Find the Largest number with given number of digits and sum of digits
Last Updated :
18 Apr, 2023
Given an integer s and d, The task is to find the largest number with given digit sum s and the number of digits d.
Examples:
Input: s = 9, d = 2
Output: 90
Input: s = 20, d = 3
Output: 992
Naive Approach:
Consider all m digit numbers and keep a max variable to store the maximum number with m digits and digit sum as s.
Time complexity: O(10m).
Auxiliary Space: O(1)
Find the Largest number with the given number of digits and sum of digits Greedy approach
Below is the idea to solve the problem:
The idea is to one by one fill all digits from leftmost to rightmost compare the remaining sum with 9 if the remaining sum is more than or equal to 9, 9 at the current position, else put the remaining sum. Since digits are filled from left to right, the highest digits will be placed on the left side, hence get the largest number y.
Illustration:
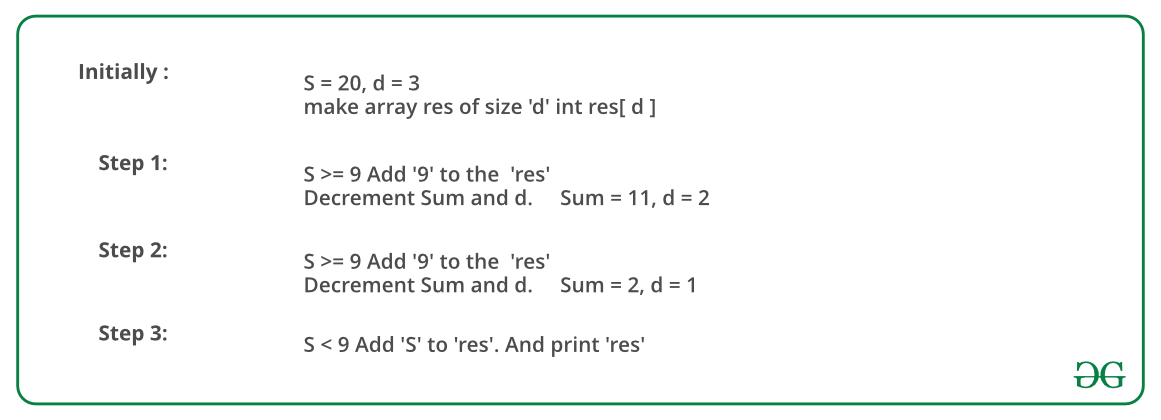
Follow the below steps to Implement the idea:
- If s is zero
- if m=1 print 0
- Else no such number is possible.
- If s > 9*m then no such number is possible.
- Run a for loop from 0 to m-1
- If s>=9 subtract 9 from s and print 9.
- Else print s and set s to 0.
Below is the Implementation of the above approach:
C++
#include <iostream>
using namespace std;
void findLargest( int m, int s)
{
if (s == 0) {
(m == 1) ? cout << "Largest number is " << 0
: cout << "Not possible" ;
return ;
}
if (s > 9 * m) {
cout << "Not possible" ;
return ;
}
int res[m];
for ( int i = 0; i < m; i++) {
if (s >= 9) {
res[i] = 9;
s -= 9;
}
else {
res[i] = s;
s = 0;
}
}
cout << "Largest number is " ;
for ( int i = 0; i < m; i++)
cout << res[i];
}
int main()
{
int s = 9, m = 2;
findLargest(m, s);
return 0;
}
|
C
#include <stdio.h>
void findLargest( int m, int s)
{
if (s == 0) {
(m == 1) ? printf ( "Largest number is 0" )
: printf ( "Not possible" );
return ;
}
if (s > 9 * m) {
printf ( "Not possible" );
return ;
}
int res[m];
for ( int i = 0; i < m; i++) {
if (s >= 9) {
res[i] = 9;
s -= 9;
}
else {
res[i] = s;
s = 0;
}
}
printf ( "Largest number is " );
for ( int i = 0; i < m; i++)
printf ( "%d" , res[i]);
}
int main()
{
int s = 9, m = 2;
findLargest(m, s);
return 0;
}
|
Java
class GFG
{
static void findLargest( int m, int s)
{
if (s == 0 )
{
System.out.print(m == 1 ? "Largest number is 0" : "Not possible" );
return ;
}
if (s > 9 *m)
{
System.out.println( "Not possible" );
return ;
}
int [] res = new int [m];
for ( int i= 0 ; i<m; i++)
{
if (s >= 9 )
{
res[i] = 9 ;
s -= 9 ;
}
else
{
res[i] = s;
s = 0 ;
}
}
System.out.print( "Largest number is " );
for ( int i= 0 ; i<m; i++)
System.out.print(res[i]);
}
public static void main (String[] args)
{
int s = 9 , m = 2 ;
findLargest(m, s);
}
}
|
Python3
def findLargest( m, s) :
if (s = = 0 ) :
if (m = = 1 ) :
print ( "Largest number is " , "0" ,end = "")
else :
print ( "Not possible" ,end = "")
return
if (s > 9 * m) :
print ( "Not possible" ,end = "")
return
res = [ 0 ] * m
for i in range ( 0 , m) :
if (s > = 9 ) :
res[i] = 9
s = s - 9
else :
res[i] = s
s = 0
print ( "Largest number is " ,end = "")
for i in range ( 0 , m) :
print (res[i],end = "")
s = 9
m = 2
findLargest(m, s)
|
C#
using System;
class GFG
{
static void findLargest( int m, int s)
{
if (s == 0)
{
Console.Write(m == 1 ?
