Getting Started with PHP
Last Updated :
01 Apr, 2024
PHP (Hypertext Preprocessor) is a powerful scripting language widely used for web development. Whether you’re looking to create dynamic web pages, handle form data, interact with databases, or build web applications, PHP has you covered. In this guide, we’ll take you through the basics of PHP, covering fundamental concepts and providing code examples along with their outputs to help you get started.
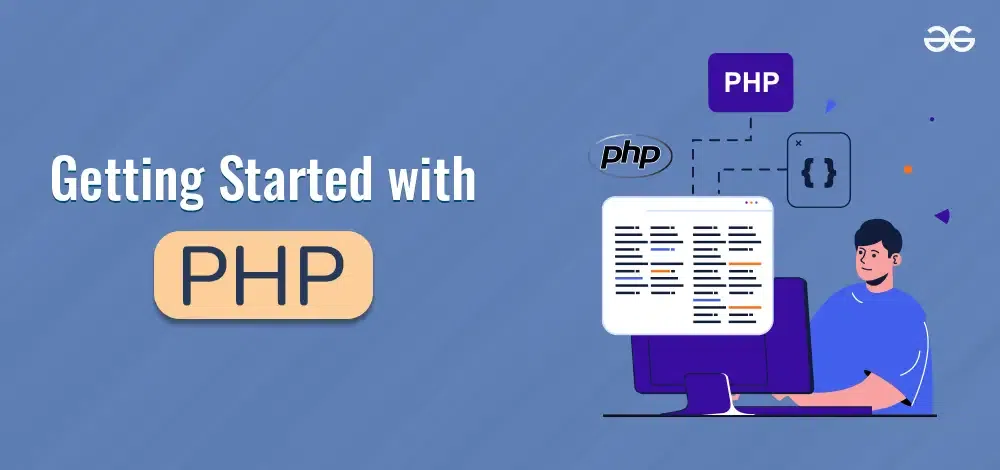
Introduction to PHP
PHP (Hypertext Preprocessor) is a server-side scripting language which was originally designed for web development. It operates on the server, dynamically generating HTML content, which is then sent to the client’s browser. This functionality enables websites to deliver dynamic and interactive content to users, enhancing their browsing experience.
Dynamic Content Generation:
- PHP enables the creation of dynamic web pages by embedding PHP code within HTML. This allows developers to generate HTML content dynamically based on various factors such as user input, database queries, or session data.
- For example, PHP can be used to retrieve user information from a database and dynamically generate personalized content for each user.
Interactivity and Responsiveness:
- With PHP, developers can create interactive web applications that respond to user actions in real-time.
- PHP scripts can process form data submitted by users, validate input, and provide immediate feedback or perform actions based on the input.
- For instance, PHP can handle user authentication, form submissions, and database operations seamlessly, making web applications more interactive and responsive.
Integration with Databases:
- PHP seamlessly integrates with various database management systems like MySQL, PostgreSQL, SQLite, and others.
- This integration allows for the development of dynamic web applications that can store and retrieve information from databases, such as user profiles, product catalogs, or content management systems.
Extensive Functionality and Libraries:
- PHP offers a vast array of built-in functions and libraries that simplify common web development tasks.
- From string manipulation and file handling to image processing and encryption, PHP provides robust functionality out of the box, reducing development time and effort.
Basic Concepts in PHP
Data Types:
PHP supports the following data types:
- Integers: Integers are whole numbers, either positive or negative, without any decimal point. They can range from -2,147,483,648 to 2,147,483,647 on a 32-bit system, and much larger values on a 64-bit system.
- Floats: Floats, also known as floating-point numbers or doubles, are numbers that have a decimal point or use exponential notation. They can represent a wider range of values, including fractions and very large or very small numbers.
- Strings: Strings are the sequences of characters which are enclosed within single quotes (”) or double quotes (“”). They can contain letters, numbers, symbols, and whitespace. Strings are commonly used to represent text data in PHP.
- Booleans: Booleans are simple data types that can have only two values: true or false. They are often used in conditional statements and comparisons to control the flow of execution in a PHP script.
- Arrays: Arrays are complex data types that can store multiple values in a single variable. They can hold elements of different data types and are indexed numerically or associatively. Arrays are versatile and commonly used for storing collections of data.
- Objects: Objects are instances of classes, which are user-defined data types in PHP. Objects can contain properties (variables) and methods (functions) that define their behavior. They are used for implementing object-oriented programming principles in PHP.
Variables in PHP are preceded by the dollar sign ($) and can store different types of data.
Example:
<?php
$integerVar = 10;
$floatVar = 3.14;
$stringVar = "Hello, PHP!";
$boolVar = true;
$arrayVar = array("apple", "banana", "orange");
?>
Control Statements:
In PHP, control statements like if…else, switch, and the ternary operator (?:) play a crucial role in decision making, allowing developers to execute specific blocks of code based on certain conditions. Let’s delve into each of these control statements to understand their functionality and usage in more detail:
If Else Statement:
The if else statement evaluates the given condition and executes a block of code only if the condition is true. If the condition is false, it executes an alternative block of code specified by the else clause.
Switch Statement:
The switch statement evaluates an expression and executes different blocks of code based on the value of the expression. It provides a more concise alternative to multiple if-else statements.
