First non-repeating character using one traversal of string | Set 2
Last Updated :
27 Mar, 2023
Given a string, find the first non-repeating character in it. For example, if the input string is “GeeksforGeeks”, then output should be ‘f’ and if input string is “GeeksQuiz”, then output should be ‘G’.
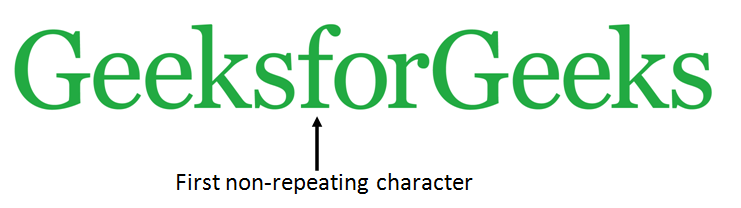
We have discussed two solutions in Given a string, find its first non-repeating character . In this post, a further optimized solution (over method 2 of previous post) is discussed. The idea is to optimize space. Instead of using a pair to store count and index, we use single element that stores index if an element appears once, else stores a negative value.
Implementation:
C++
#include <bits/stdc++.h>
using namespace std;
#define NO_OF_CHARS 256
int firstNonRepeating( char * str)
{
int arr[NO_OF_CHARS];
for ( int i = 0; i < NO_OF_CHARS; i++)
arr[i] = -1;
for ( int i = 0; str[i]; i++) {
if (arr[str[i]] == -1)
arr[str[i]] = i;
else
arr[str[i]] = -2;
}
int res = INT_MAX;
for ( int i = 0; i < NO_OF_CHARS; i++)
if (arr[i] >= 0)
res = min(res, arr[i]);
return res;
}
int main()
{
char str[] = "geeksforgeeks" ;
int index = firstNonRepeating(str);
if (index == INT_MAX)
cout << "Either all characters are "
"repeating or string is empty" ;
else
cout << "First non-repeating character"
" is " << str[index];
return 0;
}
|
C
#include <limits.h>
#include <stdio.h>
#include <math.h>
#define NO_OF_CHARS 256
int firstNonRepeating( char * str)
{
int arr[NO_OF_CHARS];
for ( int i = 0; i < NO_OF_CHARS; i++)
arr[i] = -1;
for ( int i = 0; str[i]; i++) {
if (arr[str[i]] == -1)
arr[str[i]] = i;
else
arr[str[i]] = -2;
}
int res = INT_MAX;
for ( int i = 0; i < NO_OF_CHARS; i++)
if (arr[i] >= 0)
res = min(res, arr[i]);
return res;
}
int main()
{
char str[] = "geeksforgeeks" ;
int index = firstNonRepeating(str);
if (index == INT_MAX)
printf ( "Either all characters are "
"repeating or string is empty" );
else
printf ( "First non-repeating character"
" is %c" , str[index]);
return 0;
}
|
Java
import java.io.*;
import java.util.*;
import java.lang.*;
class GFG
{
static int firstNonRepeating(String str)
{
int NO_OF_CHARS = 256 ;
int arr[] = new int [NO_OF_CHARS];
for ( int i = 0 ;
i < NO_OF_CHARS; i++)
arr[i] = - 1 ;
for ( int i = 0 ;
i < str.length(); i++)
{
if (arr[str.charAt(i)] == - 1 )
arr[str.charAt(i)] = i;
else
arr[str.charAt(i)] = - 2 ;
}
int res = Integer.MAX_VALUE;
for ( int i = 0 ; i < NO_OF_CHARS; i++)
if (arr[i] >= 0 )
res = Math.min(res, arr[i]);
return res;
}
public static void main(String args[])
{
String str = "geeksforgeeks" ;
int index = firstNonRepeating(str);
if (index == Integer.MAX_VALUE)
System.out.print( "Either all characters are " +
"repeating or string is empty" );
else
System.out.print( "First non-repeating character" +
" is " + str.charAt(index));
}
}
|
Python3
import math as mt
NO_OF_CHARS = 256
def firstNonRepeating(string):
arr = [ - 1 for i in range (NO_OF_CHARS)]
for i in range ( len (string)):
if arr[ ord (string[i])] = = - 1 :
arr[ ord (string[i])] = i
else :
arr[ ord (string[i])] = - 2
res = 10 * * 18
for i in range (NO_OF_CHARS):
if arr[i]> = 0 :
res = min (res,arr[i])
return res
string = "geeksforgeeks"
index = firstNonRepeating(string)
if index = = 10 * * 18 :
print ( "Either all characters are repeating or string is empty" )
else :
print ( "First non-repeating character is" ,string[index])
|
C#
using System;
class GFG
{
static int firstNonRepeating(String str)
{
int NO_OF_CHARS = 256;
int []arr = new int [NO_OF_CHARS];
for ( int i = 0; i < NO_OF_CHARS; i++)
arr[i] = -1;
for ( int i = 0; i < str.Length; i++)
{
if (arr[str[i]] == -1)
arr[str[i]] = i;
else
arr[str[i]] = -2;
}
int res = int .MaxValue;
for ( int i = 0; i < NO_OF_CHARS; i++)
if (arr[i] >= 0)
res = Math.Min(res, arr[i]);
return res;
}
public static void Main()
{
String str = "geeksforgeeks" ;
int index = firstNonRepeating(str);
if (index == int .MaxValue)
Console.Write( "Either all characters are " +
"repeating or string is empty" );
else
Console.Write( "First non-repeating character" +
" is " + str[index]);
}
}
|
Javascript
<script>
function firstNonRepeating(str)
{
let NO_OF_CHARS = 256;
let arr = new Array(NO_OF_CHARS);
for (let i = 0;
i < NO_OF_CHARS; i++)
arr[i] = -1;
for (let i = 0;
i < str.length; i++)
{
if (arr[str[i].charCodeAt(0)] == -1)
arr[str[i].charCodeAt(0)] = i;
else
arr[str[i].charCodeAt(0)] = -2;
}
let res = Number.MAX_VALUE;
