Get Size of the Pandas DataFrame
Last Updated :
28 Nov, 2021
In this article, we will discuss how to get the size of the Pandas Dataframe using Python.
Method 1 : Using df.size
This will return the size of dataframe i.e. rows*columns
Syntax:
dataframe.size
where, dataframe is the input dataframe
Example: Python code to create a student dataframe and display size
Python3
import pandas as pd
data = pd.DataFrame({
'name' : [ 'sravan' , 'ojsawi' , 'bobby' , 'rohith' , 'gnanesh' ],
'subjects' : [ 'java' , 'php' , 'html/css' , 'python' , 'R' ],
'marks' : [ 98 , 90 , 78 , 91 , 87 ]
})
print (data)
data.size
|
Output:
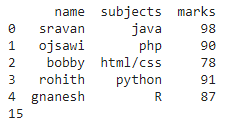
Method 2 : Using df.shape
This function will return the number of rows and columns in the dataframe
Syntax:
dataframe.shape
where, dataframe is the input dataframe
Example: Python program to get the shape of the dataframe
Python3
import pandas as pd
data = pd.DataFrame({
'name' : [ 'sravan' , 'ojsawi' , 'bobby' , 'rohith' , 'gnanesh' ],
'subjects' : [ 'java' , 'php' , 'html/css' , 'python' , 'R' ],
'marks' : [ 98 , 90 , 78 , 91 , 87 ]
})
print (data)
data.shape
|
Output:
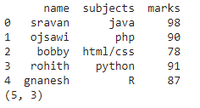
Method 3: Using df.ndim
This will return the number of dimensions present in the dataframe.
Syntax:
data.ndim
where, dataframe is the input dataframe
Example: Python program to get the dimension of the dataframe
Python3
import pandas as pd
data = pd.DataFrame({
'name' : [ 'sravan' , 'ojsawi' , 'bobby' , 'rohith' , 'gnanesh' ],
'subjects' : [ 'java' , 'php' , 'html/css' , 'python' , 'R' ],
'marks' : [ 98 , 90 , 78 , 91 , 87 ]
})
print (data)
data.ndim
|
Output:
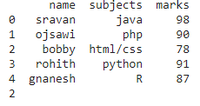
Share your thoughts in the comments
Please Login to comment...