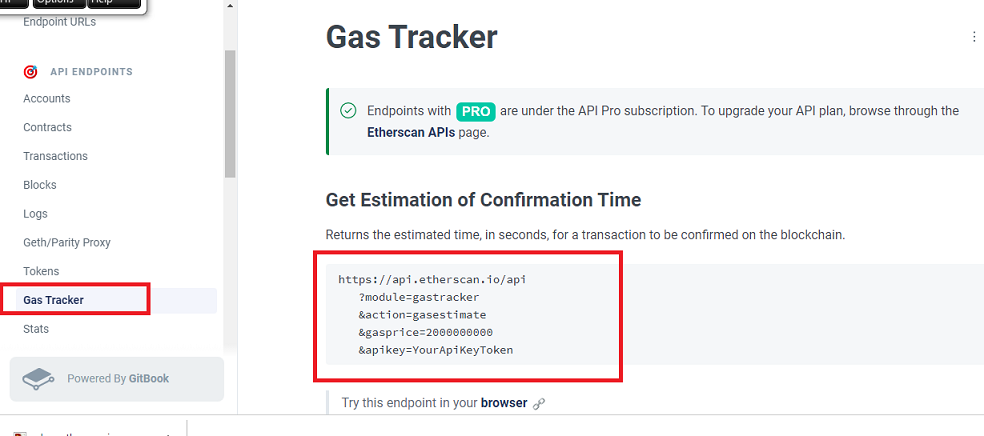
Gas Tracker
Examples of Etherscan API With Python
Get Eth_balance
Python3
addr = "0x39eB410144784010b84B076087B073889411F878"
eth_balance = float (Etherscan.get_eth_balance(addr))
print ( float (eth_balance / 1000000000000000000 ))
|
Output
0.0085417132
Get Gas Oracle
Endpoint - https://api.etherscan.io/api?module=gastracker&action=gasoracle&apikey=YourApiKeyToken
Python3
import requests as re
API_KEY = "---your API key--- "
"api?module=gastracker&" +
"action=gasoracle&" +
"apikey=HSC9H26BSMNPUUWS7V3CX12NG7PSYQENHG" )
prices = res_gas.json().get( "result" )
print (prices)
print ( "SafeGasPrice : " + prices[ "SafeGasPrice" ])
print ( "ProposeGasPrice : " + prices[ "ProposeGasPrice" ])
print ( "FastGasPrice : " + prices[ "FastGasPrice" ])
|
Output
{'LastBlock': '16218002', 'SafeGasPrice': '12', 'ProposeGasPrice': '13', 'FastGasPrice': '15', 'suggestBaseFee': '11.893
709176', 'gasUsedRatio': '0.382667833333333,0.8360639,0.374166233333333,0.244114066666667,0.999445333333333'}
SafeGasPrice: 12
ProposeGasPrice: 13
FastGasPrice: 15
Get Ether Balance for Multiple Addresses in a Single Call
Endpoint - https://api.etherscan.io/api
?module=account
&action=balancemulti
&address=0xddbd2b932c763ba5b1b7ae3b362eac3e8d40121a,0x63a9975ba31b0b9626b34300f7f627147df1f526,0x198ef1ec325a96cc354c7266a038be8b5c558f67
&tag=latest
&apikey=YourApiKeyToken
Python3
import requests as re
API_KEY = ""
"module=account&action=balancemulti" +
"&address=0xddbd2b932c763ba5b1b7ae3b362eac3e8d40121a," +
"0x63a9975ba31b0b9626b34300f7f627147df1f526,"
+ "0x198ef1ec325a96cc354c7266a038be8b5c558f67&" +
"tag=latest&apikey=HSC9H26BSMNPUUWS7V3CX12NG7PSYQENHG&" +
"endblock=2702578&page=1offset=10&sort=asc&" +
"apikey=HSC9H26BSMNPUUWS7V3CX12NG7PSYQENHG" )
d = res3.json().get( "result" )
for i in d:
print ( "Account : " + i[ "account" ] + " Balance : " + i[ "balance" ])
|
Output
Account: 0xddbd2b932c763ba5b1b7ae3b362eac3e8d40121a Balance: 40891626854930000000999
Account: 0x63a9975ba31b0b9626b34300f7f627147df1f526 Balance: 332567136222827062478
Account: 0x198ef1ec325a96cc354c7266a038be8b5c558f67 Balance: 21000000000000000
Get Ether Last Price
Endpoint - https://api.etherscan.io/api?module=stats&action=ethprice&apikey=YourApiKeyToken
Python3
import requests as re
API_KEY = ""
+ "module=stats&" +
"action=ethprice&" +
"apikey=HSC9H26BSMNPUUWS7V3CX12NG7PSYQENHG" )
d = res3.json().get( "result" )
print (d)
|
Output
{'ethbtc': '0.06539', 'ethbtc_timestamp': '1683193882', 'ethusd': '1902.65', 'ethusd_timestamp': '1683193881'}
Token Transfer Events by Address
API Endpoint - https://api.etherscan.io/api
?module=account
&action=tokentx
&contractaddress=0x9f8f72aa9304c8b593d555f12ef6589cc3a579a2
&address=0x4e83362442b8d1bec281594cea3050c8eb01311c
&page=1
&offset=100
&startblock=0
&endblock=27025780
&sort=asc
&apikey=YourApiKeyToken
Python3
import requests as re
API_KEY = ""
"account&action=tokentx&contractaddress=" +
"0x9f8f72aa9304c8b593d555f12ef6589cc3a579a2" +
"&address=0x4e83362442b8d1bec281594cea3050c8eb01311c" +
"&page=1" +
"&offset=100&startblock=0&endblock=27025780&" +
"sort=asc&" +
"apikey=HSC9H26BSMNPUUWS7V3CX12NG7PSYQENHG" )
d = res3.json().get( "result" )
for i in d:
print ( "Block Number : "
+ i[ "blockNumber" ]
+ " Time Stamp : "
+ i[ "timeStamp" ]
+ " hash : "
+ i[ "hash" ]
+ "nonce :"
+ i[ "nonce" ]
+
"contactAddress : "
+ i[ "hash" ]
+ "token name : "
+ i[ "tokenName" ])
|
Output
Block Number : 4730207 Time Stamp : 1513240363 hash : 0xe8c208398bd5ae8e4c237658580db56a2a94dfa0ca382c99b776fa6e7d31d5
b4nonce :406contactAddress : 0xe8c208398bd5ae8e4c237658580db56a2a94dfa0ca382c99b776fa6e7d31d5b4token name : Maker
Block Number : 4764973 Time Stamp : 1513764636 hash : 0x9c82e89b7f6a4405d11c361adb6d808d27bcd9db3b04b3fb3bc05d182bbc5d
6fnonce :428contactAddress : 0x9c82e89b7f6a4405d11c361adb6d808d27bcd9db3b04b3fb3bc05d182bbc5d6ftoken name : Maker
......
d1nonce :730contactAddress : 0x497f15095877bc06b9e0f422673c1e0f13a2b4224b615ef29ce8c46e249364d1token name : Maker
Block Number : 5986253 Time Stamp : 1531915309 hash : 0xad56a518a5052bf5293ab14d8fe03fc6f7dfb09c7aaa605bc89b2c3dc62acd
cdnonce :756contactAddress : 0xad56a518a5052bf5293ab14d8fe03fc6f7dfb09c7aaa605bc89b2c3dc62acdcdtoken name : Maker
Share your thoughts in the comments
Please Login to comment...