for (let i = 0; i < NO_OF_CHARS; i++)
if (arr[i] >= 0)
res = Math.min(res, arr[i]);
return res;
}
let str = "geeksforgeeks" ;
let index = firstNonRepeating(str);
if (index == Number.MAX_VALUE)
document.write( "Either all characters are " +
"repeating or string is empty" );
else
document.write( "First non-repeating character" +
" is " + str[index]);
</script>
|
Output
First non-repeating character is f
Time Complexity: O(n)
Auxiliary Space: O(1)
Alternate Implementation:
This is coded using a HashMap or Hashing Technique.
If the element(or key) repeats in the string the HashMap (or Dictionary) will change the value of that key to None.
This way we will later on only be finding keys whose value is “not None”.
Implementation:
C++
#include <bits/stdc++.h>
#include <unordered_map>
using namespace std;
unordered_map< char , int > makeHashMap(string string)
{
unordered_map< char , int > d1;
char c;
for ( int i = 0; i < string.length(); i++) {
c = string[i];
if (d1.find(c) != d1.end()) {
d1++;
}
else
d1 = 1;
}
for ( auto it = d1.begin(); it != d1.end(); it++)
{
if (it->second == 1)
it->second = 0;
else
it->second = -1;
}
return d1;
}
char firstNotRepeatingCharacter(string s)
{
unordered_map< char , int > d = makeHashMap(s);
char nonRep = '.' ;
for ( int i = 0; i < s.length(); i++) {
char c = s[i];
if (d == 0) {
nonRep = c;
break ;
}
}
if (nonRep == '.' )
return '_' ;
else
return nonRep;
}
int main()
{
string s = "bbcdcca" ;
cout << firstNotRepeatingCharacter(s);
return 0;
}
|
Java
import java.util.HashMap;
class GFG {
public static HashMap<Character, Integer> makeHashMap(String string)
{
HashMap<Character, Integer> d1 =
new HashMap<Character, Integer>();
char c;
for ( int i = 0 ; i < string.length(); i++) {
c = string.charAt(i);
if (d1.containsKey(c)) {
Integer oldValue = d1.get(c);
d1.put(c, oldValue + 1 );
}
else
d1.put(c, 1 );
}
for (Character key : d1.keySet()) {
if (d1.get(key) == 1 )
d1.put(key, 0 );
else
d1.put(key, null );
}
return d1;
}
public static Character firstNotRepeatingCharacter(String s)
{
HashMap<Character, Integer> d = makeHashMap(s);
Character nonRep = null ;
for ( int i = 0 ; i < s.length(); i++) {
Character c = s.charAt(i);
if (d.get(c) != null ) {
nonRep = c;
break ;
}
}
if (nonRep == null )
return '_' ;
else
return nonRep;
}
public static void main(String args[])
{
String s = "bbcdcca" ;
System.out.println(firstNotRepeatingCharacter(s));
}
}
|
Python3
from collections import Counter
def makeHashMap(string):
d1 = Counter(string)
d1 = {(key): ( 0 if d1[key] = = 1 else None )
for key, value in d1.items()}
return d1
def firstNotRepeatingCharacter(s):
d = makeHashMap(s)
nonRep = None
for i in s:
if d[i] is not None :
nonRep = i
break
if nonRep is not None :
return nonRep
else :
return str ( "_" )
print (firstNotRepeatingCharacter( 'bbcdcca' ))
|
C#
using System;
using System.Collections.Generic;
public class Program {
public static Dictionary< char , int >
MakeHashMap( string s)
{
var d1 = new Dictionary< char , int >();
foreach ( char c in s)
{
if (d1.ContainsKey(c)) {
d1++;
}
else {
d1 = 1;
}
}
var d2 = new Dictionary< char , int >();
foreach ( var kvp in d1)
{
if (kvp.Value == 1) {
d2[kvp.Key] = 0;
}
else {
d2[kvp.Key] = -1;
}
}
return d2;
}
public static char FirstNotRepeatingCharacter( string s)
{
var d = MakeHashMap(s);
char nonRep = '.' ;
foreach ( char c in s)
{
if (d == 0) {
nonRep = c;
break ;
}
}
if (nonRep == '.' ) {
return '_' ;
}
else {
return nonRep;
}
}
public static void Main()
{
string s = "bbcdcca" ;
Console.WriteLine(FirstNotRepeatingCharacter(s));
}
}
|
Javascript
function makeHashMap(string) {
let d1 = new Map();
for (let i = 0; i < string.length; i++) {
let char = string.charAt(i);
if (d1.has(char)) {
d1.set(char, d1.get(char) + 1);
} else {
d1.set(char, 1);
}
}
d1.forEach( function (value, key) {
if (value === 1) {
d1.set(key, 0);
} else {
d1.set(key, null );
}
});
return d1;
}
function firstNotRepeatingCharacter(s) {
let d = makeHashMap(s);
let nonRep = null ;
for (let i = 0; i < s.length; i++) {
let char = s.charAt(i);
if (d.get(char) !== null ) {
nonRep = char;
break ;
}
}
if (nonRep !== null ) {
return nonRep;
} else {
return "_" ;
}
}
console.log(firstNotRepeatingCharacter( "bbcdcca" ));
|
Time Complexity: O(N)
Auxiliary Space: O(N)
Method 3: Another simple approach to this problem without using any hashmap or array is mentioned below. We can find the first non-repeating character by just using single for loop.
