Foreground Service in Android
Last Updated :
04 Sep, 2023
A foreground service has higher priority than other types of service. It is designed to perform long-running operations indicating service is running by providing notification to the user. Foreground services are used for tasks that require ongoing user awareness and interaction, such as playing music, tracking location, or performing downloads. By running a service in the foreground, you ensure that the system doesn’t kill the service due to memory constraints, and the user is aware of the ongoing operation through the notification.
Step-by-Step Implementation
To create a foreground service in Android, you need to follow these steps:
Step 1. Create a new class that extends the Service class and overrides its methods. This will be your foreground service implementation. For example:
Kotlin
class MyService: Service() {
override fun onBind(p0: Intent?): IBinder? {
return null
}
override fun onCreate() {
super .onCreate()
}
override fun onStartCommand(intent: Intent?, flags: Int, startId: Int): Int {
val name=intent?.getStringExtra( "name" )
Toast.makeText(
applicationContext, "Service has started running in the background" ,
Toast.LENGTH_SHORT
).show()
if (name != null ) {
Log.d( "Service Name" ,name)
}
Log.d( "Service Status" , "Starting Service" )
for (i in 1 .. 10 )
{
Thread.sleep( 100 )
Log.d( "Status" , "Service $i" )
}
stopSelf()
return START_STICKY
}
override fun stopService(name: Intent?): Boolean {
Log.d( "Stopping" , "Stopping Service" )
return super .stopService(name)
}
override fun onDestroy() {
Toast.makeText(
applicationContext, "Service execution completed" ,
Toast.LENGTH_SHORT
).show()
Log.d( "Stopped" , "Service Stopped" )
super .onDestroy()
}
}
|
Step 2. In your AndroidManifest.xml file, declare the service as a separate component inside the tag. For example:
<service android:name="MyService"
tools:ignore="Instantiatable" />
Step 3. Start the foreground service from your activity or other parts of your app using an intent:
Kotlin
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super .onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val button: Button = findViewById(R.id.button)
button.setOnClickListener {
val intent=Intent( this ,MyService:: class .java)
intent.putExtra( "name" , "Geek for Geeks" )
startService(intent)
}
}
}
|
Output:
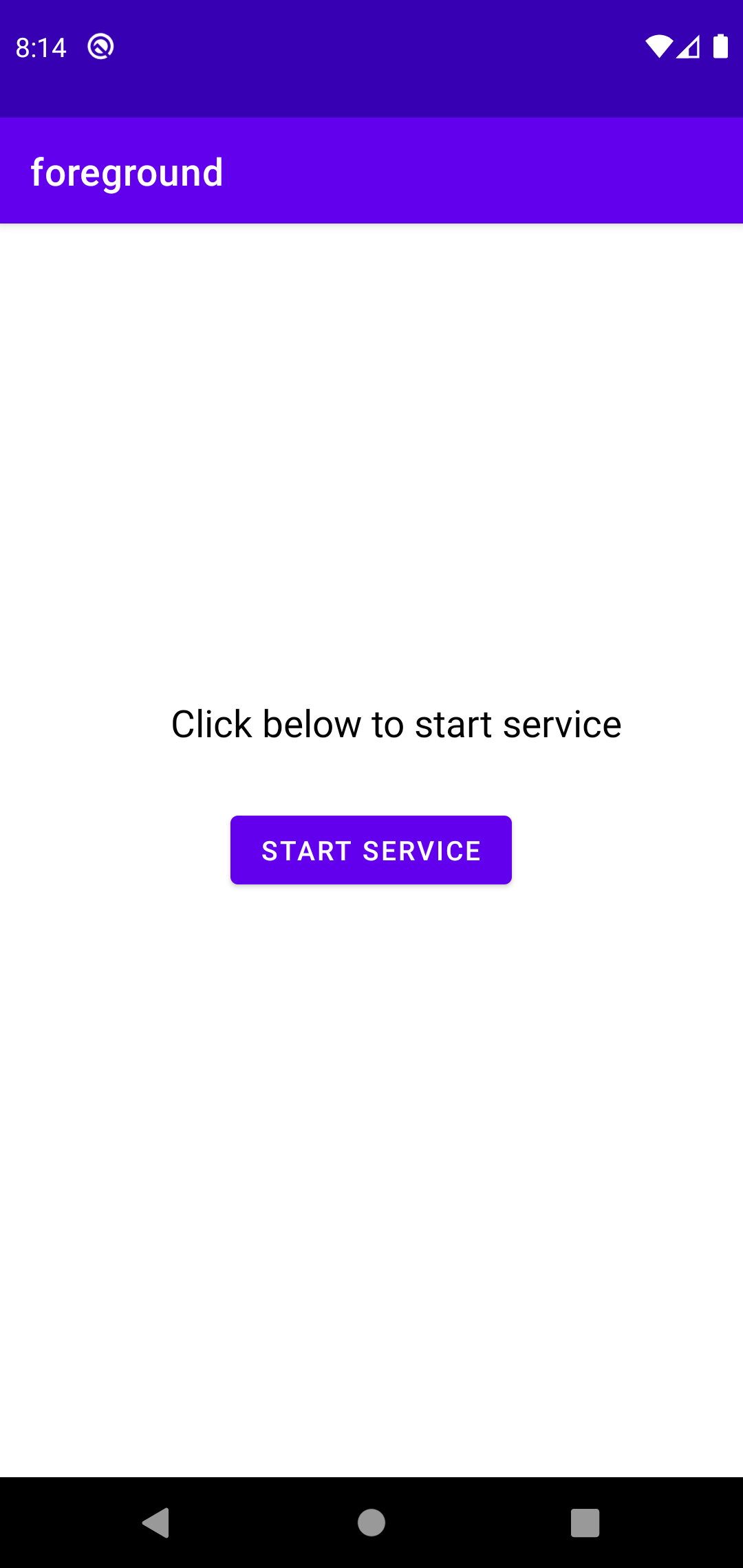
Logs:
2023-07-08 20:14:27.283 17612-17612/com.example.foreground D/Service Name: Geek for Geeks
2023-07-08 20:14:27.283 17612-17612/com.example.foreground D/Service Status: Starting Service
2023-07-08 20:14:27.389 17612-17612/com.example.foreground D/Status: Service 1
2023-07-08 20:14:27.493 17612-17612/com.example.foreground D/Status: Service 2
2023-07-08 20:14:27.599 17612-17612/com.example.foreground D/Status: Service 3
2023-07-08 20:14:27.701 17612-17612/com.example.foreground D/Status: Service 4
2023-07-08 20:14:27.807 17612-17612/com.example.foreground D/Status: Service 5
