FileInputStream skip() Method in Java with Examples
Last Updated :
01 Dec, 2021
FileInputStream class is quite helpful to read data from a file in the form of a sequence of bytes. FileInputStream is meant for reading streams of raw bytes such as image, audio, video, etc. For reading streams of characters, FileReader is recommended. It was firstly introduced in JDK 1.0.
FileInputStream skip() method
The skip(n) method in FileInputStream is quite helpful as it discards the n bytes of data from the beginning of the input stream. We can understand its working by considering the following example,
We are reading a stream of characters through FileInputStream, and we want to read it after skipping the first eight characters from the stream. Let the string be “GeeksforGeeks“, so after skipping the first eight characters, our string will become “Geeks“.
The skip() method is useful to achieve the task as mentioned above. This method is defined in FileInputStream class of the Java.io package.
Java I/O package helps the user to perform all the input-output operations. These streams support all objects, data types, characters, files, etc., to execute the I/O operations fully.
Syntax:
Input_File_Stream.skip(n)
Input_File_Stream: The file input stream.
Parameters:
- n − A Non-negative integer.
- Bytes to be skipped from a stream of characters.
Return Value: It returns the actual number of bytes skipped.
Exception: IOException − If n is a negative integer or I/O error occurs.
Example 1:
Java
import java.io.FileInputStream;
import java.io.IOException;
public class GeeksforGeeks {
public static void main(String[] args)
throws IOException
{
FileInputStream inputFileStream = null ;
int integerValue = 0 ;
char characterValue;
try {
inputFileStream = new FileInputStream(
"C:\\Users\\harsh\\Desktop\\GFG\\inputFile.txt" );
inputFileStream.skip( 4 );
integerValue = inputFileStream.read();
characterValue = ( char )i;
System.out.print( "Character read: "
+ characterValue);
}
catch (Exception exception) {
exception.printStackTrace();
}
finally {
if (inputFileStream != null )
inputFileStream.close();
}
}
}
|
Steps for Compilation in a Local System:
1. We have saved the above program with the name “GeeksforGeeks” locally:

Source code file
2. Lets now create a text file named “inputFile“:

Text file
3. We need to enter some text inside the created text file. As an example, we have written “GeeksforGeeks” in the text file.
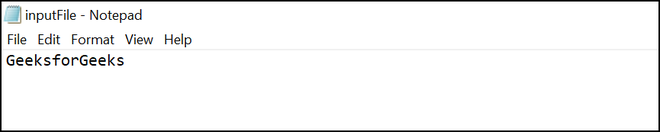
Text file data
4. We will now compile our program through the javac compiler using the command prompt:
javac GeeksforGeeks.java
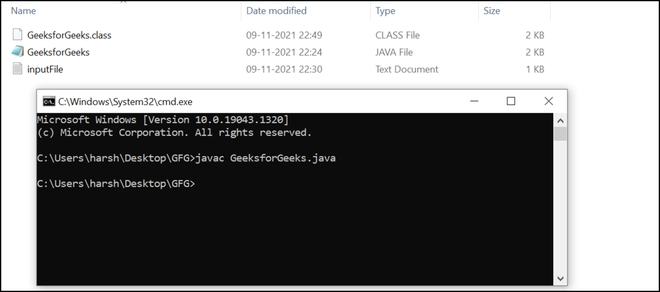
Compilation of the source code file
5. As you can see below GeeksforGeeks.class byte code file is generated. Now run the program using the following command:
java GeeksforGeeks
Output:
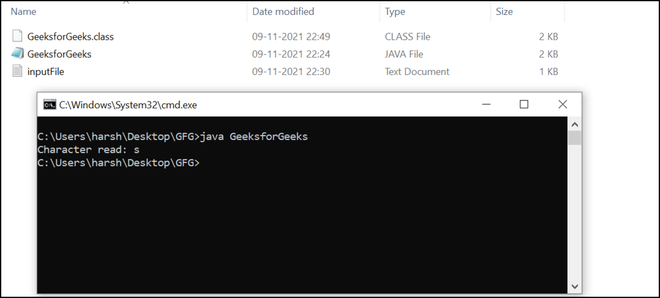
Output
Output Description: Since we have passed 4 as an argument to the skip() function in the program. As we can see in the program’s output, the first four characters (or bytes) are skipped (‘G‘, ‘e‘, ‘e‘, ‘k‘ ). The fifth character in GeekforGeeks is ‘s‘ hence, ‘s‘ is printed on the console.
Example 2:
Java
import java.io.FileInputStream;
import java.io.IOException;
public class GeeksforGeeks {
public static void main(String[] args)
throws IOException
{
FileInputStream inputFileStream = null ;
int integerValue = 0 ;
char characterValue;
try {
inputFileStream = new FileInputStream(
"C:\\Users\\harsh\\Desktop\\GFG\\inputFile.txt" );
inputFileStream.skip( 8 );
System.out.print( "String read: " );
while ((integerValue = inputFileStream.read())
!= - 1 ) {
characterValue = ( char )i;
System.out.print(characterValue);
}
}
catch (Exception exception) {
exception.printStackTrace();
}
finally {
if (inputFileStream != null )
inputFileStream.close();
}
}
}
|
In order to compile the above program in a local system, We can follow the same steps that we have mentioned in Example 1. After the compilation, we can run the program, and it generates the following output.
Output:
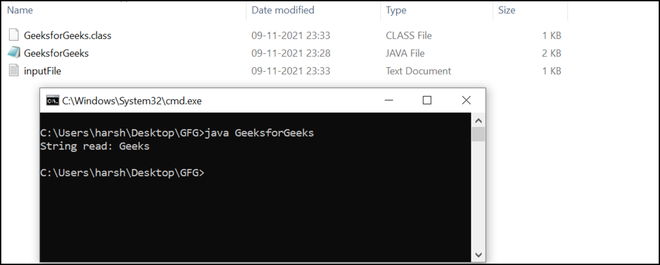
Output
Output Description: As you can see in the output first eight characters are skipped (‘G‘, ‘e‘, ‘e‘, ‘k‘, ‘s‘, ‘f‘, ‘o‘, ‘r‘). The remaining string is “Geeks” so it gets printed on the console.
Share your thoughts in the comments
Please Login to comment...