How Inner Join works in LINQ to SQL
Last Updated :
20 Feb, 2024
LINQ (Language Integrated Query) in C# provides a powerful way to query data from various data sources, including databases. In LINQ to SQL, which is specifically designed for working with relational databases, an inner join is a common operation used to combine records from two tables based on a specified condition.
This article explores the concept of an inner join in LINQ to SQL, presenting its syntax, illustrating its application with examples, and providing a comprehensive conclusion.
Inner Join in LINQ
In LINQ, an inner join combines records from two tables where there is a match based on a specified condition. The result set contains only the records that have matching values in both tables.
Syntax:
The syntax for an inner join in LINQ to SQL is expressed using the join and on keywords, specifying the join condition. The basic structure is as follows:
var query = from table1 in context.Table1
join table2 in context.Table2
on table1.CommonField equals table2.CommonField
select new
{
// Select the desired fields from both tables
};
Example of LINQ to SQL Inner Join Syntax
Example 1: Inner Join with Simple Fields
Consider two tables, Customers and Orders, where an inner join is performed based on the common field CustomerID.
Customers Table:
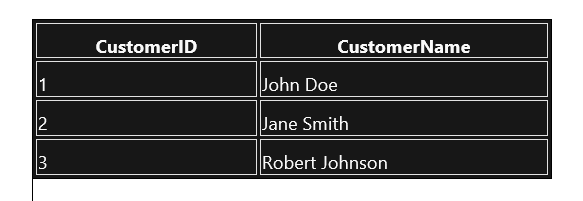
Customers Table
Orders Table:
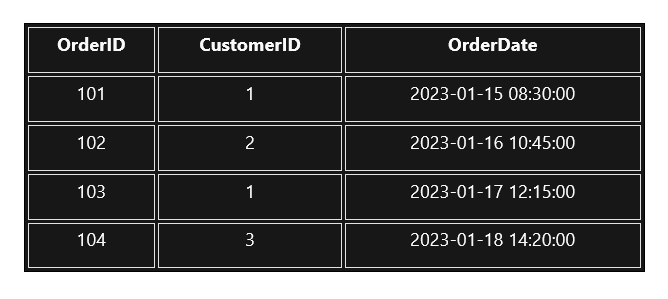
Orders Table
Query:
var innerJoinQuery = from customer in context.Customers
join order in context.Orders
on customer.CustomerID equals order.CustomerID
select new
{
customer.CustomerID,
customer.CustomerName,
order.OrderID,
order.OrderDate
};
Output:
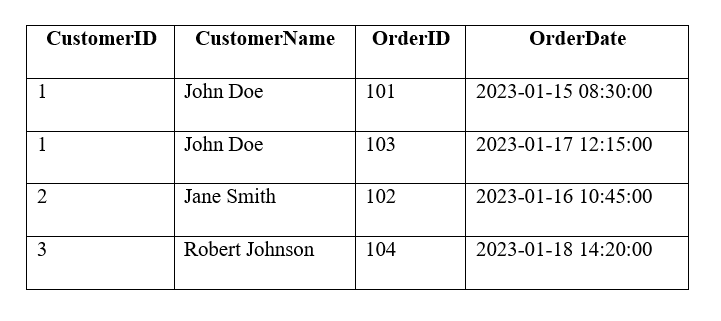
Output
Explanation: The innerJoinQuery combines data from the “Customers” and “Orders” tables, linking them based on the common CustomerID field. The output is an anonymous type with selected fields, including customer ID, customer name, order ID, and order date, providing a consolidated view of relevant information from both tables.
Example 2: Inner Join with Complex Condition
Consider two tables, Employees and Departments, where an inner join is performed based on a more complex condition involving multiple fields.
Employees Table:
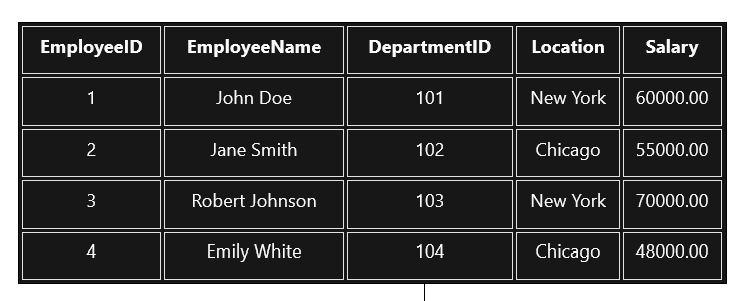
Employees Table
Departments Table:
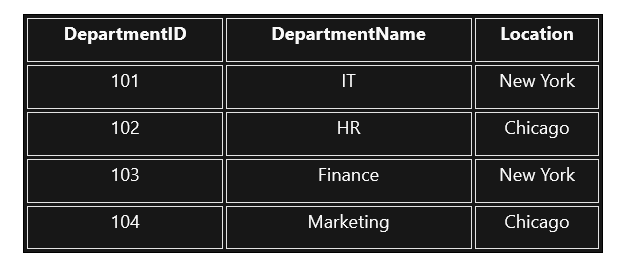
Departments Table
Query:
var complexJoinQuery = from employee in context.Employees
join department in context.Departments
on new { employee.DepartmentID, employee.Location }
equals new { department.DepartmentID, department.Location }
select new
{
employee.EmployeeID,
employee.EmployeeName,
department.DepartmentID,
department.DepartmentName
};
Output:
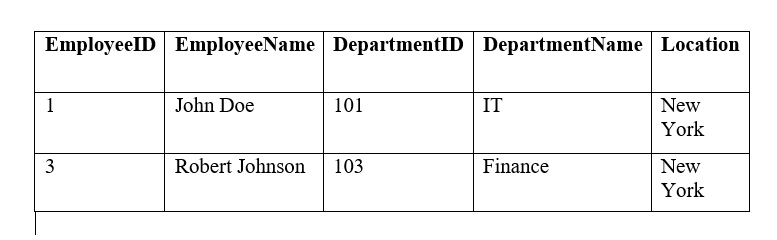
Output
Explanation: The complexJoinQuery in LINQ to SQL performs a join between “Employees” and “Departments” tables using a composite key, matching both DepartmentID and Location. The output comprises an anonymous type with selected fields, including employee ID, employee name, department ID, and department name, offering a comprehensive association between employees and their respective departments based on a compound key.
Conclusion
So, overall, the understanding the syntax and usage of inner joins in LINQ to SQL is essential for efficient data querying. By employing the join and on keywords, developers can seamlessly combine records from different tables based on specified conditions. Whether dealing with simple or complex join conditions, LINQ to SQL provides a versatile and expressive way to perform inner joins, enabling the retrieval of meaningful and consolidated data from relational databases. Mastering these techniques empowers developers to harness the full potential of LINQ to SQL for effective and streamlined data manipulation.
Share your thoughts in the comments
Please Login to comment...