2023-07-08 20:14:27.911 17612-17612/com.example.foreground D/Status: Service 6
2023-07-08 20:14:28.014 17612-17612/com.example.foreground D/Status: Service 7
2023-07-08 20:14:28.118 17612-17612/com.example.foreground D/Status: Service 8
2023-07-08 20:14:28.221 17612-17612/com.example.foreground D/Status: Service 9
2023-07-08 20:14:28.325 17612-17612/com.example.foreground D/Status: Service 10
2023-07-08 20:14:28.461 17612-17612/com.example.foreground D/Stopped: Service Stopped
Explanation:
- startForegroundService() indicates system will be running in background. Once started, you can perform your long-running tasks within the service’s onStartCommand() method. Remember to call startForeground() with a notification to make the service run in the foreground.
- stopForeground(true) is called when foreground service is not needed to remove the foreground status and notification within the service’s onDestroy() method. This allows the service to be properly stopped and cleaned up.
Note: Starting from Android 10 (API level 29), foreground services have some additional restrictions to prevent abuse. You may need to request the FOREGROUND_SERVICE permission in your app’s manifest and display a system-level notification to the user when starting a foreground service. Make sure to review the official Android documentation and guidelines for foreground services for the specific requirements and best practices applicable to your target Android version.
In conclusion, a foreground service in Android is a type of service that runs with higher priority than regular background services. It is used for long-running operations that require ongoing user awareness and interaction. By running a service in the foreground, you ensure that the service continues to run even when the app is in the background, and you provide a persistent notification to the user indicating the ongoing operation.
To create a foreground service, you need to extend the Service class, override its methods, and implement the desired functionality. You should call startForeground() with a notification to indicate that your service is running in the foreground. This notification keeps the user informed about the ongoing operation. When the service is no longer needed, you can call stopForeground(true) to remove the foreground status and notification.
However, starting from Android 10 (API level 29), foreground services have some additional restrictions to prevent abuse. You may need to request the FOREGROUND_SERVICE permission and display a system-level notification when starting a foreground service. It’s crucial to review the official Android documentation and guidelines for foreground services to ensure compliance with the latest requirements and best practices for your target Android version.
Overall, foreground services are a powerful tool in Android for running long-lasting operations while keeping the user informed and engaged through persistent notifications.
Share your thoughts in the comments
Please Login to comment...