"Largest number is 0" :
"Not possible" );
return ;
}
if (s > 9 * m)
{
Console.WriteLine( "Not possible" );
return ;
}
int []res = new int [m];
for ( int i = 0; i < m; i++)
{
if (s >= 9)
{
res[i] = 9;
s -= 9;
}
else
{
res[i] = s;
s = 0;
}
}
Console.Write( "Largest number is " );
for ( int i = 0; i < m; i++)
Console.Write(res[i]);
}
static public void Main ()
{
int s = 9, m = 2;
findLargest(m, s);
}
}
|
PHP
<?php
function findLargest( $m , $s )
{
if ( $s == 0)
{
if (( $m == 1) == true)
echo "Largest number is " , 0;
else
echo "Not possible" ;
return ;
}
if ( $s > 9 * $m )
{
echo "Not possible" ;
return ;
}
for ( $i = 0; $i < $m ; $i ++)
{
if ( $s >= 9)
{
$res [ $i ] = 9;
$s -= 9;
}
else
{
$res [ $i ] = $s ;
$s = 0;
}
}
echo "Largest number is " ;
for ( $i = 0; $i < $m ; $i ++)
echo $res [ $i ];
}
$s = 9; $m = 2;
findLargest( $m , $s );
?>
|
Javascript
<script>
function findLargest(m, s)
{
if (s == 0)
{
(m == 1)? document.write( "Largest number is " + 0)
: document.write( "Not possible" );
return ;
}
if (s > 9*m)
{
document.write( "Not possible" );
return ;
}
let res = new Array(m);
for (let i=0; i<m; i++)
{
if (s >= 9)
{
res[i] = 9;
s -= 9;
}
else
{
res[i] = s;
s = 0;
}
}
document.write( "Largest number is " );
for (let i=0; i<m; i++)
document.write(res[i]);
}
let s = 9, m = 2;
findLargest(m, s);
</script>
|
Output
Largest number is 90
Time Complexity of this solution is O(m).
Auxiliary Space: O(m), where m is the given integer.
Approach: Greedy Algorithm
- Create an empty string to store the result
- If d is 1, append s to the result and return it
- Loop from the leftmost digit to the rightmost digit
a. If the remaining sum of digits is greater than or equal to 9, append 9 to the result and subtract 9 from the remaining sum of digits
b. If the remaining sum of digits is less than 9, append the remaining sum of digits to the result and fill the remaining digits with 0s
- Return the result
C++
#include <iostream>
#include <string>
using namespace std;
int largest_number( int s, int d) {
if (s == 0) {
return 0;
}
if (s > 9 * d) {
return -1;
}
string result = "" ;
for ( int i = 0; i < d; i++) {
if (s >= 9) {
result += '9' ;
s -= 9;
} else {
result += to_string(s);
s = 0;
}
if (s == 0 && i < d-1) {
result += string(d-i-1, '0' );
break ;
}
}
return stoi(result);
}
int main() {
cout << largest_number(9, 2) << endl;
cout << largest_number(20, 3) << endl;
return 0;
}
|
Java
import java.util.*;
public class Main {
public static int largest_number( int s, int d)
{
if (s == 0 ) {
return 0 ;
}
if (s > 9 * d) {
return - 1 ;
}
String result = "" ;
for ( int i = 0 ; i < d; i++) {
if (s >= 9 ) {
result += '9' ;
s -= 9 ;
}
else {
result += Integer.toString(s);
s = 0 ;
}
if (s == 0 && i < d - 1 ) {
result += String.join(
"" ,
Collections.nCopies(d - i - 1 , "0" ));
break ;
}
}
return Integer.parseInt(result);
}
public static void main(String[] args)
{
System.out.println(
largest_number( 9 , 2 ));
System.out.println(
largest_number( 20 , 3 ));
}
}
|
Python3
def largest_number(s, d):
if s = = 0 :
return 0
if s > 9 * d:
return - 1
result = ''
for i in range (d):
if s > = 9 :
result + = '9'
s - = 9
else :
result + = str (s)
s = 0
if s = = 0 and i < d - 1 :
result + = '0' * (d - i - 1 )
break
return int (result)
print (largest_number( 9 , 2 ))
print (largest_number( 20 , 3 ))
|
C#
using System;
class Program {
static int LargestNumber( int s, int d) {
if (s == 0) {
return 0;
}
if (s > 9 * d) {
return -1;
}
string result = "" ;
for ( int i = 0; i < d; i++) {
if (s >= 9) {
result += "9" ;
s -= 9;
} else {
result += s.ToString();
s = 0;
}
if (s == 0 && i < d - 1) {
result += new string ( '0' , d - i - 1);
break ;
}
}
return int .Parse(result);
}
static void Main( string [] args) {
Console.WriteLine(LargestNumber(9, 2));
Console.WriteLine(LargestNumber(20, 3));
}
}
|
Javascript
function largestNumber(s, d) {
if (s == 0) {
return 0;
}
if (s > 9 * d) {
return -1;
}
let result = "" ;
for (let i = 0; i < d; i++) {
if (s >= 9) {
result += "9" ;
s -= 9;
} else {
result += s.toString();
s = 0;
}
if (s == 0 && i < d - 1) {
result += "0" .repeat(d - i - 1);
break ;
}
}
return parseInt(result);
}
console.log(largestNumber(9, 2));
console.log(largestNumber(20, 3));
|
Time complexity: O(d),
Auxiliary space: O(d)
Share your thoughts in the comments
Please Login to comment...