Flatten Specific Dimensions of NumPy Array
Last Updated :
13 Oct, 2023
A general-purpose array-processing package that is used for working with arrays is called NumPy. Do you want to collapse your Numpy array into one dimension? If yes, then you can do so by flattening your Numpy array. In this article, we will see how we can flatten only some dimensions of a Numpy array.
Flatten only some dimensions of a NumPy Array
- Using reshape() function
- Using reshape() with shape[] function
- Using numpy.vstack()
Using reshape() function
The function used to change the shape of an array, i.e., changing the number of elements in each dimension is known as the reshape function(). In this way, we will see how we can flatten some dimensions of the Numpy array using the reshape function.
Python3
import numpy as np
arr = np.zeros(( 5 , 2 , 5 ))
flattened_arr = arr.reshape( 10 , 5 )
print (flattened_arr)
|
Output:
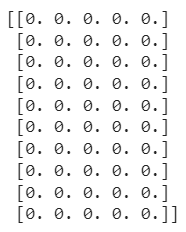
Using reshape() with shape[] function
The function which is used to give a new shape to an array without changing its data is known as reshape function(), while shape function is used to calculate the number of elements in each dimension of an array. In this way, we will see how we can flatten some dimensions of numpy array using reshape along with shape[] function.
Python3
import numpy as np
arr = np.zeros(( 5 , 2 , 5 ))
flattened_arr = arr.reshape( - 2 , arr.shape[ - 2 ])
print (flattened_arr)
|
Output:

Using numpy.vstack()
numpy.vstack() function is used to stack the sequence of input arrays vertically to make a single array.Here this way, we will see how we can flatten some dimensions of numpy array using numpy.vstack() function.
Python
import numpy as np
arr = np.zeros(( 3 , 1 , 4 ))
print (arr)
new_arr = np.vstack(arr)
print (new_arr)
|
Output:
[[0. 0. 0. 0.]
[0. 0. 0. 0.]
[0. 0. 0. 0.]]
Share your thoughts in the comments
Please Login to comment...