Getting Started with jQuery
Last Updated :
19 Mar, 2024
jQuery is a fast lightweight, and feature-rich JavaScript library that simplifies many common tasks in web development. It was created by John Resign and first released in 2006. It makes it easier to manipulate HTML documents, handle events, create animations, and interact with DOM(Document Object Model).
Prerequisites
How to use jQuery in a Project?
To use JQuery in your project, we must include JQuery in our project. There are several ways to do this task. In this part, I am using the CDN (Content Delivery Network) to do this task. Include this CDN link in your html document in the head tag.
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script>
</head>
jQuery Basics
Syntax:
$(selector).action()
Parameters:
- $: define/access the JQuery.
- selector: defines selecting the HTML element.
- action(): defines the action to be performed on the selector elements.
Example:
$("#test").hide()
Selecting Elements:
In JQuery, selecting elements is similar to CSS. JQuery selection allows you to target and manipulate HTML elements on a web page. The basic syntax for selecting elements in JQuery is similar to CSS selectors.
Syntax:
$("element_name_id_class")
Manipulating Elements:
In JQuery, manipulating elements involves selecting HTML elements and performing various actions like changing their content, modifiying attributes, applying styles, or handling events.
- Chaning Text Content: To change the text content of an element, you can use the ‘text()’ method.
$(document).ready(function() {
// Change text content of a paragraph
$('p').text('Geeks For Geeks');
});
- Changing HTML Content: To change the HTML content of an element, use the HTML () method.
$(document).ready(function() {
// Change HTML content of a div
$('#myDiv').html('<strong>New HTML content</strong>');
});
In JQuery, Handling events is very easy. It is also a crucial aspect of web development. Handling events allows you to respond to user interaction such as clicks, keypresses, or form submission. Here’s a basic example of Handling Events in JQuery
$(document).ready(function() {
// Selecting a button element
$('button').click(function() {
$('p').text ("Geeks for Geeks)
});
});
Ajax Requests:
Ajax(Asynchronous JavaScript and XML) allows you to send and receive data from the server without reloading the entire page. JQuery simplifies this process with its AJAX methods. There are many methods in JQuery for handling AJAX. Here, I have give very simple example to load the data using AJAX request. you can read more about ajax() method.
Filename: data.txt
Geeks For Geeks
Geeks for Geeks
Geeks for Geeks
Steps to run the code
Running a jQuery project involves a few key steps. jQuery is a JavaScript library, so you’ll need to create HTML, CSS, and JavaScript files to build your project.
Step 1: Set Up Your Project
- Create a new folder for your project in your computer system.
- Inside the Project folder, create three files: ‘index.html’, ‘style.css’ and ‘script.js’. That file may be change according your need. But one file must create ‘index.html’.
Step 2: Include jQuery Library:
- In the ‘<head>’ section of your HTML file, include the jQuery library from a CDN (Content Delivery Network) using the script tag. You can get the CDN link from the jQuery Website.
Step 3: Run Your Project
- Open the ‘index.html’ file in a web browser. You can do this by right clicking on the file and selecting “Open with” and choosing your preferred browser.
Advantages of using jQuery
These are the advantagse of using JQuery:
- DOM manipulation: JQuery simplifies the process of traversing and manipulating the Document Object Mode(DOM), making it more intutive and accessible.
- Event Handling: In JQuery simplifies the the process of handling the event, making it easier to attach and handle events such as clicks, keypreses. and more.
- AJAX support: JQuery provides a set of functions, making it easier to fetch and manipulate data from aserver without requirring a full page referesh.
Example: This example shows the event o click by using jQuery.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Geeks for Geeks Project</title>
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js">
</script>
</head>
<body>
<p>
I am Technical Writer
</p>
<button id="btn">
Click Me for changing Text
</button>
<script>
$(document).ready(function(){
$("#btn").click(function(){
$('p').load('data.txt') ;
});
});
</script>
</body>
</html>
Output:
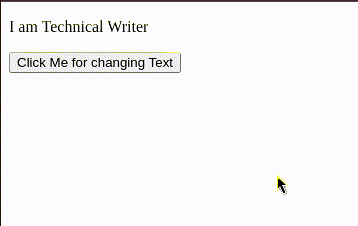
Conclusion
In Conclusion, getting started with JQuery provides a powerful and efficent way to enhance a web development by simplifying common task such as DOM manipulation, event handling, and AJAX requests. It accessible for both beginners and experienced developers. JQuery allows you to achieve complex operations with minimal code, resulting in faster development and improvied cross-browser compatiblity.
Share your thoughts in the comments
Please Login to comment...