Another Approach:
To count the frequency of character we can do the following step:
frequency of a character = length_of_string – length_of_string_without_that _character
for example: Given String is “helloh” and we want to count frequency of character “h” so by using the above formula we can say
frequency of character “h” = 6(length of string) – 4(length of string without “h”) = 2
So by this way, we can count the frequency of every character in a string and then if we found count == 1 that means that character is the first non-repeating character in the string.
Implementation: Implementation of the above method in java is shown below:
C++
#include<bits/stdc++.h>
using namespace std;
void firstNonRepeating(string s)
{
bool flag = false ;
int index = -1;
for ( int i = 0; i < s.size(); i++) {
string temp = s;
temp.erase( remove (temp.begin(), temp.end(), s[i]), temp.end());
int count_occurrence = s.size() - temp.size();
if (count_occurrence == 1)
{
flag = true ;
index = i;
break ;
}
}
if (flag)
cout << "First non repeating character is " << s[index] << endl;
else
cout << "There is no non-repeating character is present in the string" << endl;
}
int main(){
string s = "GeeksforGeeks" ;
firstNonRepeating(s);
return 0;
}
|
Java
public class first_non_repeating {
static void firstNonRepeating(String s)
{
boolean flag = false ;
int index = - 1 ;
for ( int i = 0 ; i < s.length(); i++) {
int count_occurrence
= s.length()
- s.replace(
Character.toString(s.charAt(i)),
"" )
.length();
if (count_occurrence == 1 ) {
flag = true ;
index = i;
break ;
}
}
if (flag)
System.out.println(
"First non repeating character is "
+ s.charAt(index));
else
System.out.println(
"There is no non-repeating character is present in the string" );
}
public static void main(String arg[])
{
String s = "GeeksforGeeks" ;
firstNonRepeating(s);
}
}
|
Python3
def firstNonRepeating(s):
flag = False
index = - 1
for i in range ( len (s)):
count_occurrence = len (s) - len (s.replace(s[i],''))
if (count_occurrence = = 1 ):
flag = True
index = i
break
if (flag):
print (f "First non repeating character is {s[index]}" )
else :
print ( "There is no non-repeating character is present in the string" )
s = "GeeksforGeeks"
firstNonRepeating(s)
|
C#
using System;
class first_non_repeating {
static void firstNonRepeating( string s)
{
bool flag = false ;
int index = -1;
for ( int i = 0; i < s.Length; i++) {
int count_occurrence
= s.Length
- s.Replace(Char.ToString(s[i]), "" )
.Length;
if (count_occurrence == 1) {
flag = true ;
index = i;
break ;
}
}
if (flag) {
Console.WriteLine(
"First non repeating character is "
+ s[index]);
}
else {
Console.WriteLine(
"There is no non-repeating character is present in the string" );
}
}
public static void Main()
{
string s = "GeeksforGeeks" ;
firstNonRepeating(s);
}
}
|
Javascript
<script>
function firstNonRepeating(s)
{
let flag = false ;
let index = -1;
for (let i = 0; i < s.length; i++) {
let count_occurrence
= s.length - s.replaceAll(s[i], '' ).length;
if (count_occurrence == 1)
{
flag = true ;
index = i;
break ;
}
}
if (flag)
document.write(
"First non repeating character is "
+ s[index], "</br>" );
else
document.write(
"There is no non-repeating character is present in the string" , "</br>" );
}
let s = "GeeksforGeeks"
firstNonRepeating(s)
</script>
|
Output
First non repeating character is f
Time Complexity: O(N)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...