Ternary Operator (?:):
The ternary operator is a shorthand version of the if…else statement and is used to assign a value based on a condition.
Loops:
In PHP, loops are essential constructs used to execute a block of code repeatedly based on a specific condition. Let’s delve into the various types of loops supported by PHP and understand their syntax, functionality, and usage in detail:
For Loop:
The for loop is used to iterate over a block of code a fixed number of times. It consists of an initialization, condition, and increment/decrement expression.
While Loop:
A while loop is a programming structure that repeatedly executes a block of code as long as a specified condition remains true. It iterates based on the truth of the condition, ensuring code execution until the condition evaluates to false.
Do-While Loop:
The do-while loop is a control flow structure that executes a block of code at least once, regardless of the condition. It then checks the condition, and if true, repeats the execution. This loop guarantees the block’s execution before evaluating the condition, suitable for initialization-dependent scenarios.
Functions:
Functions in PHP play a crucial role in organizing code, promoting reusability, and enhancing the maintainability of web applications. They encapsulate a set of instructions that can be called multiple times within a script or across different scripts.
Arrays:
An array in PHP is a fundamental and flexible data structure that enables you to store and organize multiple values within a single variable name. Arrays are widely used in PHP programming for various tasks such as storing lists of items, grouping related data, and managing collections of elements.
Key Features of Arrays in PHP:
- Index-Based Access
- Dynamic Size
- Mixed Data Types
Strings:
In PHP, a string is a fundamental data type that represents a sequence of characters, including letters, numbers, symbols, and whitespace, enclosed within single quotes (”) or double quotes (“”). Strings are extensively used in PHP for storing and manipulating textual data, such as user input, file contents, and database records..
Creating Strings:
- Strings are created using single quotes (”) or double quotes (“”).
PHP with HTML
To run PHP code embedded within HTML, you need to have access to a web server environment that supports PHP. You can set up a local development environment on your computer using software packages like XAMPP, WAMP, or MAMP, which provide Apache web server, MySQL database, and PHP interpreter. Alternatively, you can upload your PHP files to a web hosting provider that supports PHP scripting.
Once you have a web server environment set up, you can create a PHP file with HTML markup and save it with a .php extension. Then, you can access the PHP file through a web browser by navigating to its URL.
Example: This example shows the basic use of PHP in HTML file.
PHP
<!DOCTYPE html>
<html>
<head>
<title>PHP with HTML Example</title>
</head>
<body>
<h1>Welcome to My Website</h1>
<?php
// PHP code starts here
$name = "John";
echo "<p>Hello, $name!</p>";
// PHP code ends here
?>
<p>This is a paragraph written in HTML.</p>
</body>
</html>
Output:
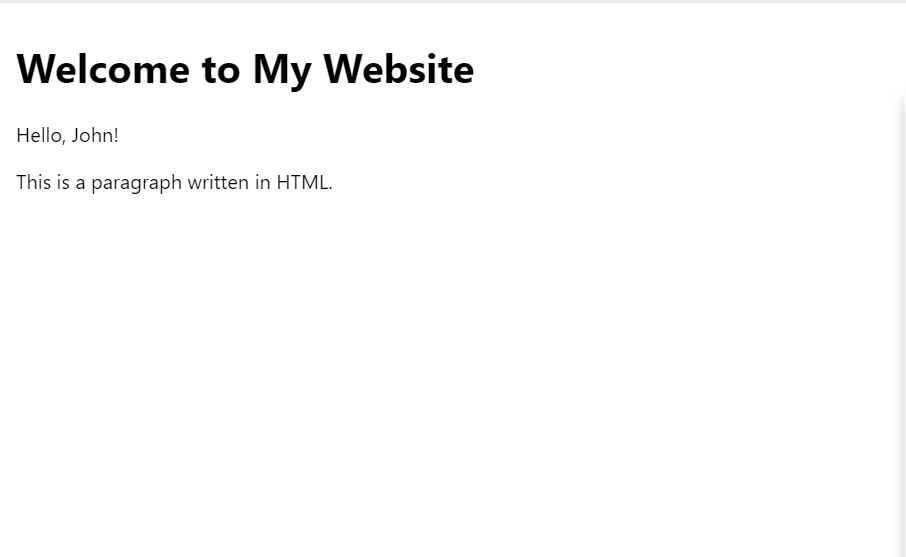
output
Advantages
- Versatility: PHP is versatile and can be used for a wide range of web development tasks, from creating simple websites to building complex web applications. Its flexibility allows developers to tailor solutions to specific project requirements.
- Cost-effective: PHP is open-source, meaning it is free to use and has a vast community of developers who contribute to its continuous improvement. This reduces development costs significantly, especially for startups and small businesses.
- Platform independence: PHP is compatible with various operating systems like Windows, Linux, and macOS, making it a platform-independent language. Developers can deploy PHP applications on different hosting environments without compatibility issues.
- Large ecosystem: PHP has a vast ecosystem of frameworks, libraries, and tools that streamline development and enhance productivity. Popular frameworks like Laravel, Symfony, and CodeIgniter provide robust structures and built-in functionalities for building scalable web applications.
Share your thoughts in the comments
Please Login to comment...