Height of Binary Tree using JavaScript
Last Updated :
10 Apr, 2024
The height of a binary tree can be defined as the maximum depth or length of the longest path from the root node to any leaf node. Below is an example to illustrate the height of the binary tree.
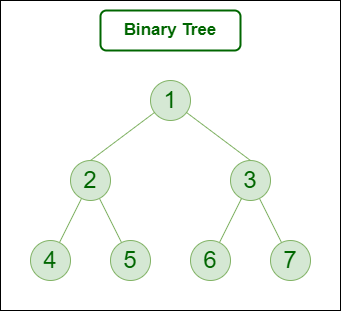
Height of Binary tree is 3
There are several approaches to find height of Binary tree using JavaScript which are as follows:
Iterative Approach
Create a function and set the initial height to 0 and create a queue with the root node. While queue is not empty , calculate the size of the current level by getting the length of the queue. Iterate over each node in current level and dequeue a node from queue and enqueue its left and right child if exist. Increase the height after processing each level. Return the height.
Example: To demonstrate how to find height of Binary tree using iterative approach
JavaScript
class TreeNode {
constructor(value) {
this.value = value;
this.left = null;
this.right = null;
}
}
// iterative approach
function heightOfBinaryTree(root) {
// height of empty tree is zero
if (root === null) {
return 0;
}
let height = 0;
let queue = [root];
while (queue.length !== 0) {
let levelSize = queue
.length;
for (let i = 0; i < levelSize; i++) {
let node = queue
.shift();
if (node.left) queue
.push(node.left);
if (node.right) queue
.push(node.right);
}
// Increment height
// after processing each level
height++;
}
return height;
}
const root = new TreeNode(11);
root.left = new TreeNode(12);
root.right = new TreeNode(13);
root.left.left = new TreeNode(14);
root.left.right = new TreeNode(15);
console.log(" height of binary tree using iterative approach is ",
heightOfBinaryTree(root));
Output height of binary tree using iterative approach is 3
Time Complexity : O(n)
Space Complexity : O(n)
Recursive Approach
If root is null , return height of binary tree zero. Recursively calculate the height of the left subtree by calling function with the left child of the root. Recursively calculate the height of the right subtree by calling function with the right child of the root. Return the maximum of the heights of left and right subtrees plus one (for root node).
Example: To demonstrate how to find height of Binary tree using Recursive approach
JavaScript
class TreeNode {
constructor(value) {
this.value = value;
this.left = null;
this.right = null;
}
}
// recursive approach
function heightOfBinaryTree(root) {
if (root === null) {
return 0;
}
const leftHeight = heightOfBinaryTree(root.left);
const rightHeight = heightOfBinaryTree(root.right);
return Math
.max(leftHeight, rightHeight) + 1;
}
const root = new TreeNode(11);
root.left = new TreeNode(12);
root.right = new TreeNode(13);
root.left.left = new TreeNode(14);
root.left.right = new TreeNode(15);
console.log(" height of binary tree using recursive approach is ",
heightOfBinaryTree(root));
Output height of binary tree using recursive approach is 3
Time Complexity : O(n)
Space Complexity : O(n)
Share your thoughts in the comments
Please Login to comment...